Hi Friends, in this tutorial, we'll develop a Rest API by combining Spring Data Rest and Spring Boot.
Spring Data REST merges Spring Data capabilities with Spring HATEOAS (Hypermedia As The Engine Of Application State) to enable developing hypermedia-driven REST APIs on top of Spring Data repositories simple. Simple REST endpoints for executing CRUD operations on Spring Data repositories are created and exposes using the basic functionality supplied out of the box.
Each resource should have its own URI / endpoint, according to the HATEOAS concept. We can implement RESTful API around Spring Data Repositories without creating a lot of code like controller and service classes. It is the fundamental set of rules that all RESTful Web Services must adhere to.
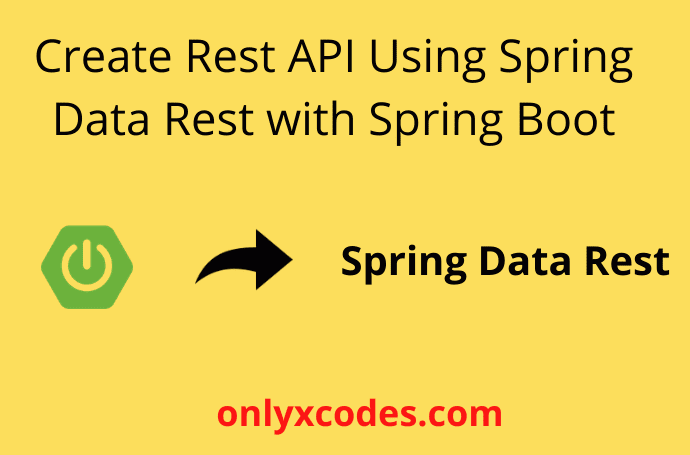
What is Spring Data Rest?
Spring Data REST builds on top of Spring Data repositories, analyzes your application’s domain model, and exposes hypermedia-driven HTTP resources for aggregates contained in the model. ( source: Spring Doc )
Prerequisites
You'll require Java 8 or above (up to Java 11) on your system to keep in with this tutorial.Â
You'll need an IDE (Integrated Development Environment) to assist you in the development process in addition to Java. Spring Tool Suite (STS), IntelliJ IDEA, and Eclipse are some of the best options out there for this. STS was used to make this project.
Create Database
Create a database in PhpMyAdmin with whatever name you like. I used springboot_data_rest_api_db.
