This tutorial provides you to code Multiple Checkbox Value Add Edit and Delete with MySQL using PHP PDO.
Most beginner learners meet this query of how multiple checkbox values can be saved and updated into a single row or column of the database table. This tutorial solves all your queries by inserting, updating, and deleting multiple checkbox values when you select checkboxes and hit the submit button.
These tutorial codes are very useful for your PHP web project by selecting users to insert or edit multiple values such as hobbies, multiple topic collection, multiple question answers, and more through checkboxes, etc. Let's look at those codes.
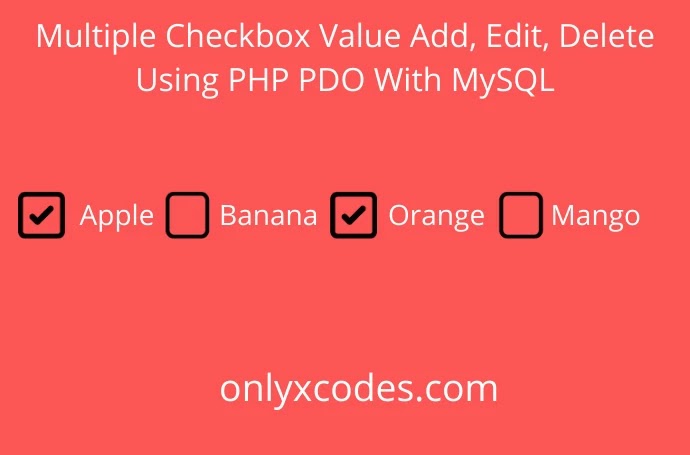
Table Content
1. Set Up Project In XAMPP Server
2. Database / Table
3. connection.php
4. add.php
    4.1 PHP Insert Code For Multiple Checkbox Value
5. edit.php
    5.1 PHP Edit Code For Multiple Checkbox Value
6. index.php
7. PHP Delete Codes For Multiple CheckBox Value
1. Set Up Project In XAMPP Server
XAMPP Server – Download I have set up and run this project in the XAMPP server.
See the complete directory structure of the project which is set up in the location of the xampp\htdocs folder.
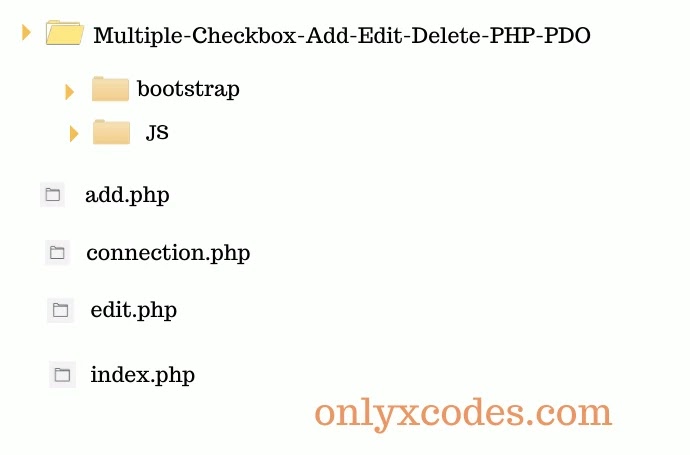
2. Database / Table
I created the fruit table in the "multiple_checkbox_db" database below, simply establish the "multiple_checkbox_db" database on your PHPMyAdmin and insert the SQL code below to create the table.
--
-- Database: `multiple_checkbox_db`
--
-- --------------------------------------------------------
--
-- Table structure for table `tbl_fruits`
--
CREATE TABLE `tbl_fruits` (
`id` int(11) NOT NULL,
`name` varchar(30) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
3. connection.php
A common basic PDO extension database configuration file.
<?php
$db_host="localhost"; //localhost server
$db_user="root"; //database username
$db_password=""; //database password
$db_name="multiple_checkbox_db"; //database name
try
{
$db=new PDO("mysql:host={$db_host};dbname={$db_name}",$db_user,$db_password);
$db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
}
catch(PDOEXCEPTION $e)
{
$e->getMessage();
}
?>
Bootstrap – I used the Bootstrap classes to build the form, and buttons as well as using different alert boxes which show success or failure messages.
