In this article, I will teach you how to use jQuery Ajax method to upload files to the server using PHP. On this blog, I've already written the tutorial on how to upload files.
However, in this post, I used the Ajax method, which allows us to upload files to the server more rapidly. The Ajax approach improves the user experience by allowing the webserver to respond significantly faster. It just refreshes active components in real-time, rather than reloading the entire page.
I used the PHP script at the back-end side which handles the file uploading process which is sent from the Ajax () function.

Table Content
1. Creating Database
2. Project Directory Structure
3. Database Connection Utility
4. Create Simple HTML Form
5. Include jQuery library
6. Implement Ajax Code
7. PHP Script for File Uploading
8. Demo of Testing Application
Creating Database
Open your phpMyAdmin and create a new database with the name that you want. The SQL query for creating the database is mentioned below. I used the database called jquery_fileupload_db.
create database jquery_fileupload_db;
After establishing the database, you can select the below SQL code and run it to rapidly construct the file table with two fields id and filename.
CREATE TABLE `tbl_file` (
`id` int(11) NOT NULL,
`filename` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Project Directory Structure
Take a look at the project directory I have created in the XAMPP server's htdocs folder. Include this project in the www folder if you're using a WAMP server.
xampp/
├── htdocs/
├── jQuery-File-Upload-PHP/
├── bootstrap/
├── css/
│ └── bootstrap.min.js
├── js/
│ └── bootstrap.min.js
├── fonts/
│ └── glyphicons-halflings-regular.eot
│ └── glyphicons-halflings-regular.svg
│ └── glyphicons-halflings-regular.ttf
│ └── glyphicons-halflings-regular.woff
│ └── glyphicons-halflings-regular.woff2
├── js/
│ └── jquery-1.12.4-jquery.min.js
│ │
├── upload/
dbconfig.php
index.php
upload.php
You just need three files with PHP extension to upload a file to the server using Ajax jQuery method which are below.
dbconfig.php – In this file, we will connect the MySQL database.
index.php – In this file, we will create a simple HTML form that will provide a file upload option.
upload.php – In this file, we will apply our PHP code to upload files to the server.
And jQuery Ajax code to upload a file without having to reload the page.
Database Connection Utility
This is the database connection file loaded with the PDO extension, copy the mentioned script and save it to the dbconfig.php name file.
<?php
$db_host="localhost";
$db_user="root";
$db_password="";
$db_name="jquery_fileupload_db";
try
{
$db=new PDO("mysql:host={$db_host};dbname={$db_name}",$db_user,$db_password);
$db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
}
catch(PDOEXCEPTION $e)
{
$e->getMessage();
}
?>
Create Simple HTML Form
I used the bootstrap package to create a simple HTML form within the index.php file that allows a user to upload file or image to the server.
In this form, I have made a file upload input type file field and an upload button for submitting selected files to the server.
I have included a division tag after the upload button. Look at the id attribute value "img_result" on this div tag. The uploaded file or image will preview in this div tag.
In the next step, we'll submit this form request using an Ajax method.
<form class="form-horizontal">
<div class="form-group">
<label class="col-sm-3 control-label">Choose File</label>
<div class="col-sm-6">
<input type="file" name="file" id="userfile" class="form-control"/>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-3 col-sm-6 m-t-15">
<input type="button" id="btn_upload" class="btn btn-success btn-lg btn-block" value="Upload">
</div>
</div>
<div class="form-group">
<div id="img_result" class="col-sm-offset-3 col-sm-6 m-t-15" >
</div>
</div>
</form>
Look at the visual shows of this form below this style.

Include jQuery library
The jQuery library is necessary before beginning the Ajax code. Look at the jQuery library code below, which I included before closing the index.php file's </head> tag.
<script src="js/jquery-1.12.4-jquery.min.js"></script>
Implement Ajax Code
After the closing </body> tag in the index.php file, the JavaScript Ajax code begins below.
Inside the code below, I've generated a jQuery button click event because when the upload button is clicked, it triggers the action.
Next, I built the FormData() object and supplied the current form to it. You can also supply the form id instead of this.form.
The $.ajax() method uses the POST method to deliver the formData object request to the "upload.php" file. If an image is successfully uploaded, it will display a preview of the image along with the words uploaded successfully.
