I will show you jQuery onchange select option value using Ajax in JSP.
There are other cases you'll need a dynamic dropdown when you have a dropdown that includes the name of the country and you'll need to discover all selected state and city names in the selected box or dropdown.
We say loads content from the server dynamically and presenting it in a select box without unloading the entire page with both the use of jQuery and Ajax.
For example, we will collect every country from the country table and then use Ajax and jQuery to collect all state selected. When we've all the state we are going to add on state dropdown to all-state. The same method applies to state and city procedures. Let's get this task done very easily.
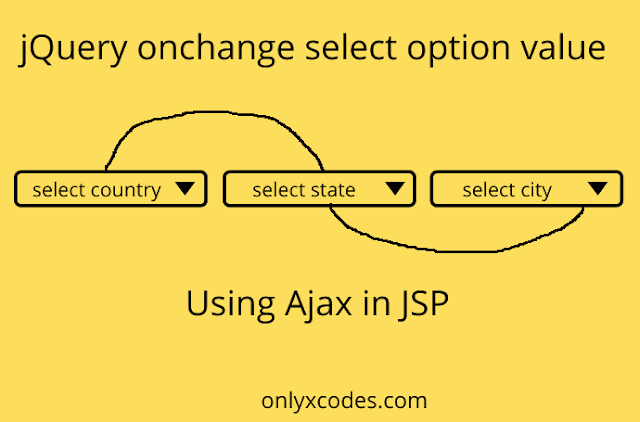
Database And Table Configuration
This tutorial uses the database called "onchange_select_option_db" and this database involves three-country, state, and city tables being presented here.Â
The table of states has a relation with the table of countries and the table of cities has a relation with the table of states.
country :
The following SQL builds a country table with two country id fields and country name fields.
CREATE TABLE `country` (
`country_id` int(11) NOT NULL,
`country_name` varchar(14) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;

state :
Below are SQL codes that build a country id foreign key-based state table.
CREATE TABLE `state` (
`state_id` int(11) NOT NULL,
`country_id` int(11) NOT NULL,
`state_name` varchar(15) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;

city :
Last to build a city table based on the foreign state id key below SQL codes.
CREATE TABLE `city` (
`city_id` int(11) NOT NULL,
`state_id` int(11) NOT NULL,
`city_name` varchar(15) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;

