In this tutorial, I will guide you to create Java MVC Add, Edit, and Delete operations using JSP and Servlet with MySQL.
In my previous tutorial, I explained how to build the Login and Register script based on Java MVC codes that play the role of Model (Java Classes), View (JSP), and Controller(Servlet).
Now I used the same strategies regarding the previous tutorial in this tutorial and I have developed this project based on Java MVC.
I have clarified all about MVC in my previous tutorial. What is MVC Architecture in Java, POJO, or Model class purpose and DAO class as to how to apply the business logic in this class? If you don't know how it works on Java MVC, read my previous post. Let's begin building a new project.
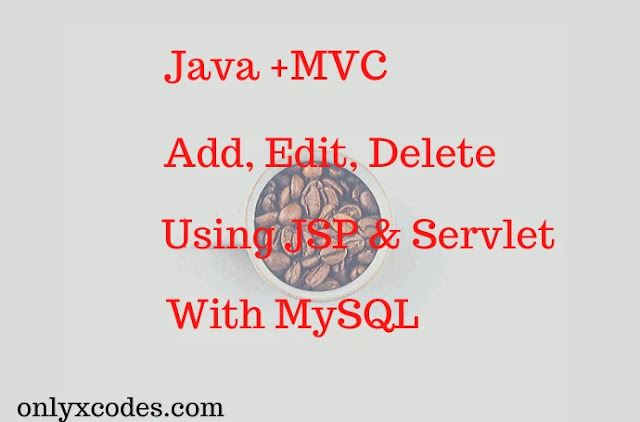
Prerequisite
Platfrom Version – Java 8
Editor: NetBeans IDE
Jar files – That project required three jar files. They're mysql-connector-java-5.1.15-bin.jar, jsp-api.jar, and servlet-api.jar.
Copy and paste all the jar files into WEB-INF —>lib folder.

I made 3 packages within the src folder.
com.mvc.bean: It contains the class POJO (Plain Old Java Object). This class protects the variable of entity member by the method getters and setters.
com.mvc.controller: contains the servlets.
com.mvc.dao: It contains class DAO (Data Access Object) logic for the operation of databases.
Database Schema
This SQL code will generate the "person" name table in your database. Go to your PhpMyAdmin to run the following SQL code.
Here, I used the previous database name called "db_mvcoperation" but you can use a different name according to your project requirement.
CREATE TABLE `person` (
`id` int(11) NOT NULL,
`name` varchar(15) NOT NULL,
`owner` varchar(15) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
add.jsp
On this page, I built an easy HTML form with two text box fields.
When you fill in form data and click on the Add button, the AddController first executes because it is the attribute value of the form tag action parameter (action="AddController").
The AddController URL I specified in the web.xml file.
<form method="post" action="AddController" onsubmit="return validate();">
<table>
<tr>
<td>Name</td>
<td><input type="text" name="txt_name" id="name"></td>
</tr>
<tr>
<td>Owner</td>
<td><input type="text" name="txt_owner" id="owner"></td>
</tr>
<tr>
<td><input type="submit" name="btn_add" value="Add"></td>
</tr>
</table>
<center>
<h3 style="color:red;">
<%
if(request.getAttribute("InsertErrorMsg")!=null)
{
out.println(request.getAttribute("InsertErrorMsg")); //get insert record fail error message from AddController.java
}
%>
</h3>
<h1><a href="index.jsp">Back</a></h1>
</center>
</form>
AddController.java
I created a Servlet file with AddController class name that is mapped to the URL: AddController.
This file gets all the values of the form that users enter.
This class communicates with the AddBean and AddDao model classes.