Bootstrapping Your Project with Spring Boot
To begin, you'll need to bootstrap your new project before digging into creating your RESTful web services API. To do so, access the Spring Initializr website in your browser and complete the following form:
When you first visit the site, the three options listed below are selected by default. As it is, I'm the only one that mentioned it.
- Project: Maven Project
- Language: Java
- Spring Boot: 2.5.1Â
Project MetadataÂ
- Group: com.onlyxcodes
- Artifact:SpringDataRestAPI
- Description: Create Rest API using Spring Data Rest
- Package name: com.onlyxcodes.app
- Packaging: Jar
- Java: 8
Next, under the Dependencies part, add five dependencies using the search for dependencies field:Â
- Spring Web: This dependency for using Tomcat and Spring MVC to create web apps.Â
- MySQL Driver: This dependency allows you to interact with the MySQL database.Â
- Spring Data JPA: Its Java Persistence API This dependency facilitates the mapping of SQL databases to objects and conversely.Â
- Rest Repositories: That This dependency will allow you to make a RESTful API out of your database.Â
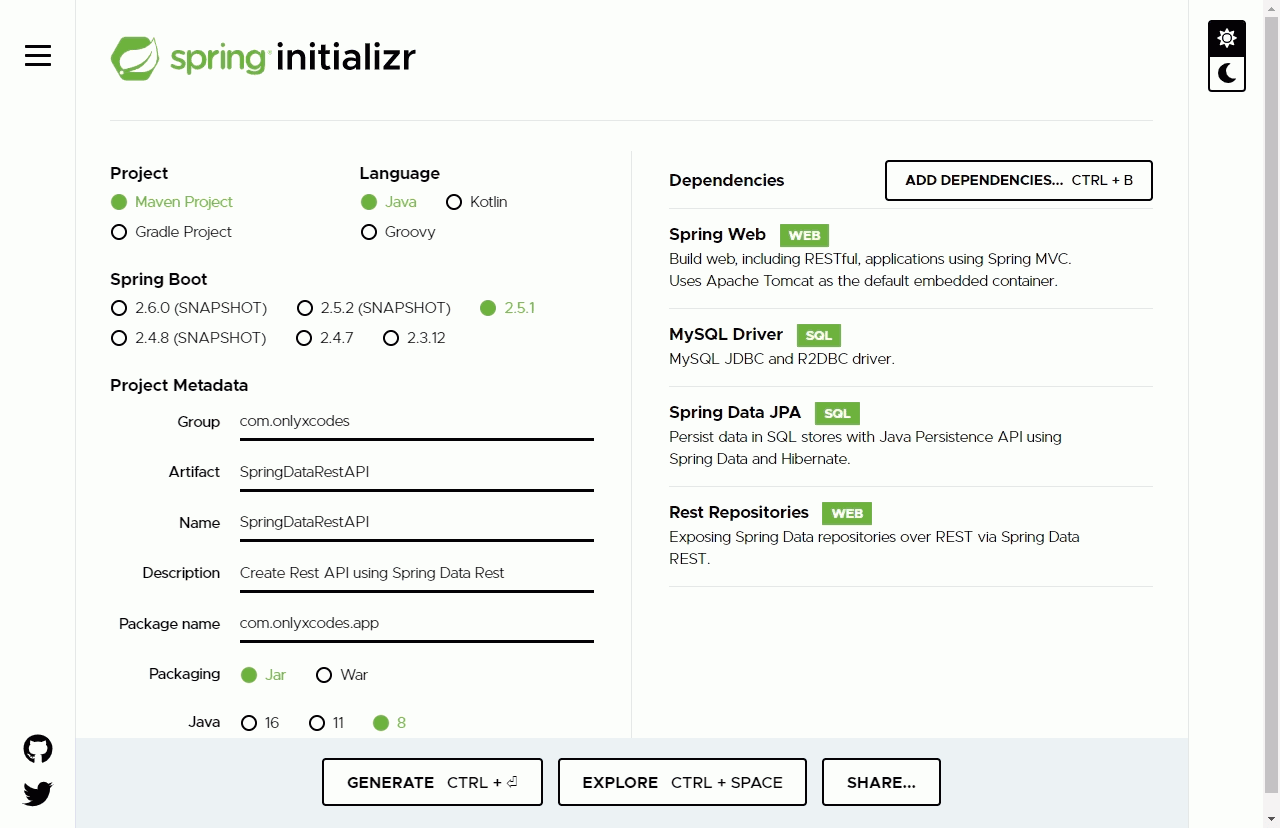
To download your new app, fill out this form and then click the Generate Project button. After that, you must extract the downloaded zip file and then transfer the extracted folder to the desired place.Â
Open your new project in your IDE. If you're using STS, for example, open the project by following the steps below.