<script type="text/javascript">
$(document).ready(function(){
$('#btn_upload').click(function(e){
e.preventDefault();
var file = $('#userfile').val();
if(file=='')
{
alert ('Please Select File !!! ');
}
var formData = new FormData(this.form);
$.ajax({
url:'upload.php',
type:'post',
data:formData,
contentType:false,
processData:false,
success:function(response){
$('#img_result').html(response);
$('#userfile').val('');
}
});
});
});
</script>
More Explanation:
The upload button's id attribute is #btn_upload. This is how the button click event is triggered.
I used the preventDefault() method to disable the upload button from being clicked by default when the form is submitted.
The #userfile id attribute of the input type file is used by the .val() method to obtain the file value. The value of this attribute is saved in a file variable.
If the file value is blank as a result of the condition check, an alert popup with the relevant error message appears.
The FormData object is then created, and we use it to capture our form and submit it using the Ajax procedure.
I assigned the formData object to the data property in the $.ajax() method.
You can use multipart/form-data or set contentType to false. The FormData object is encoded and sent using the Content-Type: multipart/form-data encoding, which enables the sending of files.
According to the documentation for jQuery, the default value for processData is true, which means it will process and turn the data into a query string. This is set to false because we don't want to do it.
Within this <div id="img_result" > tag, the success function displays an uploaded image with a successful message. Because the id attribute value of this div tag is img_result.
PHP Script for File Uploading
This is the upload.php file, which runs in the background and communicates with the server via the ajax method.
That file simply contains PHP code that will use ajax to upload file to the directory "upload/." I've added file upload validation so that it will only upload valid extension files.
<?php
require_once "dbconfig.php";
if($_FILES['file']['name'] != '')
{
$fileName = $_FILES['file']['name'];
$tempPath = $_FILES['file']['tmp_name'];
$fileSize = $_FILES['file']['size'];
$upload_path = 'upload/'; // set upload folder path
$fileExt = strtolower(pathinfo($fileName,PATHINFO_EXTENSION)); // get image extension
// valid image extensions
$valid_extensions = array('jpeg', 'jpg', 'png', 'gif');
// allow valid image file formats
if(in_array($fileExt, $valid_extensions))
{
//check file not exist our upload folder path
if(!file_exists($upload_path . $fileName))
{
// check file size '5MB'
if($fileSize < 5000000){
move_uploaded_file($tempPath, $upload_path . $fileName); // move file from system temporary path to our upload folder path
}
else{
$errorMsg = "Sorry, your file is too large, please upload 5 MB size";
echo "<div class='alert alert-danger'>
<button type='button' class='close' data-dismiss='alert'>×</button>"
.$errorMsg.
"</div>";
}
}
else
{
$errorMsg = "Sorry, file already exists check upload folder";
echo "<div class='alert alert-danger'>
<button type='button' class='close' data-dismiss='alert'>×</button>"
.$errorMsg.
"</div>";
}
}
else
{
$errorMsg = "Sorry, only JPG, JPEG, PNG & GIF files are allowed";
echo "<div class='alert alert-danger'>
<button type='button' class='close' data-dismiss='alert'>×</button>"
.$errorMsg.
"</div>";
}
// if no error caused, continue ....
if(!isset($errorMsg))
{
$stmt=$db->prepare('INSERT INTO tbl_file(filename) VALUES(:fname)'); //sql insert query
$stmt->bindParam(':fname',$fileName);
if($stmt->execute())
{
$successMsg = "File Upload Successfully........";
echo "<div class='alert alert-success alert-dismissible'>
<button type='button' class='close' data-dismiss='alert'>×</button>"
.$successMsg.
"</div>";
echo '<img src="upload/'.$fileName.'" style="width:350px; height:240px;">';
}
}
}
?>
Full Code Explanation:
Row no 3 – I have included the database's connection file.
require_once "dbconfig.php";
Row no 5 to 9 – The PHP superglobal array $_FILES[ ] stores the “file” file field key. The if condition ensures that the file name is not empty.
$fileName = $_FILES[file]['name'] – This array value determines the file's original name.
$tempPath = $_FILES['file']['tmp_name'] – The temporary location that is uploaded to the file is retrieved from the it array. Use that information to move the uploaded file into our folder's path.
$fileSize = $_FILES['file']['size'] – The file size is defined in bytes by this value of the array.
if($_FILES['file']['name'] != '')
{
$fileName = $_FILES['file']['name'];
$tempPath = $_FILES['file']['tmp_name'];
$fileSize = $_FILES['file']['size'];
Row no 11 – Create the $upload_path variable and save the path to the upload folder on the server where the image will be uploaded.