The class AddBean sets the property of form fields to that object and AddDao class contains logic for JDBC codes to insert new user records into the database table.
package com.mvc.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.mvc.bean.AddBean;
import com.mvc.dao.AddDao;
public class AddController extends HttpServlet
{
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException
{
if(request.getParameter("btn_add")!=null) //check button click event not null from add.jsp page after continue
{
String name=request.getParameter("txt_name"); //get textbox name "txt_name"
String owner=request.getParameter("txt_owner"); //get textbox name "txt_owner"
AddBean addBean=new AddBean(); //this class contain setting up all receive values from add.jsp page to seeter and getter method for application require effectively
addBean.setName(name); //set name through addBean object
addBean.setOwner(owner); //set owner through addBean object
AddDao addDao=new AddDao(); //this class contain main logic to perform function calling and database operation
String insertValidate=addDao.checkInsert(addBean); //send addBean object values into checkInsert() function in AddDao class
if(insertValidate.equals("INSERT SUCCESS")) //check calling checkInsert() function receive string "INSERT SUCCESS" after redirect to index.jsp page and display record
{
request.setAttribute("InsertSuccessMsg",insertValidate); //setAttribute value is "InsertSuccessMsg" for insert successfully message
RequestDispatcher rd=request.getRequestDispatcher("index.jsp");
rd.forward(request, response);
}
else
{
request.setAttribute("InsertErrorMsg",insertValidate); //setAttribute value is "InsertErrorMsg" for insert fail message
RequestDispatcher rd=request.getRequestDispatcher("add.jsp");
rd.include(request, response);
}
}
}
}
AddBean.java
In this class, I defined all fields of the form that must be private and implemented with the public by methods getters and setters.
package com.mvc.bean;
public class AddBean
{
private String name,owner;
public String getName(){
return name;
}
public void setName(String name){
this.name=name;
}
public String getOwner(){
return owner;
}
public void setOwner(String owner){
this.owner=owner;
}
}
AddDao.java
In this class, I created the MySQL database connection and applied the SQL insert query to properly insert new user records into the person table.
If records are added, this class will return the success message to AddController, otherwise, returns the failed message.
package com.mvc.dao;
import com.mvc.bean.AddBean;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
public class AddDao
{
public String checkInsert(AddBean addBean)
{
String name=addBean.getName(); //get name through addBean object and store in temporary variable "name"
String owner=addBean.getOwner(); //get owner through addBean object and store in temporary variable "owner"
String url="jdbc:mysql://localhost:3306/db_mvcoperation"; //database connection url string
String username="root"; //database connection username
String password=""; //database password
try
{
Class.forName("com.mysql.jdbc.Driver"); //load driver
Connection con=DriverManager.getConnection(url,username,password); //create connection
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("insert into person(name,owner) values(?,?)"); //sql insert query
pstmt.setString(1,name);
pstmt.setString(2,owner);
pstmt.executeUpdate(); //execute query
pstmt.close(); //close statement
con.close(); //close connection
return "INSERT SUCCESS"; //if valid return "INSERT SUCCESS" string
}
catch(Exception e)
{
e.printStackTrace();
}
return "FAIL INSERT"; //if invalid return "FAIL INSERT" string
}
}
edit.jsp
On this page, I made the editing form. When users click on the Edit hyperlink, the form collects selected records from the database and shows them in the text boxes.
If users edit the form and click on the Update button then the EditController runs because it is the edit form tag action parameter value (action="EditController").
The EditController is the Servlet class file URL that gets new values of edit form fields using the HTTP POST method.
Note: Look exactly at closing the update button I created the hidden text box which holds specific table IDs that can be retrieved. This hidden ID helps in updating existing records.
See just before the form tag is closed if any update error occurred this page will display a suitable message.
<form method="post" action="EditController" onsubmit="return validate();">
<table>
<%
if(request.getParameter("edit_id")!=null) //get edit_id from index.jsp page with href link and check not null after continue
{
int id=Integer.parseInt(request.getParameter("edit_id")); //get edit_id store in "id" variable
String url="jdbc:mysql://localhost:3306/db_mvcoperation"; //database connection url string
String username="root"; //database connection username
String password=""; //database password
try
{
Class.forName("com.mysql.jdbc.Driver"); //load driver
Connection con=DriverManager.getConnection(url,username,password); //create connection
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("select * from person where id=?"); //sql select query
pstmt.setInt(1,id);
ResultSet rs=pstmt.executeQuery(); //execute query and set in Resultset object rs.