4. add.php
Using Bootstrap I have created a simple insert form that contains four checkboxes and each checkbox shows different names of fruits.
Note – I assigned [] at the end of the name attribute that creates a checkbox value array and gets multiple checked checkbox values.
<form method="post" class="form-horizontal">
<div class="form-group">
<label class="col-sm-5 control-label">Hobbies</label>
<div class="col-sm-6">
<input type="checkbox" name="chk_fruit[]" value="apple">Apple
<input type="checkbox" name="chk_fruit[]" value="banana">Banana
<input type="checkbox" name="chk_fruit[]" value="orange">Orange
<input type="checkbox" name="chk_fruit[]" value="mango">Mango
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-5 col-sm-9 m-t-15">
<input type="submit" name="btn_insert" class="btn btn-success" value="Insert">
<a href="index.php" class="btn btn-danger">Cancel</a>
</div>
</div>
</form>

4.1 PHP Insert Code For Multiple Checkbox Value
If you check multiple checkboxes and click on the submit button after that this code will insert multiple selected checkbox values to the database by comma.
implode() – This function joins array elements with a string ( Source php.net )
Syntax – implode(separator, array)
The separator parameter of implode() is optional and the default is empty(" "). But here I used a comma symbol to read any values in a database table with ease.
<?php
require_once("connection.php");
if(isset($_REQUEST["btn_insert"]))
{
if(isset($_REQUEST["chk_fruit"]))
{
$fruit=implode(",",$_REQUEST["chk_fruit"]);
}
if(empty($fruit))
{
$errorMsg="Please Select Checkbox";
}
else
{
try
{
if(!isset($errorMsg))
{
$insert_stmt=$db->prepare("INSERT INTO tbl_fruits(name) VALUES(:fname)"); //sql insert query
$insert_stmt->bindParam(':fname',$fruit); //bind parameter
if($insert_stmt->execute())
{
$insertMsg="Insert Successfullt....."; //execute query success message
header("refresh:3;index.php"); //refresh 3 second and after redirect to index.php page
}
}
}
catch(PDOException $e)
{
echo $e->getMessage();
}
}
}
?>
See multiple selected values inserted perfectly into the table.

5. edit.php
This file shows checkbox checked column values that are selected for editing from the user.
explode() – This function split a string by a string.
Syntax – explode(separator, string,limit) Â
The function explode() splits up a string into an array.
Here the explode() function splits all values of a specific row that occurred by the symbol of a comma.
See understanding the simple logic of editing how it works. Â Â
First I have created an array and within this array, I have stored constantly the same fruit names as the style of insert form.
Apply foreach() loop, Within this loop, if the condition checks the array values of the specific table row and the new array values match then show checkbox checked values within this condition.Â
else condition shows unchecked values and creates new value array.Â
Note – The table row array values index by the variable $divide and the new array value index by the variable $result that refers to the $fruit.
Again, don't forget to add [ ] bracket end to each naming attribute in the checkbox.Â
<form method="post" class="form-horizontal">
<div class="form-group">
<label class="col-sm-5 control-label">Hobbies</label>
<div class="col-sm-6">
<?php
$divide=explode(",",$row["name"]);
$fruit=array("apple","banana","orange","mango");
foreach($fruit as $result)
{
if($result==$divide[0] OR $result==$divide[1] OR $result==$divide[2] OR $result==$divide[3])
{
?>
<input checked="checked" type="checkbox" name="chk_fruit[]" value="<?php echo $result;?>"><?php echo $result;?>
<?php
}
else
{
?>
<input type="checkbox" name="chk_fruit[]" value="<?php echo $result;?>"><?php echo $result;?>
<?php
}
}
?>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-5 col-sm-9 m-t-15">
<input type="submit" name="btn_update" class="btn btn-primary" value="Update">
<a href="index.php" class="btn btn-danger">Cancel</a>
</div>
</div>
</form>

5.1 PHP Edit Code For Multiple Checkbox Value
If we selects new checkbox values, these codes will update new values to existing column values, otherwise, the old checkbox values will be the same as they are.