Step 1 – Select File -> Open Projects from File System.Â
Step 2 – After you've opened a new window, you'll notice two buttons on the right side; click the Directory button.Â
Step 3 – Select your project from the place where you extracted it.Â
Step 4 – Look for two checkboxes with the name of your project. Check the second checkbox, which contains Maven, after unchecking the first.Â
Finally, click the Finish button to complete the project setup. Look at the project directory structure below to see how we'll add new packages and Java classes to the project.Â
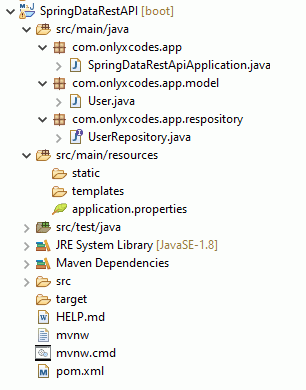
pom.xml
Spring Boot Starter JPA, Spring Boot Data REST Starter, Spring Boot Starter Web, and MySQL connection dependencies are all configured automatically due to maven.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.1</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.onlyxcodes</groupId>
<artifactId>SpringDataRestAPI</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>SpringDataRestAPI</name>
<description>Create Rest API using Spring Data Rest</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.properties
We connect the MySQL database and configure JPA properties by following the scripts. These codes are placed in the src/main/resources/application.properties.
# MySQL database connecting utility
spring.datasource.url=jdbc:mysql://localhost:3306/springboot_data_rest_api_db
spring.datasource.username=root
spring.datasource.password=
# JPA property utility
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.show_sql=true
Make RESTful APIs for Model Class ( JPA Entities ) with Spring Data REST.
User.java
Once you've created a new Spring Boot project and launched it in your IDE, the next thing you'll require to do is construct the model class ( JPA entity ) that you'll distribute using Spring Data REST.
To go there, develop a new class named User in the com.onlyxcodes.app.model package and populate it with the following code:Â
package com.onlyxcodes.app.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name = "tbl_user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private int user_id;
private String name;
private String founder;
public int getUser_id() {
return user_id;
}
public void setUser_id(int user_id) {
this.user_id = user_id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getFounder() {
return founder;
}
public void setFounder(String founder) {
this.founder = founder;
}
}
You're creating a new JPA @Entity named User to store three distinct fields, as you'll see in the code top. The majority of these fields are identified, and their names should suffice to explain what they will contain.
The int id field is the only one that could require some explanation. This field is tagged with two annotations, as you've seen:Â
- @Id: The annotation designates the field as the User's unique identification (i.e., the primary key in the database).Â
- @GeneratedValue: This annotation informs JPA that the database must provide its value. In this scenario, the database will auto-generate (GenerationType.AUTO) this item, regardless of the technique used to do so, which varies depending on the database.Â
Note:Â If your database doesn't have a table, @Entity annotation informs Hibernate to create a table out of that class.
UserRepository.java
Following the creation of the User model class ( JPA entity ), you must concentrate on developing a class that allows you to interact with the database. You'll also need to link database activities to RESTful API endpoints so that external clients may access them. Spring Data REST excels in this area.Â
There is only one thing you need to do to map to the database and publish the operations in your API. Within the package com.onlyxcodes.app.repository, build an interface named UserRepository, and enter the following code to this.Â
package com.onlyxcodes.app.respository;
import org.springframework.data.jpa.repository.JpaRepository;
import com.onlyxcodes.app.model.User;
public interface UserRepository extends JpaRepository<User, Integer>{
}
You're prepared to start your application and start sending requests to it now that you've set up this interface.Â
Spring Boot, a framework that adheres to the rule over configuration approach, and Spring Data REST recognize when you specified an interface that extends JpaRepository and collaborate to provide a set of endpoints for you.Â
JpaRepository has JPA-related methods including flushing the persistent context and batch removing records.
CrudRepository and PagingAndSorting repository are both extended by the JPA repository. It obtains and removes entities and inherits some detectors from the crud repository, such as findOne.
It also includes various JPA-specific methods, such as batch record deletion, data flushing straight to a database base, and pagination and sorting methodologies.
Spring Boot Main Class
This is the main Spring Boot class for bootstrapping our application. The com.onlyxcodes.app package contains and executes this class.Â
For UserRepository Interface class object injection, we apply @Autowired.Â
The Command Line Runner Interface is implemented in this class. This is an interface. After the Spring Boot application has begun, it is utilized to run the code.