while(rs.next())
{
%>
<tr>
<td>Name</td>
<td><input type="text" name="txt_name" id="name" value="<%=rs.getString("name")%>"></td>
</tr>
<tr>
<td>Owner</td>
<td><input type="text" name="txt_owner" id="owner" value="<%=rs.getString("owner")%>"></td>
</tr>
<tr>
<td><input type="submit" name="btn_edit" value="Update"></td>
</tr>
<input type="hidden" name="hidden_id" value="<%=rs.getInt("id")%>">
<%
}
pstmt.close(); //close statement
con.close(); //close connection
}
catch(Exception e)
{
e.printStackTrace();
}
}
%>
</table>
<center>
<h3 style="color:red;">
<%
if(request.getAttribute("UpdateErrorMsg")!=null)
{
out.print(request.getAttribute("UpdateErrorMsg")); //get update record fail error message from EditController.java
}
%>
</h3>
<h1><a href="index.jsp">Back</a></h1>
</center>
</form>
EditController.java
I created a Servlet class file called EditController that mapped to the URL: EditController. This class gets all the new values that are modified from users to edit form.
This class interacts with two EditBean and EditDao model classes.
The class EditBean sets the property of the editable fields for the edit form by that object and the EditDao class includes logic for JDBC codes to update specific user records.
package com.mvc.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.mvc.bean.EditBean;
import com.mvc.dao.EditDao;
public class EditController extends HttpServlet
{
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException
{
if(request.getParameter("btn_edit")!=null) //check button click event not null form edit.jsp page after continue
{
String name_up=request.getParameter("txt_name"); //get textbox name "txt_name"
String owner_up=request.getParameter("txt_owner"); //get textbox name "txt_owner"
int hidden_id=Integer.parseInt(request.getParameter("hidden_id")); //get hidden id name "hidden_id"
EditBean editBean=new EditBean(); //this class contain setting up all receive values from edit.jsp page to seeter and getter method for application require effectively
editBean.setName_UP(name_up);
editBean.setOwner_UP(owner_up); //set all value through editBean object
editBean.setHidden_ID(hidden_id);
EditDao editDao=new EditDao(); //this class contain main logic to perform function calling and database operation
String updateValidate=editDao.checkUpdate(editBean); //send editBean object values into checkUpdate() function in EditDao class
if(updateValidate.equals("UPDATE SUCCESS")) //check calling checkUpdate() function receive string "UPDATE SUCCESS" after redirect to index.jsp page and display update record
{
request.setAttribute("UpdateSuccessMsg",updateValidate); //setAttribute value is "UpdateSuccessMsg" for update successfully message
RequestDispatcher rd=request.getRequestDispatcher("index.jsp");
rd.forward(request, response);
}
else
{
request.setAttribute("UpdateErrorMsg",updateValidate); //setAttribute value is "UpdateErrorMsg" for update fail message
RequestDispatcher rd=request.getRequestDispatcher("edit.jsp");
rd.include(request, response);
}
}
}
}
EditBean.java
In this class, I defined properties of edit form and all properties I specified with the public by methods getters and setters to define private and implements.
package com.mvc.bean;
public class EditBean
{
private String name_up,owner_up;
private int hidden_id;
public String getName_UP(){
return name_up;
}
public void setName_UP(String name_up){
this.name_up=name_up;
}
public String getOwner_UP(){
return owner_up;
}
public void setOwner_UP(String owner_up){
this.owner_up=owner_up;
}
public int getHidden_ID(){
return hidden_id;
}
public void setHidden_ID(int hidden_id){
this.hidden_id=hidden_id;
}
}
EditDao.java
This is the DAO class which contains logic for JDBC codes for updating specific user records.
In this class, I set up all settings in the MySQL database connection and applied the SQL update query.