$upload_path = 'upload/'; // set upload folder path
Row no 13 – This code gets information about a file path and saves it to the variable $fileExt.
The pathinfo() function delivers information about the path to a file. Only the extension is returned by PATHINFO EXTENSION.
The strtolower() function lowercases all characters in a string.
$fileExt = strtolower(pathinfo($fileName,PATHINFO_EXTENSION)); // get image extension
Row no 16 – Create an array with the valid image extensions jpeg, jpg, png, and gif using the array() function. The variable $valid_extensions is allocated to this array.
// valid image extensions
$valid_extensions = array('jpeg', 'jpg', 'png', 'gif');
Row no 19 – With this if condition check, you can enable valid file or image formats.
Understand this,
in_array($fileExt, $valid_extensions)
The in_array() function receives the $fileExt and $valid extensions parameters.
The $fileExt variable receives information about the file, such as its name, as we reviewed in the code for Row 13.
The $valid_extensions variable contains the array values jpeg, jpg, png, and gif, which we mentioned before in the Row no 16 code.
The in_array() function first looks for file information in the $fileExt variable, then searches the $valid_extensions array for the file extension values jpeg, jpg, png, and gif.
If the condition is valid, continue.
// allow valid image file formats
if(in_array($fileExt, $valid_extensions))
{
Row no 22 – The file_exists() function is used to verify whether or not a file has been uploaded to the server.
//check file not exist our upload folder path
if(!file_exists($upload_path . $fileName))
{
Row no 25 to 27 – The file size must be at least 5 MB if the condition check is valid.
The move_uploaded_file() function will move the file from the temporary location to the upload folder path if the above conditions are met.
// check file size '5MB'
if($fileSize < 5000000){
move_uploaded_file($tempPath, $upload_path . $fileName); // move file from system temporary path to our upload folder path
}
Row no 59 to 75 – If the $errorMSG variable does not contain any errors, the prepare() work process the insert query.
The bindParam () function in the PDO insert query binds the placeholder :fname parameter. The $fileName variable holds the value of this parameter.
If the condition is true, the execute() method will run the $stmt statement. The message "Send File Successfully" includes a witch attach bootstrap alert-success box.
In addition, I've included the server directory path as well as the concinnated uploaded file name.
<?php
if(!isset($errorMsg))
{
$stmt=$db->prepare('INSERT INTO tbl_file(filename) VALUES(:fname)'); //sql insert query
$stmt->bindParam(':fname',$fileName);
if($stmt->execute())
{
$successMsg = "File Upload Successfully........";
echo "<div class='alert alert-success alert-dismissible'>
<button type='button' class='close' data-dismiss='alert'>×</button>"
.$successMsg.
"</div>";
echo '<img src="upload/'.$fileName.'" style="width:350px; height:240px;">';
}
}
?>
Note:- I have not described any of the else conditions; as you can see, they all detect a specific file uploading error message, and all messages are sent through the bootstrap alert box of the incorrect class.
All error messages assigning to the $errorMsg variable.
Demo of Testing Application
Select the file you want to upload and click the Upload button. As demonstrated below, once the file upload is complete, it will deliver a success message along with the image.

If you choose a file type that isn't listed in the condition, such as pdf or doc, an error will appear as seen below.
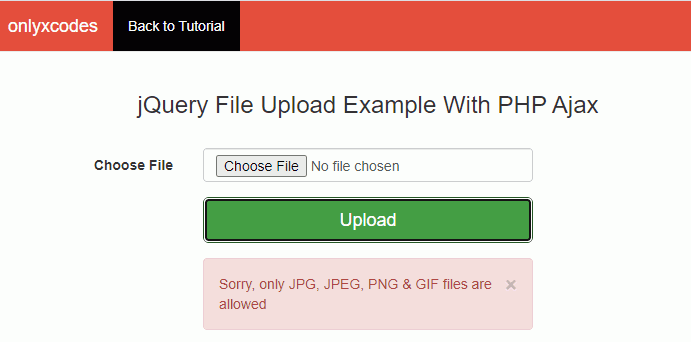
If you select an image that has already been uploaded, you will receive a file already existing error message, as shown below.

No comments:
Post a Comment