In this tutorial, I will show you how to display data from a database in a Bootstrap modal using JSP.
A well-liked front-end framework for creating mobile-friendly and responsive websites is called Bootstrap. It offers a collection of JavaScript and CSS elements for making slick and simple user interfaces. One popular user interface element is modal, which is a dialog window that shows up at the top of the screen to provide content or request input from the user.
I'll walk through how to quickly and simply use an Ajax call to dynamically display Oracle data in a bootstrap modal.
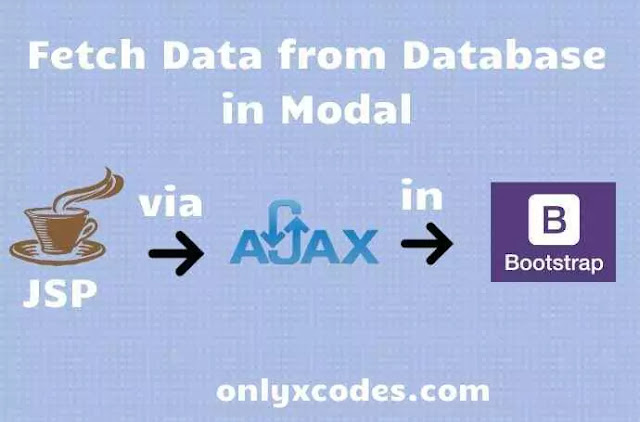
Create Table
First, I created a table called "bootstrap_modal" in my Oracle SQL command prompt.
create table bootstrap_modal(
id number,
username varchar2(13),
owner varchar2(12),
country varchar2(12)
);
Next, In this table I have inserted three dumping data using SQL insert query.
insert into bootstrap_modal (id,username,owner,country) values (1,'steve jobs','Apple','U.S.A');
insert into bootstrap_modal (id,username,owner,country) values (2,'Bill Gates','Microsoft','U.S.A');
insert into bootstrap_modal (id,username,owner,country) values (3,'Lary Page','Google','U.S.A');
Using SQL select query I verified completely inserted three dumping data.
select * from bootstrap_modal;
Display The Data
This straightforward code takes certain data from the table and displays it in an HTML table. Three data attributes are present in the view button.
The attributes data-target="#view-modal" and data-toggle="modal" work together to toggle the modal on click events, open a targeted modal with the view-modal id, and pass the id of our JSP page via Ajax. The final attribute is id="<%=rs.getInt("id")%>" that holds the table's id.
<td><button data-toggle="modal" data-target="#view-modal" id="<%=rs.getInt("id")%>" class="btn btn-sm btn-success"><i class="glyphicon glyphicon-eye-open"></i> View</button></td>
See I've full codes pasted here.
<table class="table">
<thead>
<tr>
<th>Username</th>
<th>View</th>
</tr>
</thead>
<%
String dburl="jdbc:oracle:thin:@localhost:1521:XE"; //database url string
String dbusername="system"; //database username
String dbpassword="tiger"; //database password
try
{
Class.forName("oracle.jdbc.driver.OracleDriver"); //load driver
Connection con=DriverManager.getConnection(dburl, dbusername, dbpassword); //create connection
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("SELECT * FROM bootstrap_modal"); //sql select query
ResultSet rs=pstmt.executeQuery(); //execute query and set in ResultSet object "rs"
%>
<tbody>
<%
while(rs.next())
{
%>
<tr>
<td><%=rs.getString("username")%></td>
<td><button data-toggle="modal" data-target="#view-modal" id="<%=rs.getInt("id")%>" class="btn btn-sm btn-success"><i class="glyphicon glyphicon-eye-open"></i> View</button></td>
</tr>
<%
}
%>
</tbody>
<%
}
catch(Exception e)
{
e.printStackTrace();
}
%>
</table>
All the data will be shown in this type.
As you can see, each row has a view button. When a button is clicked, a popup modal containing the specific clicked row's whole row detail will dynamically appear in the bootstrap modal.
You see when we click on the button with the id="<%=rs.getInt("id")%>" property, our Oracle database table data for that specific id (row) pops up in a modal window.

Bootstrap Modal Codes
This bootstrap modal code has the target id of data-target="#view-modal" and the id="view-modal" attribute. When the button is clicked, the following modal with the specific row's complete details in table format will appear.Â
The modal body class with id="show-data" will still load the server response, which will be in HTML format.
<div id="view-modal" class="modal fade" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true" style="display: none;">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
<h4 class="modal-title">
<i class="glyphicon glyphicon-user"></i> All User Records
</h4>
</div>
<div class="modal-body">
<!-- all data will be show here -->
<div id="show-data"></div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div><!-- /.modal -->
Ajax Request Codes
Here I specified the ajax( ) function that sends our request to the server.Â
It is the responsibility of ajax() to make bootstrap modal dynamic. The $(this).attr("id"); stores the value of id in the "uid" variable, sends it via an HTTP POST request to "getData.jsp," and receives an HTML response.
Data will be returned after a request is completed and shown in $("#show-data").html(data);.
Note:Â The attr() method returns the current id value of the table.Â
<script type="text/javascript">
$(document).ready(function(){
$('.btn-success').click(function(){
var id=$(this).attr("id");
$.ajax({
url:"getData.jsp",
type:"post",
data:"uid="+id,
success:function(data){
$("#show-data").html(data);
}
});
});
});
</script>
7. getData.jsp
This is the server file and I applied its name getData.jsp page.
To dynamically load material in a bootstrap modal with the specified ID, this file is called separately in the backend using Ajax. The content is then displayed in bootstrap modals using the $("# show-data") tag.
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.Connection"%>
<%
if(request.getParameter("uid")!=null) //get "uid" from index.jsp file jQuery ajax part this line "uid="+id".
{
int id=Integer.parseInt(request.getParameter("uid")); //get "uid" and store in new "id" variable
String dburl="jdbc:oracle:thin:@localhost:1521:XE"; //database url string
String dbusername="system"; //database username
String dbpassword="tiger"; //database password
try
{
Class.forName("oracle.jdbc.driver.OracleDriver"); //load driver
Connection con=DriverManager.getConnection(dburl,dbusername,dbpassword); //create connection
PreparedStatement pstmt=null; //create statement
pstmt=con.prepareStatement("SELECT * FROM bootstrap_modal WHERE id=?"); //sql select query
pstmt.setInt(1,id); //variable "id" set this
ResultSet rs=pstmt.executeQuery(); //execute query and set in ResultSet object "rs"
while(rs.next())
{
%>
<div class="table-responsive">
<table class="table table-striped table-bordered">
<tr>
<th>ID</th>
<td><%=rs.getInt("id")%></td>
</tr>
<tr>
<th>Username</th>
<td><%=rs.getString("username")%></td>
</tr>
<tr>
<th>Owner</th>
<td><%=rs.getString("owner")%></td>
</tr>
<tr>
<th>Country</th>
<td><%=rs.getString("country")%></td>
</tr>
</table>
</div>
<%
con.close();
}
}
catch(Exception e)
{
e.printStackTrace();
}
}
%>
Result:
When you click any button to display specific data like a username, the name of the company, or a country, all codes are implemented perfectly.
See the Modal shown below in table format.

No comments:
Post a Comment