This tutorial will show you how to use the command prompt to run a Java package program.
Modern IDEs include an embedded feature that executes a program inside a package and saves the byte code of a class file when you run a Java program.
However, some folks are questioning how to compile and run a Java class manually in the command prompt once it has been put in a package.
This tutorial will walk you through the process of manually compiling and running a Java program in the command prompt.
Table Content
1. What is Package in Java?
2. Create a Package with a Program and Run using CMD
3. Types of Packages in Java
4. How to Import User-Defined Packages
5. Sub Packages in Java
6. Access Modifier in Package
7. How to Create a Package in Eclipse?
8. Points to Remember About Package
9. Advantages of Packages
1. What is Package in Java?
In Java, a package is a bundle of classes, sub-packages, and interfaces. It helps you assemble your classes into folders, making them easier to find and utilize.
In Java, every package has its name and its classes and interfaces are grouped into a separate namespace.
Interfaces and classes with the same name can't be found in the same package, although they can be found in distinct ones. This is accomplished by giving each Java package its namespace.
2. Create a Package with a Program and Run using CMD
We must first develop a program with a package before executing a package program.
To begin, paste the example below into your editor and save it to your desired location.
In my instance, the above program was saved to my H: drive. I'd like to write and compile this package program on my H: drive.
Java allows you to construct custom packages to meet your specific requirements. User-defined packages are what they're called.
The keyword package is used to define a package in Java.
package packageName;
We declare a user-defined package called mypackage in the program below.
We also add a Test name Java class file within this package.
Note:Â The Test.java file must be saved with the example below.
package mypackage;
class Test
{
public static void main(String args[])
{
System.out.println("Hello World");
}
}
Compile and Run Package Program using CMD
In this step, we use the Command Prompt(cmd) to compile and run the Test Java class file.
Select the drive where the Test.java file was created in your Command Prompt.
In my example, I moved my H: disk since I want to use it to create and assemble the above package program.
Because I created the Test.java file on my H: drive, I migrate it.
After you've moved to a certain drive, type the command below into your command prompt.
After successfully compiling the command below, it will create the mypackage (or folder) package in the current directory and store the byte code of Test.java within it.
Javac -d . Test.java
Let's look closely at the command above.
The Java compiler, javac, is responsible for properly compiling our program.
The -d option specifies the location of the created class file. You can use any directory name, such as /home (in Linux), C:/folderName (in Windows), and so on.Â
You can use the dot if you want the package to be in the same directory ( . )
The current working directory is represented by the dot "." operator.
Our class file is called Test.java.
Look at my H: the above command built the mypackage (folder) successfully.
And it also stores the Test.class file byte code.
Run the Program using Command Prompt
After the program has been compiled, run it using the command.
java mypackage.Test
Recognize the following command.
java:-Â It is the Java application launcher.
mypackage:-Â It is our package name.
Test:-Â It is our Java class file name
Output:
Hello World
        Â
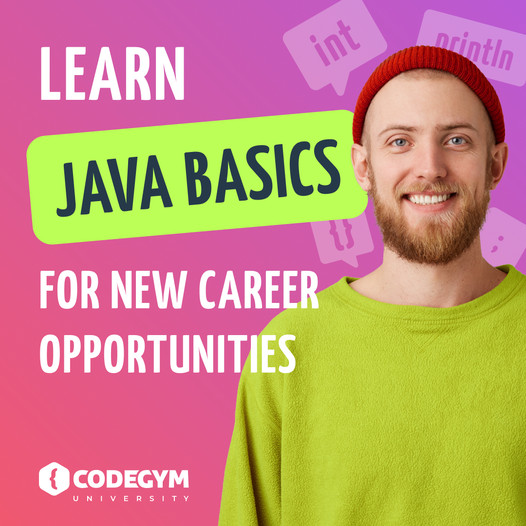
3. Types of Packages in Java
Two types of packages are available:
1) Built-in Package
2) User-Defined Package
1) Built-in Package
Existing Java packages that come with the JDK are known as built-in packages.
java.lang:Â Primitive types, strings, math functions, threads, and exceptions are all represented by classes in this library.
