In this tutorial you will learn how to call servlet from Ajax and jQuery.
Ajax (Asynchronous JavaScript and XML) is a method for making asynchronous HTTP requests from a web page. This allows you to update a web page without reloading the entire page. jQuery is a popular JavaScript library that makes it easy to use Ajax.
Servlets are Java programs that can be used to handle HTTP requests. They are often used to generate dynamic web content.
To call a servlet from Ajax jQuery, you will need to use the jQuery ajax() method. The ajax() method takes several parameters, including the URL of the servlet, the HTTP method (GET or POST), the data to send to the servlet, and the callback function to execute when the servlet responds.
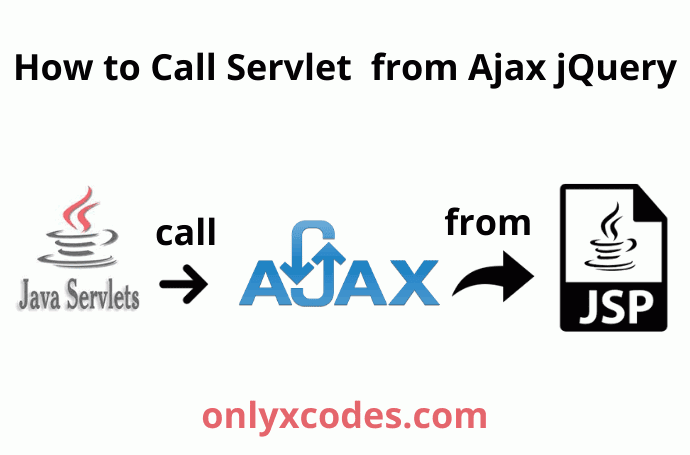
I'm assuming you already have Eclipse IDE installed on your system.
Create A New Project
Step 1 – Launch the Eclipse IDE. As seen in the illustration, go to File -> New -> Dynamic Web Project.
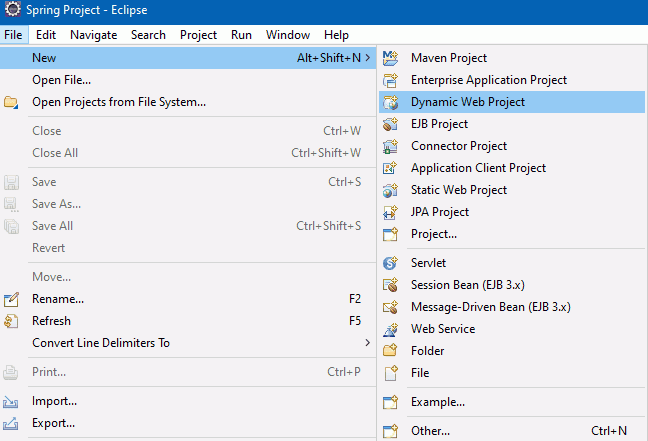
Step 2 – In the second stage, I only typed the project name below, and I placed the other default configuration unchanged. After you've filled in your name, click the Next button.Â
Project Name – call servlet from ajax
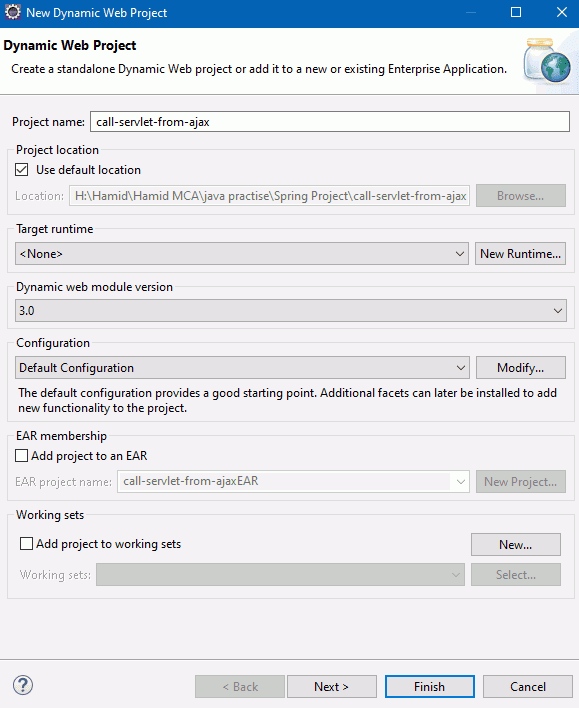
Step 3 – A new window has opened. I used the default configuration, and now we'll build a Servlet class in the src folder.
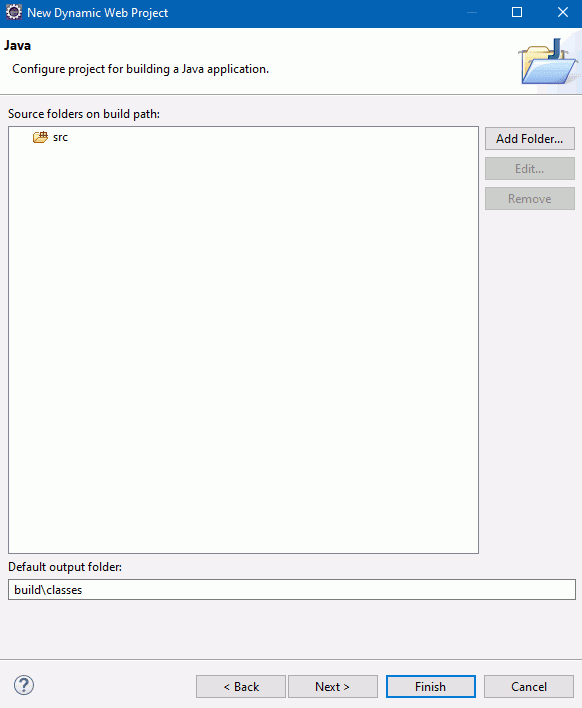
Step 4 – Finally, the final step in establishing a project. Choose the Finish button. Since it isn't needed, I unchecked the checkbox for generating web.xml file deployment descriptor.