It is necessary to override the run method after implementing the Command Line Runner Interface.Â
Using the run method I generated two User model objects and used the save() method to insert two records. CrudRepository is in the handle of this method. This method populates the database table with records.
package com.onlyxcodes.app;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import com.onlyxcodes.app.model.User;
import com.onlyxcodes.app.respository.UserRepository;
@SpringBootApplication
public class SpringDataRestApiApplication implements CommandLineRunner {
@Autowired
private UserRepository userRepository;
public static void main(String[] args) {
SpringApplication.run(SpringDataRestApiApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
User user = new User();
user.setName("Hamid");
user.setFounder("onlyxcodes");
User user1 = new User();
user1.setName("Steve Jobs");
user1.setFounder("apple");
this.userRepository.save(user);
this.userRepository.save(user1);
}
}
Let's launch our application and the Restful APIs that Spring Data Rest exposes.Â
Normal Test ( GET Method ) :
We obtain all data for the resource in this example. Using the Postman tool, go to http://localhost:8080/. All of the accessible REST URLs will be represented by HATEOAS.
{
"_links": {
"users": {
"href": "http://localhost:8080/users{?page,size,sort}",
"templated": true
},
"profile": {
"href": "http://localhost:8080/profile"
}
}
}
_links – HATEOAS provides connections to additional related resources in this area.
Users – It's the service that Spring Data REST generates from our repository.Â
profile – It renders the additional metadata information available.Â
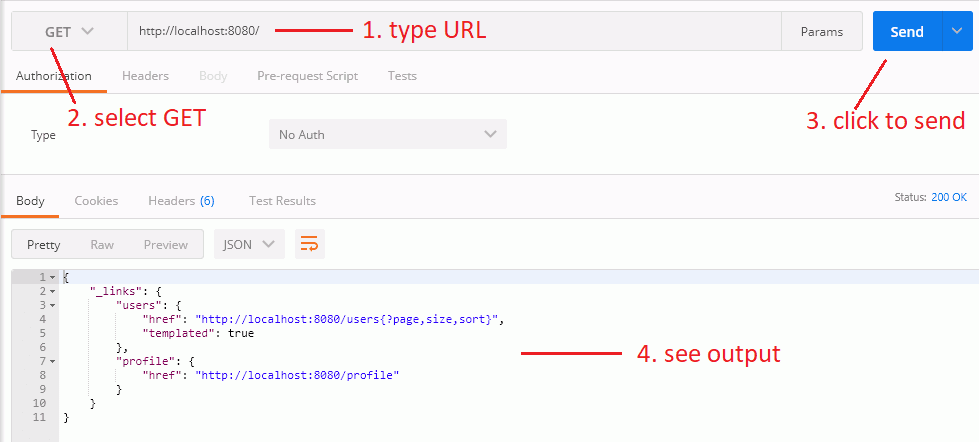
Get all user record from the table ( GET Method ):
We fetch all user records from the table in this example. Now go to http://localhost:8080/users and it will show you a list of all the users in the table.Â