java.util:Â It has classes like vectors, hash tables, dates, Calendars, and so on.
java.io:Â It has input/output, and stream classes.
java.awt: Graphical User Interface (GUI) classes — windows, buttons, menus, and so forth.
java.net:Â Classes on how to network
java. Applet:Â Applet creation and implementation classes
import java.util.ArrayList;
class Fruits
{
public static void main(String args[])
{
ArrayList<String> list = new ArrayList<>(4);
list.add("apple");
list.add("orange");
list.add("mango");
list.add("banana");
System.out.println(list);
}
}
The package java.util contains the ArrayList class. We must first use the import statement to install the package before utilizing it.
import java.util.ArrayList;
Compile:
javac Fruits.java
Run:
java Fruits
Output:
[apple, orange, mango, banana]
2) User-Defined Package
In the command line argument program, we already established a user-defined package.
package packageName;
Example:
In the example below, the Hello class is now part of the demopackage package.
package demopackage;
class Hello
{
public static void main(String args[])
{
System.out.println("onlyxcodes tutorial");
}
}
Compile Program:
javac -d . Hello.java
Run Program:
java demopackage.Hello
Output:
onlyxcodes tutorial
        Â
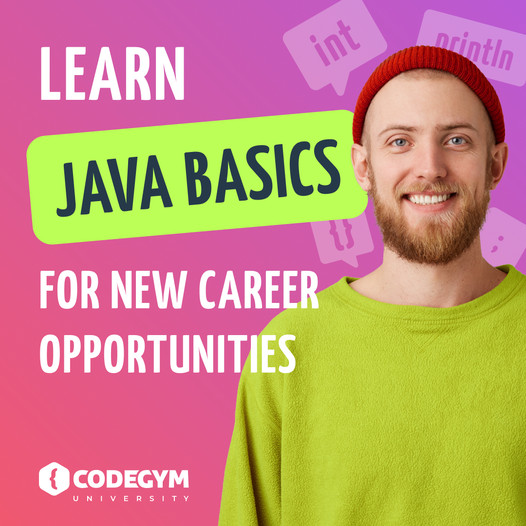
4. How to Import User-Defined PackagesÂ
In Java, you can use the import statement to import all of a package's classes and interfaces.
There are three methods to go about it:
1) Using * After the Import Statement
2) Particular Class Name (import package.classname)
3) Fully Qualified Name
1) Using * After the Import Statement
We can access all of the package's classes but not the sub-packages if we use * after the import declaration.
Syntax:
To import all of the classes, follow the command:
import packageName.*;
In the example below, we build a package in the same way we would a domain name, but in reverse order.
The number of subdirectories (package hierarchy) you can create is unlimited.
In the example below, we create a package called package1 and place the Test class name Java file into it.
I saved the Test.java file outside of package1.
package package1;
public class Test
{
public void message()
{
System.out.println("onlyxcodes tutorial");
}
}
Compile Program:
Let's run the command to compile the above program.
If the program is properly compiled, the byte code for Test.class will be stored in package1.
javac -d . Test.java
In the second example, we create a package with the name package2 instead of package1.
We also include the MainClass Java file, which contains the main method, in package2.
To import package1, we use an import statement, and to access the Test.class file, we use *.
We know that we can access all of package1's classes by using *.
We created an object from the Test class file and used that object to invoke the Test class's message() method.
Compile Program:
javac -d . MainClass.java
Run Program:
java package2.MainClass
Output:
onlyxcodes tutorial
2) Particular Class Name (import package.classname)
We can obtain a specific class package but not its sub-packages by providing a specific class name following the import declaration.
Syntax:
To import a specific class, use the following syntax:
import packageName.className;
I've developed a class Test inside a package named package1 in the example below.Â
package package1;
public class Test
{
public void message()
{
System.out.println("onlyxcodes tutorial");
}
}
Follow the command to compile the above program.
If the program is properly compiled, the byte code for the Test.class file will be stored in package1.
javac -d . Test.java
In the second example below, I create a package called package2.
Then, from the package1 package, I imported the Test class.
Package2 is where the MainClass is built.