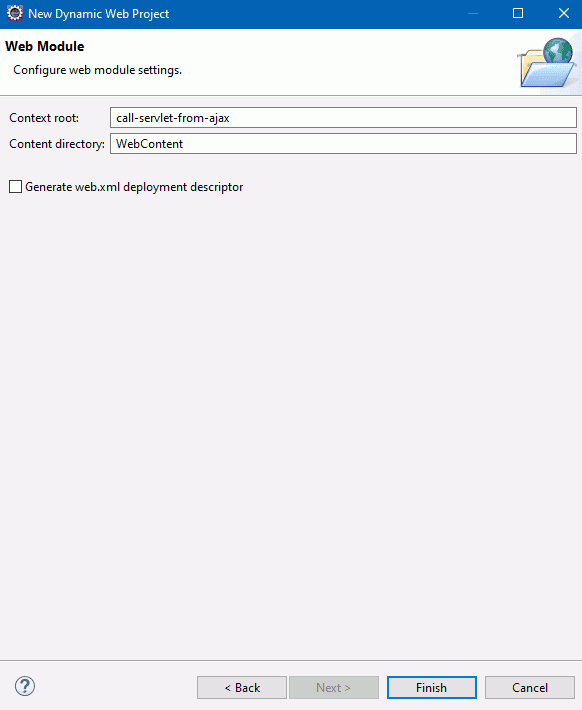
See the complete project directory structure, as well as the additional packages we'll create in this project, ahead.
Note: I've already saved the jQuery file in the JS folder and imported the bootstrap package folder into the WebContent folder for a better user interface.
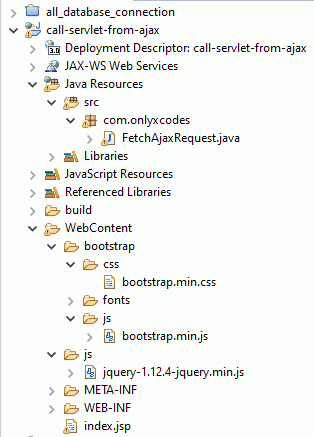
index.jsp
Inside the WebContent folder, I made a JSP page with the name index.jsp and I Built a simple form with an input text box and a submit button using the bootstrap classes on this page.
This JSP page's input text box request is sent to the Servlet using an ajax method.
<form id="formID" class="form-horizontal">
<div class="form-group first_division">
<label class="col-sm-3 control-label">Enter Name</label>
<div class="col-sm-6">
<input type="text" id="txt_name" class="form-control" placeholder="enter name" />
</div>
</div>
<div class="form-group first_division">
<div class="col-sm-offset-3 col-sm-6 m-t-15">
<input type="button" id="btn_submit" class="btn btn-success btn-block" value="Submit">
</div>
</div>
<b><h2 class="text-success text-center" id="show_result"></h2></b>
</form>
See how to send a POST request from ajax to a servlet and get a response from the servlet on the same JSP page without reloading.
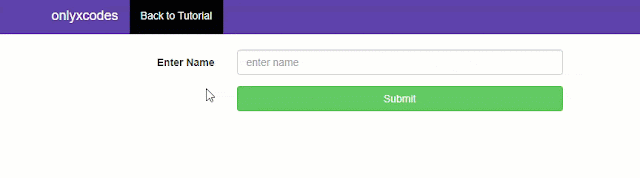
Call the Servlet from ajax() by Implementing jQuery Ajax Code
In this code, when a user clicks on the submit button, the click() method is triggered with the ID "btn_submit" and calls the ajax() function.
The URL parameter "FetchAjaxRequest" specifies the URL of the servlet class file.
In the type parameter, I specified the HTTP POST method.
In the data parameter, I specified 'yourname' as the data to send to the servlet class file.
I used the success callback function. If our ajax() function request will properly execute, the success callback function will display the response of our servlet class file.
Last, I used the JavaScript reset() method to erase all the values of the form elements with the id #formID of the form tag.
<script src="js/jquery-1.12.4-jquery.min.js" type="text/javascript"></script>
<script type="text/javascript">
$(document).ready(function(){
$('#btn_submit').click(function(){
var name=$('#txt_name').val();
if(name=="")
{
alert("Please enter your name ! ")
}
else
{
$.ajax({
url:'FetchAjaxRequest',
type:'POST',
data:'yourname='+name,
success:function(response)
{
$('#show_result').html(response);
$('#formID')[0].reset();
}
});
}
});
});
</script>
To process the AJAX request, create a Servlet class.
FetchAjaxRequest.java
The last move is to build a servlet class that can receive AJAX requests from the browser. Add the following code to a class named FetchAjaxRequest.java.
package com.onlyxcodes;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/FetchAjaxRequest")
public class FetchAjaxRequest extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException
{
String name = request.getParameter("yourname");
response.getWriter().print("Hi, "+ name);
}
}
Explanation:
In front of the class, the @WebServlet annotation specifies the servlet URL. When the user submits the form, the servlet container calls the servlet's doPost method. Within the doPost method, we will complete the following tasks.
The getParameter() method is used to get user-defined text box values which are transferred through the ajax data property.
A request is an object of HttpServletRequest and the response is an object of HttpServletResponse. These objects are used to get requests from browsers and to send responses to users.
Using the response object, we are sending a response to the browser with the welcoming text "Hi" attached.
The getWriter() method delivers word text to the user, and we publish the output on the browser JSP page as a response using the print() method.
Download Codes
No comments:
Post a Comment