{
"_embedded": {
"users": [
{
"name": "Hamid",
"founder": "onlyxcodes",
"_links": {
"self": {
"href": "http://localhost:8080/users/1"
},
"user": {
"href": "http://localhost:8080/users/1"
}
}
},
{
"name": "Steve Jobs",
"founder": "apple",
"_links": {
"self": {
"href": "http://localhost:8080/users/2"
},
"user": {
"href": "http://localhost:8080/users/2"
}
}
}
]
},
"_links": {
"self": {
"href": "http://localhost:8080/users"
},
"profile": {
"href": "http://localhost:8080/profile/users"
}
},
"page": {
"size": 20,
"totalElements": 2,
"totalPages": 1,
"number": 0
}
}
_embedded – This JSON element contains information about all users.Â
page – If we are applying pagination, this phase covers the details of the current page.Â
http://localhost:8080/users?size=2&page=0 is an example. will return the two array index records. If the page size is empty.Â
{
"_embedded": {
"users": [
{
"name": "Hamid",
"founder": "onlyxcodes",
"_links": {
"self": {
"href": "http://localhost:8080/users/1"
},
"user": {
"href": "http://localhost:8080/users/1"
}
}
},
{
"name": "Steve Jobs",
"founder": "apple",
"_links": {
"self": {
"href": "http://localhost:8080/users/2"
},
"user": {
"href": "http://localhost:8080/users/2"
}
}
}
]
},
"_links": {
"first": {
"href": "http://localhost:8080/users?page=0&size=2"
},
"self": {
"href": "http://localhost:8080/users?size=2&page=0"
},
"next": {
"href": "http://localhost:8080/users?page=1&size=2"
},
"last": {
"href": "http://localhost:8080/users?page=1&size=2"
},
"profile": {
"href": "http://localhost:8080/profile/users"
}
},
"page": {
"size": 2,
"totalElements": 3,
"totalPages": 2,
"number": 0
}
}
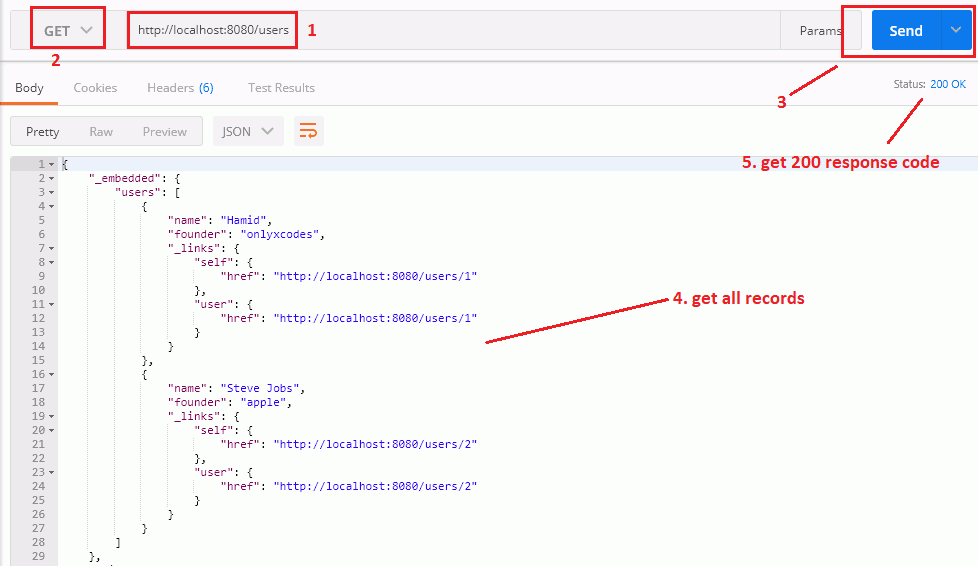
Sorting support is available at http://localhost:8080/users?sort=founder: Sort by the name of the founder. Because his founder begins with the letter "a" Steve Jobs would come before Hamid in the results.