We created the Test class object in the main method and used that object to invoke the message method.
package package2;
import package1.Test; //importing a particular class Test
class MainClass
{
public static void main(String args[])
{
Test obj = new Test();
obj.message();
}
}
Compile Program:
javac -d . MainClass.java
Run Program:
The command below runs the above program and displays the results.
java package2.MainClass
Output:
onlyxcodes tutorial
3) Fully Qualified Name
We can retrieve a defined class/interface of various packages without using the import statement by using a fully qualified name.
When accessing a class or interface that is in a different package, however, you must use a properly qualified name every time.
Let's look at an example to help us understand the above concept.
In the example below, I created a package named package1 and placed the Test class inside it.
I developed a multiplication method with a return statement inside the Test class.
package package1;
public class Test
{
public int multiplication(int i, int j)
{
return i*j;
}
}
Compile Program:
javac -d . Test.java
I built a package called package2 in the second program below. I included the MainClass, which contains the main method, in this package.
I retrieve the Test class from package1 and create the Test class object within the main method.
I used the multiplication() method with its object and gave two values for multiplication output.
package package2;
class MainClass
{
public static void main(String args[])
{
package1.Test obj = new package1.Test();
System.out.println(obj.multiplication(10,10));
}
}
Compile Program:
javac -d . MainClass.java
Run Program:
java package2.MainClass
Output:
100
5. Sub Packages in Java
A subpackage is a package that belongs inside another package.
For example, if we establish a package within the com package, it is referred to as a subpackage.
Assume we've built another package within com with the name onlyxcodes as its subpackage.
So, if we build a class in this subpackage, it should contain the following package declaration:
We're going to make a package in the same way we're going to make a domain name but in reverse order.
For example,
package com.onlyxcodes.classname;
package com.onlyxcodes;
public class Test
{
public static void main (String args[])
{
System.out.println("onlyxcodes tutorial");
}
}
Compile Program:
javac -d .. Test.java
Run Program:
java com.onlyxcodes.Test
Output:
onlyxcodes tutorial
        Â
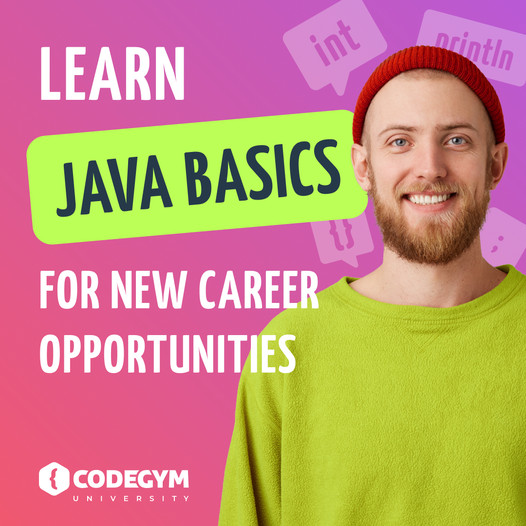
6. Access Modifier in Package
Java packages give access control to a new level. Data encapsulation can be accomplished using both classes and packages.
Classes act as containers for data and code, whereas packages operate as boxes for classes and other subordinate packages.
Java packages address four types of visibility for class members as a result of this interaction between packages and classes:
Sub-classes in the same package
Non-subclasses in the same package
Sub-classes in different packages
Classes that are neither in the same package nor sub-classes
When using packages in Java, the table indicates which types of access are possible and which are not:
Private | No Modifier | Protected | Public | |
---|---|---|---|---|
Same Class | Yes | Yes | Yes | Yes |
Same Package Subclasses | No | Yes | Yes | Yes |
Same Package Non-Subclasses | No | Yes | Yes | Yes |
Different Packages Subclasses | No | No | Yes | Yes |
Different Packages Non- Subclasses | No | No | No | Yes |
Let's first see the private access modifier.
1) Private:
Anything marked as private can only be viewed by members of that class.
In the example below, I built a package called com.onlyxcodes. I developed the A class within this package.
I declared a private method within the class.