country table dumping data :
Execute codes below for storing two-country dumping data.
INSERT INTO `country` (`country_id`, `country_name`) VALUES
(1, 'India'),
(3, 'United States');
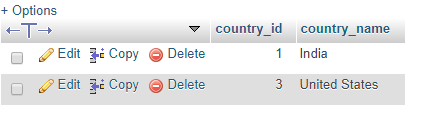
state table dumping data :
By following SQL codes to store the state name depends on the country id foreign key.
INSERT INTO `state` (`state_id`, `country_id`, `state_name`) VALUES
(1, 1, 'Gujarat'),
(2, 1, 'Maharastra'),
(3, 3, 'Alaska'),
(4, 3, 'Alabama');
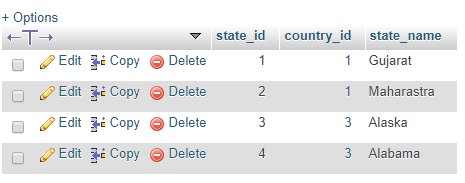
city table dumping data :
Below are SQL codes to store the name of the city depending on the foreign key state id.Â
INSERT INTO `city` (`city_id`, `state_id`, `city_name`) VALUES
(1, 1, 'Vadodra'),
(2, 1, 'Surat'),
(3, 2, 'Pune'),
(4, 2, 'Mumbai'),
(5, 3, 'Angoon'),
(6, 3, 'Aniak'),
(7, 4, 'Abbeville'),
(8, 4, 'Alpine');
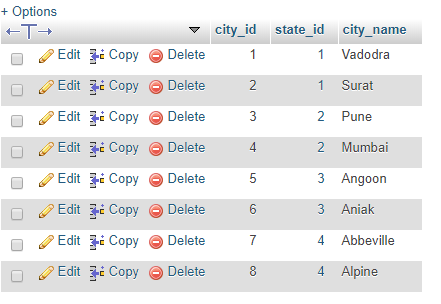
index.jsp
I put the JavaScript and JSP codes all over. That shows three selected boxes, country, state, and city.
The country box already gets all countries from the database. Depending on the on-change function event, the state and city will change automatically.
country_change() function code guide :-
When we select any country name then the function country_change() event will cause, Under ajax{( )} function sending selected country id request to 'state.jsp' page using an HTTP POST method to get all-state names in ajax response from the state table.
Finally, success function to display state name by country id selection inside the state dropdown.
#country is id attribute of the country select box using that attribute the .val() method to get the country id value.
state_change() function code guide :-
The same procedure here but we replace the name of the function if we select any state name that causes the state_change() function, Inside ajax {( )} function, sends selected state id to request to 'city.jsp' page using the HTTP POST method to get all city names in ajax response from city table.Â
The same function here is the success of showing the name of the city within the city drop-down according to the selection of the state id.Â
#state is the state select box id attribute that uses the .val() method to get the value of the state id.
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>How to Make jQuery Onchange Select Option Value using ajax in JSP</title>
<link href="bootstrap/css/bootstrap.min.css" rel="stylesheet" type="text/css">
<script src="js/jquery-1.12.4-jquery.min.js" type="text/javascript"></script>
<script src="bootstrap/js/bootstrap.min.js" type="text/javascript"></script>
<script type="text/javascript">
function country_change()
{
var country = $("#country").val();
$.ajax({
type: "POST",
url: "state.jsp",
data: "country_id="+country,
cache: false,
success: function(response)
{
$("#state").html(response);
}
});
}
function state_change()
{
var state = $("#state").val();
$.ajax({
type: "POST",
url: "city.jsp",
data: "state_id="+state,
cache: false,
success: function(response)
{
$("#city").html(response);
}
});
}
</script>
</head>
<body>
<div class="container">
<h3 align="center"><a href="https://www.onlyxcodes.com/2019/05/jquery-onchange-select-option-value-using-ajax.html">jQuery Onchange Select Option Value Using Ajax in JSP</a></h3>
</br>
<div class="form-group">
<label class="col-sm-1 control-label">Country</label>
<div class="col-sm-3">
<select class="form-control" id="country" onchange="country_change()">
<option value="" selected="selected"> - select country - </option>
<%
String dburl="jdbc:mysql://localhost:3306/onchange_select_option_db";
String dbusername="root";
String dbpassword="";
try
{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(dburl,dbusername,dbpassword);
PreparedStatement pstmt=null ;
pstmt=con.prepareStatement("SELECT * FROM country");
ResultSet rs=pstmt.executeQuery();
while(rs.next())
{
%>
<option value="<%=rs.getInt("country_id")%>">
<%=rs.getString("country_name")%>
</option>
<%
}
}
catch(Exception e)
{
e.printStackTrace();
}
%>
</select>
</div>
<label class="col-sm-1 control-label">State</label>
<div class="col-sm-3">
<select class="form-control" id="state" onchange="state_change()">
<option selected="selected"> - select state - </option>
</select>
</div>
<label class="col-sm-1 control-label">City</label>
<div class="col-sm-3">
<select class="form-control" id="city">
<option selected="selected"> - select city - </option>
</select>
</div>
</div>
</body>
</html>
state.jsp
Here are JSP codes that select state records from the state table base of country_id column and dynamically return all records to ajax success function.
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<%
if(request.getParameter("country_id")!=null)
{
int country_id=Integer.parseInt(request.getParameter("country_id"));
String dburl="jdbc:mysql://localhost:3306/onchange_select_option_db";
String dbusername="root";
String dbpassword="";
try
{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(dburl,dbusername,dbpassword);
PreparedStatement pstmt=null ;
pstmt=con.prepareStatement("SELECT * FROM state WHERE country_id=? ");
pstmt.setInt(1,country_id);
ResultSet rs=pstmt.executeQuery();
%>
<option selected="selected">--Select State--</option>
<%
while(rs.next())
{
%>
<option value="<%=rs.getInt("state_id")%>">
<%=rs.getString("state_name")%>
</option>
<%
}
con.close(); //close connection
}
catch(Exception e)
{
out.println(e);
}
}
%>
city.jsp
Above fairly JSP codes here which select city records from city table base of state_id column and dynamically return all records to ajax success function.
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<%
if(request.getParameter("state_id")!=null)
{
int state_id=Integer.parseInt(request.getParameter("state_id"));
String dburl="jdbc:mysql://localhost:3306/onchange_select_option_db";
String dbusername="root";
String dbpassword="";
try
{
Class.forName("com.mysql.jdbc.Driver"); //load driver
Connection con=DriverManager.getConnection(dburl,dbusername,dbpassword);
PreparedStatement pstmt=null ;
pstmt=con.prepareStatement("SELECT * FROM city WHERE state_id=? ");
pstmt.setInt(1,state_id);
ResultSet rs=pstmt.executeQuery();
%>
<option selected="selected">--Select city--</option>
<%
while(rs.next())
{
%>
<option value="<%=rs.getInt("city_id")%>">
<%=rs.getString("city_name")%>
</option>
<%
}
con.close(); //close connection
}
catch(Exception e)
{
out.println(e);
}
}
%>
Download Codes
Sir Put new tutorials fast
ReplyDelete