{
"_embedded": {
"users": [
{
"name": "Steve Jobs",
"founder": "apple",
"_links": {
"self": {
"href": "http://localhost:8080/users/2"
},
"user": {
"href": "http://localhost:8080/users/2"
}
}
},
{
"name": "Hamid",
"founder": "onlyxcodes",
"_links": {
"self": {
"href": "http://localhost:8080/users/1"
},
"user": {
"href": "http://localhost:8080/users/1"
}
}
}
]
},
"_links": {
"self": {
"href": "http://localhost:8080/users?sort=founder"
},
"profile": {
"href": "http://localhost:8080/profile/users"
}
},
"page": {
"size": 20,
"totalElements": 2,
"totalPages": 1,
"number": 0
}
}
Create A New User Record ( POST Method ) :
Let's try adding a third user using the Spring Data Rest POST method because we've already generated two users in the spring boot main class.Â
Select the POST method, the raw radio button in the Body tab, set Content-Type="application/json" in the Headers tab, and paste the following JSON code using this URL http:/localhost:8080/users.Â
{
"name": "Bill Gates",
"founder": "microsoft"
}
We will receive a 201 response code and a new user created as a JSON object after executing the above post.Â
{
"name": "Bill Gates",
"founder": "microsoft",
"_links": {
"self": {
"href": "http://localhost:8080/users/4"
},
"user": {
"href": "http://localhost:8080/users/4"
}
}
}
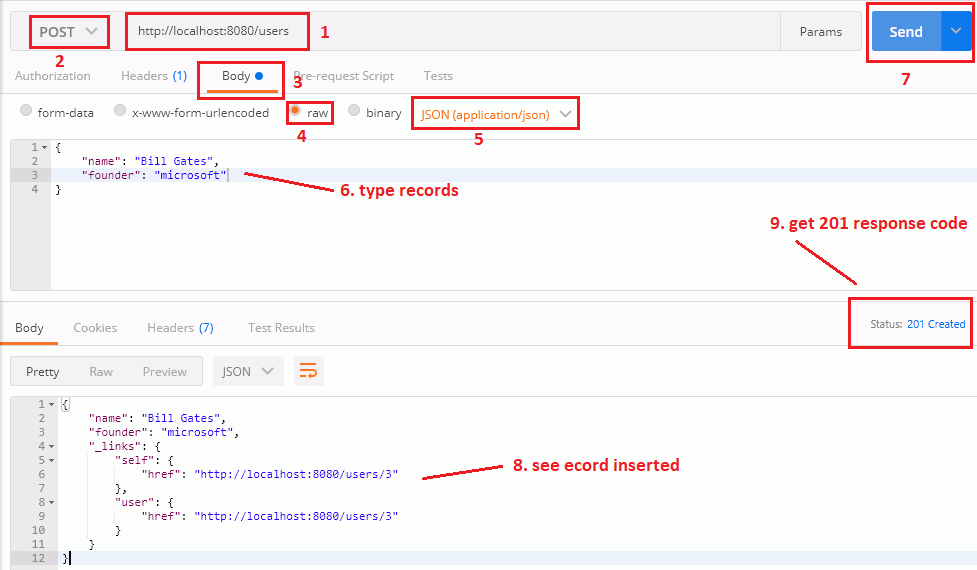
See new user also creates in the tableÂ
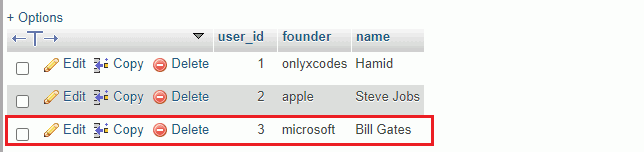
