Hi, In this tutorial I will explain how to check username availability using Ajax in JSP.
Checking username availability is a common feature in web applications to ensure that a new user can select a unique username. Implementing this feature using Ajax in JSP provides a seamless user experience by checking the availability in real time without requiring a page reload.
The process of this tutorial is straightforward: after the user enters their desired username in the input box, the "$.ajax()" function dynamically launches an HTTP POST request, retrieves the username via the JSP Script from the MySQL Database Table and indicates whether the username is available.
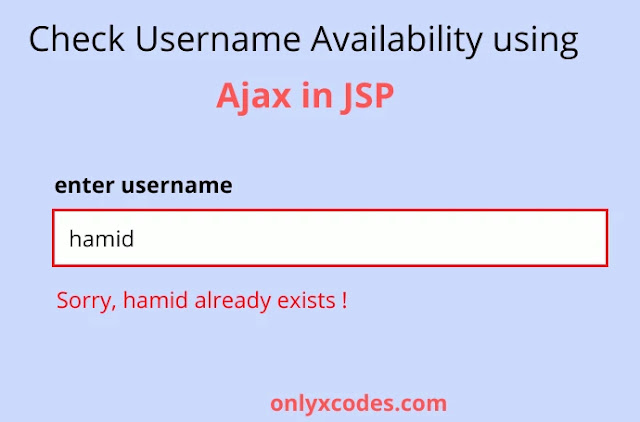
Create Table
I have used "username_available_db" named database and within this database, I have created the "user" named table.
CREATE TABLE `tbl_user` (
`id` int(11) NOT NULL,
`username` varchar(20) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1 ROW_FORMAT=COMPACT;

I have added a dummy record to the table to see if the username is available.
INSERT INTO `tbl_user` (`id`, `username`) VALUES
(2, 'Hamid');
3. Make Text Box
Using the Bootstrap package, I made an easy textbox. This text box will indicate whether or not the username exists once users have typed their username into it.
<form role="form" method="post">
<div class="form-group">
<label>Enter Username</label>
<input type="text" id="username" class="form-control" placeholder="enter username">
</div>
<span id="available"> <!--- data show this span tag --->
</span>
</form>
Implement Ajax Code
I've included some jQuery Ajax code here. If the user types in their username in the text box and clicks outside of it, an HTTP POST event will be triggered by the blur() method. The Ajax() function will then send the textbox value request to the "check.jsp" file, which will display whether the username is available or not.
My Note: The #username is the textbox ID attribute and the .val() method gets the entered value using this attribute.Â
<script type="text/javascript">
$(document).ready(function(){
$("#username").blur(function(){
var username =$("#username").val();
if(username.length >= 3)
{
$.ajax({
url:"check.jsp",
type:"post",
data:"uname="+username,
dataType:"text",
success:function(data)
{
$("#available").html(data)
}
});
}
else
{
$("#available").html(" ");
}
});
});
</script>
check.jsp
I use the JSP code in this file to verify the textbox name value request.
This is a tiny JSP file that works smoothly on the backend and contains JSP code. Using the $.ajax() function, it is called.
The username is scanned from the database by this file. If the username is found, Ajax receives the username; if not, it receives the message Sorry, username already exists.
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<%
String dburl="jdbc:mysql://localhost:3306/username_available_db";
String dbusername="root";
String dbpassword ="";
if(request.getParameter("uname")!=null)
{
String user_name=request.getParameter("uname");
try
{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(dburl,dbusername,dbpassword);
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("SELECT * FROM tbl_user WHERE username=? ");
pstmt.setString(1,user_name);
ResultSet rs=pstmt.executeQuery();
if(rs.next())
{
%>
<span class="text-danger">Sorry, <%=rs.getString("username")%> Already Exists ! </span>
<%
}
else
{
%>
<span class="text-success">Username is available</span>
<%
}
con.close(); //close connection
}
catch(Exception e)
{
e.printStackTrace();
}
}
%>
Download Codes
No comments:
Post a Comment