I called the display method from within the main method using the class object.
package com.onlyxcodes;
public class A
{
private void display()
{
System.out.println("onlyxcodes tutorial");
}
public static void main (String args[])
{
A obj = new A();
obj.display();
}
}
Let's see what happens if we compile the program.
javac -d .. A.java
Okay, no errors were identified throughout the compilation process.
Run Program:
The code will display output if you run it by following the command.
java com.onlyxcodes.A
Output:
onlyxcodes tutorial
Let's see what happens if another class in the package tries to access the A-private class's method.
package com.onlyxcodes;
public class A
{
private void display()
{
System.out.println("onlyxcodes tutorial");
}
}
class B
{
public static void main (String args[])
{
A obj = new A();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Because the display method is a private part of the A class, we get the following error message.
If another class tries to access it, an error message will appear during compilation.
Output:
error: display() has private access in A
obj.display();
2) default
If no access specifier is supplied in the default access modifier, a component is visible to subclasses as well as other classes in the same package.
Let's look at an example to help us understand this principle.
A class can access a default method within the same package in the Java program below.
The default keyword is not used to assign the method in this case.
package com.onlyxcodes;
public class A
{
void display()
{
System.out.println("onlyxcodes tutorial");
}
public static void main (String args[])
{
A obj = new A();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.A
Output:
onlyxcodes tutorial
Within the same package, another class B can also access a method of the A-class.
package com.onlyxcodes;
public class A
{
void display()
{
System.out.println("onlyxcodes tutorial");
}
}
class B
{
public static void main (String args[])
{
A obj = new A();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.B
Output:
onlyxcodes tutorial
Within the same package, another class B can also access the default method of the A-class.
By using the extends keyword, the B class extends the A class.
And in this case, I generated a B class object that accessed the A-default class's display method.
package com.onlyxcodes;
public class A
{
void display()
{
System.out.println("onlyxcodes tutorial");
}
}
class B extends A
{
public static void main (String args[])
{
B obj = new B();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.B
Output:
onlyxcodes tutorial
3) Protected
A property with a protected access modifier is visible to subclasses and other classes in the same package.
Furthermore, a component is visible in another package yet is required to extend a class in the first.
Let's look at an example to help us understand this concept.
A class can access a protected method within the same package in the Java program below.
The protected keyword is applied to this method.
package com.onlyxcodes;
public class A
{
protected void display()
{
System.out.println("onlyxcodes tutorial");
}
public static void main (String args[])
{
A obj = new A();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.A
Output:
onlyxcodes tutorial
Within the same package, another class B can also access the protected method of the A-class.
package com.onlyxcodes;
public class A
{
protected void display()
{
System.out.println("onlyxcodes tutorial");
}
}
class B
{
public static void main (String args[])
{
A obj = new A();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.B
Output:
onlyxcodes tutorial
The extends keyword is used by the B class to extend the A class in the example below.
And in this case, I made a B class object that accessed the A-protected class's display method.
package com.onlyxcodes;
public class A
{
protected void display()
{
System.out.println("onlyxcodes tutorial");
}
}
class B extends A
{
public static void main (String args[])
{
B obj = new B();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.B
Output:
onlyxcodes tutorial
Another package class can access the protected member through the protected specifier.
However, the extends keyword is necessary to extend the class.
And the import keyword is necessary to import the available package name.
Let's have a look at an example.
package com.onlyxcodes;
public class A
{
protected void display()
{
System.out.println("onlyxcodes tutorial");
}
}
Compile Program:
javac -d .. A.java
I generated a second package called package2 in the example below.
The A class is then imported from the com.onlyxcodes package. The A class from the com.onlyxcode package can be imported using *.
After that, I built the C class and used the extends keyword to inherit the A class.
Finally, I used the C class object to invoke the A-display() class's method.
package package2;
import com.onlyxcodes.*;
public class C extends A
{
public static void main (String args[])
{
C obj = new C();
obj.display();
}
}
Compile Program:
javac -d . C.java
Run Program:
java package2.C
Output:
onlyxcodes tutorial
4) Public
If something is declared public in this specifier, we can retrieve it from anywhere.
In another package, a public item is also exposed.