Update User Record ( PUT Method ) :
The existing user record with id 3 is updated in this example.Â
In the postman tool, go to http://localhost:8080/users/3, pick the PUT method, select the raw radio button in the Body tab, set Content-Type="application/json" in the Headers tab, and paste the JSON code below.Â
Note:Â Your table ID may be found at the end of the URL. Since I changed the 3 Id number record.Â
{
"name": "Mark Zuckerberg",
"founder": "facebook"
}
When we run the above request, we'll obtain a 200 response code along with the updated 3 id user record in JSON format.Â
{
"name": "Mark Zuckerberg",
"founder": "facebook",
"_links": {
"self": {
"href": "http://localhost:8080/users/3"
},
"user": {
"href": "http://localhost:8080/users/3"
}
}
}
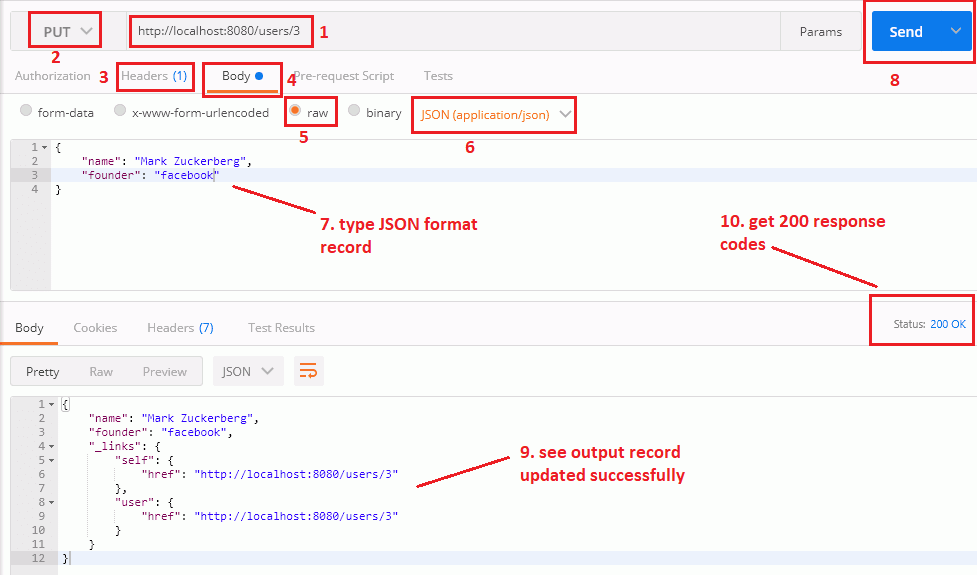
On the table, you'll also see that the 3 Id user record has been changed.Â
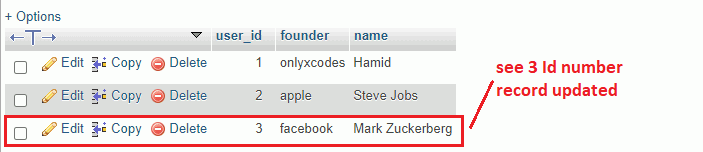
Delete User Record ( DELETE Method ) :
Lastly, in this example, we delete the user record using the URL http:/localhost:8080/users / delete/3, choose the DELETE method, and click the send button.Â
Note:Â Because I want to delete the record with the 3 Id number, your table Id is at the end of URL 3.Â
We'll get a 204 No Content response code since the user won't be available after being deleted.Â
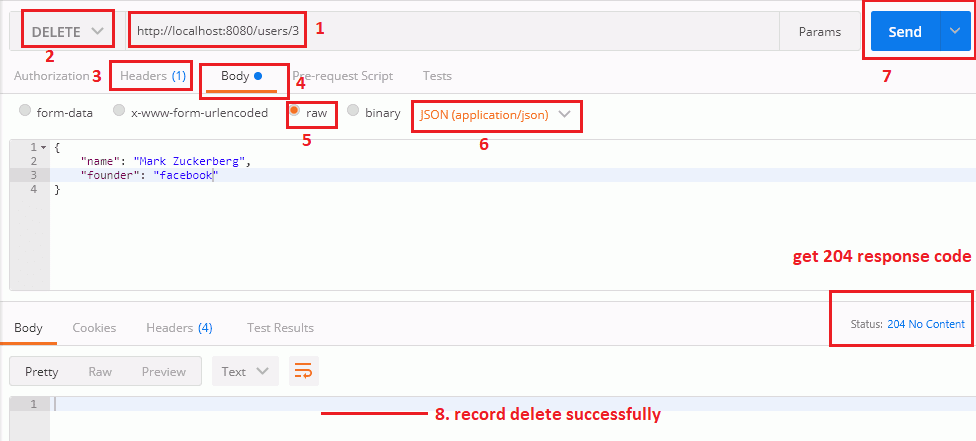
The record was also deleted from the table.Â

Download Codes
No comments:
Post a Comment