Here again, I used implode() function in these codes to update new checkbox values separated by a comma symbol.
<?php
error_reporting( ~E_NOTICE ); //avoid error notice
require_once("connection.php");
if(isset($_REQUEST["update_id"]))
{
try
{
$id = $_REQUEST["update_id"]; //get "update_id" from index.php file through anchor tag operation and store in "$id" variable
$select_stmt = $db->prepare("SELECT * FROM tbl_fruits WHERE id =:id"); //sql select query
$select_stmt->bindParam(":id",$id); //bind parameter
$select_stmt->execute(); //execute query
$row = $select_stmt->fetch(PDO::FETCH_ASSOC); //fetch record and store in "$row" array variable
extract($row);
}
catch(PDOException $e)
{
$e->getMessage();
}
}
if(isset($_REQUEST['btn_update']))
{
if(isset($_REQUEST["chk_fruit"]))
{
$fruit_update=implode(",",$_REQUEST["chk_fruit"]); //checkbox name "chk_fruit"
}
if(empty($fruit_update))
{
$errorMsg="Please Select Checkbox Fruit";
}
else
{
try
{
if(!isset($errorMsg))
{
$update_stmt=$db->prepare('UPDATE tbl_fruits SET name=:fruit_up WHERE id=:id'); //sql update query
$update_stmt->bindParam(':fruit_up',$fruit_update);
$update_stmt->bindParam(':id',$id); //bind all parameter
if($update_stmt->execute()) //execute query
{
$updateMsg="Record Update Successfully......."; //update success message
header("refresh:3;index.php"); //refresh 3 second and after redirect to index.php page
}
}
}
catch(PDOException $e)
{
echo $e->getMessage();
}
}
}
?>
6. index.php
In this file, I fetch all checkbox values from the database and show them in the table.
<table class="table table-striped table-bordered table-hover">
<thead>
<tr>
<th>Name</th>
<th>Edit</th>
<th>Delete</th>
</tr>
</thead>
<tbody>
<?php
$select_stmt=$db->prepare("SELECT * FROM tbl_fruits"); //sql select query
$select_stmt->execute();
while($row=$select_stmt->fetch(PDO::FETCH_ASSOC))
{
?>
<tr>
<td><?php echo $row["name"]; ?></td>
<td><a href="edit.php?update_id=<?php echo $row["id"]; ?>" class="btn btn-warning">Edit</a></td>
<td><a href="?delete_id=<?php echo $row["id"]; ?>" class="btn btn-danger">Delete</a></td>
</tr>
<?php
}
?>
</tbody>
</table>

7. PHP Delete Code for Multiple Checkbox Value
See below codes beginning in index.php file above <! DOCTYPE html> tag. When you clicks on a delete hyperlink, all values from the database will be removed from the particular column.
<?php
require_once ("connection.php");
if(isset($_REQUEST['delete_id']))
{
// select record from db to delete
$id=$_REQUEST['delete_id']; //get delete_id though anchor tag operation and store in $id variable
$select_stmt= $db->prepare('SELECT * FROM tbl_fruits WHERE id =:id'); //sql select query
$select_stmt->bindParam(':id',$id); //bind parameter
$select_stmt->execute();
$row=$select_stmt->fetch(PDO::FETCH_ASSOC);
//delete an orignal record from db
$delete_stmt = $db->prepare('DELETE FROM tbl_fruits WHERE id =:id');
$delete_stmt->bindParam(':id',$id);
$delete_stmt->execute();
header("Location:index.php");
}
?>
Learn More PDO Tutorials:
How to upload an image to a database using PHP PDO
How to Upload, Insert, Update and Delete File Using PHP PDO & MySQL
Multi User Role Based Login in PHP with MySQL PDO
Add to Cart and Checkout Code in PHP PDO
Login and Register Script In PHP PDO With MySQL
Download Codes
No comments:
Post a Comment