Hi Friends, following the tutorial post you will learn Delete MySQL Row Using JSP & Ajax with SweetAlert2.
I have already discussed delete MySQL rows with bootstrap confirm modal in my recent post. Now I am going to use this dialog to delete MySQL row with Ajax in JSP for the new SweetAlert2 confirmation dialog.
At the moment of the data deletion phase, the SweetAlert2 confirms dialog generates a sweet animation impact on the popup window; people mostly enjoy animated popup UI dialog compared to a plain default JavaScript confirmation dialog.
If you like animated popup dialog please exchange the default JavaScript confirm the dialog with SweetAlert2 confirmation dialog for all visiting fresher folks to get better UI / UX experiences. Let's dive into the tutorial we generate this dialog.
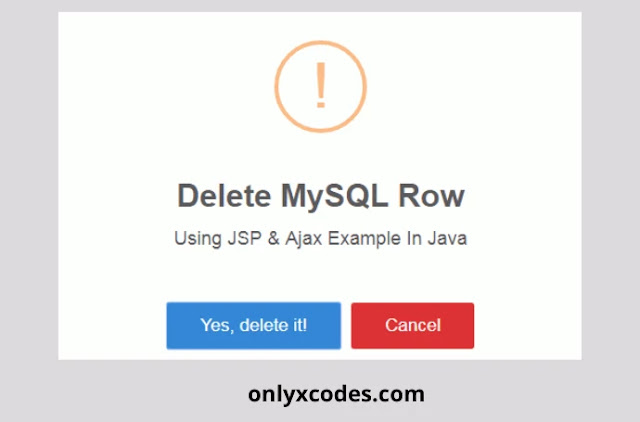
Table Content
1. Database / Table Creating
2. Retrieve Record From Table
3. Display Record
4. SweetAlert2 Confirmation Delete Dialog
5. $.ajax() Method Deleting Code
6. delete.jsp
7. SwalDelete() Function To Handle SweetAlert Delete by Ajax
8. Activate The SwalDelete() function
9. index.jsp / All code here.
1. Database / Table Creating
I've already set up the 'sweetalert_db' name database, so first create your PhpMyAdmin database and then copy and paste SQL codes below to create a fruit table.
CREATE TABLE `tbl_fruit` (
`id` int(11) NOT NULL,
`fruit_name` varchar(30) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1 ROW_FORMAT=COMPACT;

I inserted 5 different dumping fruit name records in the table so you can copy the paste below codes in your table option again.
INSERT INTO `tbl_fruit` (`id`, `fruit_name`) VALUES
(1, 'Apple'),
(2, 'Banana'),
(3, 'Mango'),
(4, 'Orange'),
(5, 'Grapes');
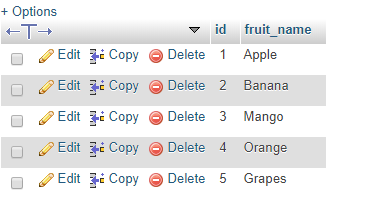
2. Retrieve Record From Tabe
That’s read.jsp file inside this file to view the fruit records from tbl_fruit.
The last delete column, including two data properties, is for the record deletion phase with the anchor tag.Â
<td><a id="delete_fruit" data-id="<%=rs.getInt("id")%>" href="javascript:void(0)" class="btn btn-sm btn-danger"><i class="glyphicon glyphicon-trash"></i></a></td>
The data-id attribute will store the fruit Id and cause the delete_fruit Id by using the jQuery click action, so you can get the fruit Id from the table.Â
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<div class="table-responsive">
<table class="table table-striped table-bordered table-hover">
<thead>
<tr>
<th>Fruits Name</th>
<th>Delete</th>
</tr>
</thead>
<tbody>
<%
String dburl="jdbc:mysql://localhost:3306/sweetalert_db";
String dbusername="root";
String dbpassword ="";
try
{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(dburl,dbusername,dbpassword);
PreparedStatement pstmt=null;
pstmt=con.prepareStatement("SELECT * FROM tbl_fruit");
ResultSet rs=pstmt.executeQuery();
while(rs.next())
{
%>
<tr>
<td><%=rs.getString("fruit_name")%></td>
<td><a id="delete_fruit" data-id="<%=rs.getInt("id")%>" href="javascript:void(0)" class="btn btn-sm btn-danger"><i class="glyphicon glyphicon-trash"></i></a></td>
</tr>
<%
}
con.close();
}
catch(Exception e)
{
e.printStackTrace();
}
%>
</tbody>
</table>
</div>
3. Display Record
We fully build 'read.jsp' a file that carries MySQL data in tabular format, Next use the function jQuery load() to show the file read.jsp inside the index file.Â
<!--- read.jsp files table will be load in this div --->
<div id="load_fruit"></div>
<!--- javascript function to load read.jsp file --->
function readFruit(){
$('#load_fruit').load('read.jsp');
}
4. SweetAlert2 Confirmation Delete Dialog
Below you can see the SweetAlert2 confirm dialog, which deletes all data from either the popup dialog.
The showCancelButton, confirmButtonColor, cancelButtonColor, and confirmButtonText all the properties created by this dialog are being asked to generate the popup window.Â
Within the Promise function, we use the $.ajax() method to send a request for deletion and that is responsible for the process of deletion of records.
swal({
title: 'Are you sure?',
text: "It will be deleted permanently",
type: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6', //sweetalert confirm dialouge
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!',
showLoaderOnConfirm: true,
preConfirm: function() {
return new Promise(function(resolve){ //Promise() function take care delete process done by ajax
//ajax codes beginning here
},
allowOutsideClick: false
});
5. $.ajax() Method Deleting Code
See below ajax codes that must be within the function of SweetAlert Promise and it will keep hold of the deletion operation.