If users type new records this class will update existing records otherwise put old records the same as they are.
package com.mvc.dao;
import com.mvc.bean.EditBean;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
public class EditDao
{
public String checkUpdate(EditBean editBean)
{
String name_up=editBean.getName_UP();
String owner_up=editBean.getOwner_UP(); //get all value through editBean object and store in temporary variable
int hidden_id=editBean.getHidden_ID();
String url="jdbc:mysql://localhost:3306/db_mvcoperation"; //database connection url string
String username="root"; //database connection username
String password=""; //database password
try
{
Class.forName("com.mysql.jdbc.Driver"); //load driver
Connection con=DriverManager.getConnection(url,username,password); //create connection
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("update person set name=?,owner=? where id=? "); //sql update query
pstmt.setString(1,name_up);
pstmt.setString(2,owner_up);
pstmt.setInt(3,hidden_id);
pstmt.executeUpdate(); //execute query
pstmt.close(); //close statement
con.close(); //close connection
return "UPDATE SUCCESS"; //if valid return "UPDATE SUCCESS" string
}
catch(Exception e)
{
e.printStackTrace();
}
return "FAIL UPDATE"; //if invalid return "FAIL UPDATE" string
}
}
index.jsp
This is the home or index page.
Within this page, I have extracted all records from the person table and arranged them in table format.
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Owner</th>
<th>Edit</th>
<th>Delete</th>
</tr>
<%
String url="jdbc:mysql://localhost:3306/db_mvcoperation"; //database connection url string
String username="root"; //database connection username
String password=""; //database password
try
{
Class.forName("com.mysql.jdbc.Driver"); //load driver
Connection con=DriverManager.getConnection(url,username,password); //create connection
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("select * from person"); //sql select query
ResultSet rs=pstmt.executeQuery(); //execute query and set in ResultSet object rs.
while(rs.next())
{
%>
<tr>
<td><%=rs.getInt("id")%></td>
<td><%=rs.getString("name")%></td>
<td><%=rs.getString("owner")%></td>
<td><a href="edit.jsp?edit_id=<%=rs.getInt("id")%>">Edit</a></td>
<td><a href="delete.jsp?delete_id=<%=rs.getInt("id")%>">Delete</a></td>
</tr>
<%
}
pstmt.close(); //close statement
con.close(); //close connection
}
catch(Exception e)
{
e.printStackTrace();
}
%>
</table>
delete.jsp
I created a new page separately called "delete.jsp". If users click on the delete hyperlink this page will delete records according to the specific table ID.
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<%@page import="java.sql.PreparedStatement"%>
<%
if(request.getParameter("delete_id")!=null) //get delete_id from index.jsp page with href link and check not null after continue
{
int id=Integer.parseInt(request.getParameter("delete_id")); //get delete_id store in "id" variable
String url="jdbc:mysql://localhost:3306/db_mvcoperation"; //database connection url string
String username="root"; //database connection username
String password=""; //database password
try
{
Class.forName("com.mysql.jdbc.Driver"); //load driver
Connection con=DriverManager.getConnection(url,username,password); //create connection
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("delete from person where id=? "); //sql delete query
pstmt.setInt(1,id);
pstmt.executeUpdate(); //execute query
RequestDispatcher rd=request.getRequestDispatcher("index.jsp"); //after delete success forward index.jsp page
rd.forward(request, response);
pstmt.close(); //close statement
con.close(); //close connection
}
catch(Exception e)
{
e.printStackTrace();
}
}
%>
web.xml
See I Configured the web.xml file below. The file tags help map the available AddController and EditController Servlet files in different packages.
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="3.1" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd">
<servlet>
<servlet-name>AddController</servlet-name>
<servlet-class>com.mvc.controller.AddController</servlet-class>
</servlet>
<servlet>
<servlet-name>EditController</servlet-name>
<servlet-class>com.mvc.controller.EditController</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>AddController</servlet-name>
<url-pattern>/AddController</url-pattern>
</servlet-mapping>
<servlet-mapping>
<servlet-name>EditController</servlet-name>
<url-pattern>/EditController</url-pattern>
</servlet-mapping>
<session-config>
<session-timeout>
30
</session-timeout>
</session-config>
</web-app>
Read Also:
Java MVC Login and Register Script Using JSP & Servlet With MySQL
How to Send Email After Registration with Activation Link in Java
Download Codes
Super very helpfull
ReplyDeleteThanks. Cheers
ReplyDelete