Let's look at an example to help us understand this subject.
A class can access a public method within the same package in the Java program below.
The public keyword is used to assign the method here.
public class A
{
public void display()
{
System.out.println("onlyxcodes tutorial");
}
public static void main(String args[])
{
A obj = new A();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.A
Output:
onlyxcodes tutorial
Within the same package, another class B can also access the public method of the A-class.
package com.onlyxcodes;
public class A
{
public void display()
{
System.out.println("onlyxcodes tutorial");
}
}
class B
{
public static void main (String args[])
{
A obj = new A();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.B
Output:
onlyxcodes tutorial
The extends keyword is used by the B class to extend the A class in this example.
And in this scenario, I built a B class object that accessed the A-public class's specifier display method.
package com.onlyxcodes;
public class A
{
public void display()
{
System.out.println("onlyxcodes tutorial");
}
}
class B extends A
{
public static void main (String args[])
{
B obj = new B();
obj.display();
}
}
Compile Program:
javac -d .. A.java
Run Program:
java com.onlyxcodes.B
Output:
onlyxcodes tutorial
Another package class can acquire the public member using the public specifier.
Let's have a look at an example.
package com.onlyxcodes;
public class A
{
public void display()
{
System.out.println("onlyxcodes tutorial");
}
}
Compile Program:
javac -d .. A.java
I generated a second package named package in the example below. Inside package 2 I added the C class with the main method.
The A class is then imported from the com.onlyxcodes package. The A class from the com.onlyxcode package can be imported using *.
Finally, I used the A class object to invoke the A-display() class's method.
package package2;
import com.onlyxcodes.*;
public class C
{
public static void main (String args[])
{
A obj = new A();
obj.display();
}
}
Compile Program:
javac -d . C.java
Run Program:
java package2.C
Output:
onlyxcodes tutorial
        Â
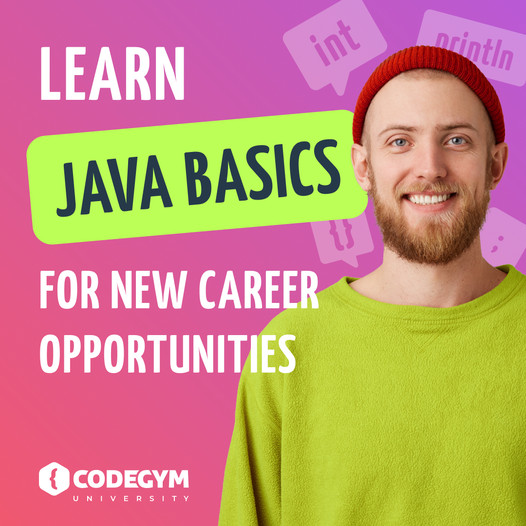
7. How to Create a Package in Eclipse?
Is here how you can make a package with Eclipse:
Select the source (src) folder with a right-click.
To get started, go to new and then package.
You'll see a pop-up window where you may type in the package name.
An equivalent directory architecture will be established on your file system after the package is formed. You can now create classes, interfaces, and other objects within the package.
8. Points to Remember About Package
The package must have the exact name of the folder where the file is saved.
A file can include many classes and interfaces that are all part of the same package.
To minimize naming problems, packages are given the reverse of the company's domain name, such as com.onlyxcodes, com.oracle, com.apple, and so on.
Although a class can only have one package declaration, it can have many package import statements.
For example:
package mypackage;
import package1;
import package2;
import package3;
Each class is part of a package. If no package is supplied, the classes in the file are moved to a special unidentified package that is shared by all files that do not belong to a package.
While importing other packages, the initial statement must be a package declaration, indicated by package import.
9. Advantages of Packages
Packages are a type of data encapsulation (or data-hiding).
To avoid name issues, we can define two classes with the same name in distinct packages.
Make it simple to find and search for classes and interfaces.
Compare the classes in different packages in a unique way.
Controlling access: package-level access control is available in both protected and default modes. Classes in the same package and its subclasses can access a protected member. Only classes in the same package have access to a default member.
Make advantage of the classes found in other programs' packages.
No comments:
Post a Comment