$.ajax({
url:'delete.jsp',
type:'POST',
data:'delete='+fruitId,
dataType:'JSON'
})
.done(function(response){
swal('Deleted!', response.message, response.status);
readFruit();
})
.fail(function(){
swal('Oops...', 'Something went wrong with ajax !', 'error');
});
});
6. delete.jsp [ JSP Code To Delete Record ]
Within this file, we create data deleting codes wrapping it up. Â
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<%@page import="org.json.JSONObject,java.util.*"%>
<%
if(request.getParameter("delete")!=null)
{
int getID=Integer.parseInt(request.getParameter("delete"));
String dburl="jdbc:mysql://localhost:3306/sweetalert_db";
String dbusername="root";
String dbpassword="";
try
{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(dburl,dbusername,dbpassword);
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("DELETE FROM tbl_fruit WHERE id=?");
pstmt.setInt(1,getID);
int i=pstmt.executeUpdate();
JSONObject obj=new JSONObject();
if(i!=0){
obj.put("status", "success");
obj.put("message", "Fruit Delete Successfully");
}
else{
obj.put("status", "error");
obj.put("message", "Unable to delete fruit....");
}
out.print(obj);
con.close(); //close the connection
}
catch(Exception e)
{
e.printStackTrace();
}
}
%>
7. SwalDelete() Function To Handle SweetAlert Delete by Ajax
See below the completed custom SwalDelete() function that manages the delete operation with SweetAlert confirm dialog from ajax request.
function SwalDelete(fruitId){
swal({
title: 'Are you sure?',
text: "It will be deleted permanently",
type: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6', //sweetalert confirm dialouge
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!',
showLoaderOnConfirm: true,
preConfirm: function() {
return new Promise(function(resolve){ //Promise() function take care delete process done by ajax
$.ajax({
url:'delete.jsp', //ajax codes start for delete data
type:'POST',
data:'delete='+fruitId,
dataType:'JSON'
})
.done(function(response){
swal('Deleted!', response.message, response.status); readFruit();
})
.fail(function(){
swal('Oops...', 'Something went wrong with ajax !', 'error');
});
});
},
allowOutsideClick: false
});
}
8. Activate The SwalDelete() function
Everything is done, all we need is to activate the SwalDelete() function by using the below code, it's here. Whenever we press the delete button, the SwalDelete function receives the Id of that unique row and the bulk of the procedure will occur.
$(document).ready(function(){
readFruit(); /* it will load fruit name when document loads */
$(document).on('click','#delete_fruit',function(e){
var fruitId = $(this).data('id');
SwalDelete(fruitId);
e.preventDefault();
});
});
9. index.jsp / All code here
Below you can see all index.jsp file codes which I have explained separately above. But here I've outlined the entire location of codes you'll find some codes easily.
Row no 7 to 8 – I have imported CSS library of Bootstrap and SweetAlert dialog.
Row no 35 – Here's the tag < div id="load fruit."></div>. In which the jQuery load() function loads MySQL record.
Row no 40 to 42 – JQuery library and JavaScript library of bootstrap and SweetAlert dialog.
Row no 43 to 93 – Total codes to create an animated popup window for Sweet Alert.
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>SweetAlert2 : Delete MySQL Row Using JSP,AJAX Example</title>
<link href="assets/bootstrap/css/bootstrap.min.css" rel="stylesheet" type="text/css">
<link href="assets/swal2/sweetalert2.min.css" rel="stylesheet" type="text/css">
</head>
<body>
<nav class="navbar navbar-default navbar-static-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#navbar" aria-expanded="false" aria-controls="navbar">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="http://www.onlyxcodes.com/">onlyxcodes</a>
</div>
<div id="navbar" class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li class="active"><a href="http://www.onlyxcodes.com/2019/03/sweetalert2-delete-mysql-row-using-jsp.html" target="_blank">Back to Tutorial</a></li>
</ul>
</div><!--/.nav-collapse -->
</div>
</nav>
<div class="container">
<h3 align="center">SweetAlert2 : Delete MySQL Row Using JSP, Ajax Example</h3>
<div class="row">
<div id="load_fruit"></div>
</div>
</div>
<script src="js/jquery-1.12.4-jquery.min.js" type="text/javascript"></script>
<script src="assets/bootstrap/js/bootstrap.min.js" type="text/javascript"></script>
<script src="assets/swal2/sweetalert2.min.js" type="text/javascript"></script>
<script type="text/javascript">
$(document).ready(function(){
readFruit(); /* it will load fruit name when document loads */
$(document).on('click','#delete_fruit',function(e){
var fruitId = $(this).data('id');
SwalDelete(fruitId);
e.preventDefault();
});
});
function SwalDelete(fruitId){
swal({
title: 'Are you sure?',
text: "It will be deleted permanently",
type: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!',
showLoaderOnConfirm: true,
preConfirm: function() {
return new Promise(function(resolve){
$.ajax({
url:'delete.jsp',
type:'POST',
data:'delete='+fruitId,
dataType:'JSON'
})
.done(function(response){
swal('Deleted!', response.message, response.status); readFruit();
})
.fail(function(){
swal('Oops...', 'Something went wrong with ajax !', 'error');
});
});
},
allowOutsideClick: false
});
}
function readFruit(){
$('#load_fruit').load('read.jsp');
}
</script>
</body>
</html>
Download Codes
No comments:
Post a Comment