In this post, you'll learn how to submit a form using JavaScript in PHP.
AJAX, which stands for "Asynchronous JavaScript and XML," is a great way to update portions of a web page without having to reload the whole page.
Ajax communicates with the web server by sending and receiving data using the browser's integrated XMLHttpRequest (XHR) object.
In this tutorial, we will interact with the server using XMLHttpRequest and pure JavaScript, rather than the jQuery library.
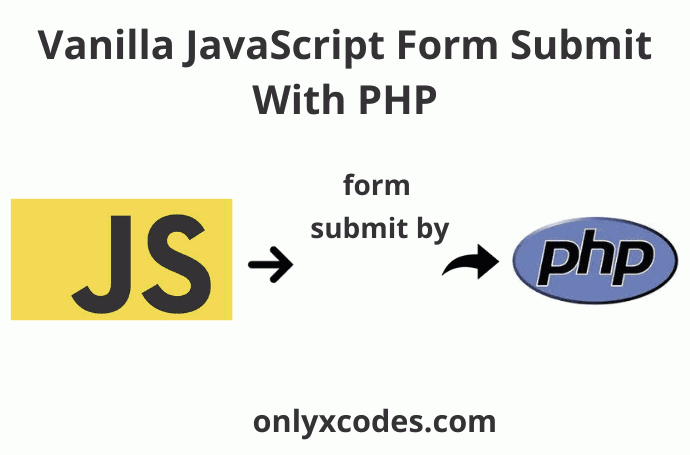
Table Content
1. Create Database And Table
2. Setup Database Connection
3. Create Registration Form
4. Implement Vanilla JavaScript Code
5. PHP Script
Create Database And Table
Create a database with any name in PhpMyAdmin and then run the SQL commands below to create a user table with five fields.
CREATE TABLE `tbl_user` (
`id` int(11) NOT NULL,
`username` varchar(30) NOT NULL,
`email` varchar(100) NOT NULL,
`password` varchar(50) NOT NULL,
`gender` varchar(7) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Setup Database Connection
This is the dbconfig.php file, which contains the database connection using the PHP PDO extension.
<?php
$db_host="localhost";
$db_user="root";
$db_password="";
$db_name="vanilla_js_form_submit_db";
try
{
$db=new PDO("mysql:host={$db_host};dbname={$db_name}",$db_user,$db_password);
$db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
}
catch(PDOEXCEPTION $e)
{
$e->getMessage();
}
?>
Let's start with JavaScript create form and submit by the PHP script.
Create Registration Form
The is the index.html file. This file has a simple client-side registration form that I designed using the bootstrap package classes.
We will submit this form-data request to a PHP script using Ajax (XMLHttpRequest) and pure Vanilla Javascript ( JS ).
Look for form_data in every field class attribute value. We obtain values from all fields by using this attribute value.
Make sure that every field that contains a span tag is closed. These span tags' id attribute value displays an appropriate error message from the server or PHP script on the client side.
The last submit button contains the submit_record() method, which we will implement in JavaScript to send the form data to the PHP script. The onclick method is used to handle the click event in this method.
return false; is the key to preventing the full HTML page from being reloaded.
<span id="message_box"></span>
<form id="registration_form" class="form-horizontal">
<div class="form-group">
<label class="col-sm-3 control-label">Userame</label>
<div class="col-sm-6">
<input type="text" class="form-control form_data" name="txt_username" placeholder="enter username" />
<span id="username_error" class="text-danger"></span>
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">Email</label>
<div class="col-sm-6">
<input type="text" class="form-control form_data" name="txt_email" placeholder="enter email" />
<span id="email_error" class="text-danger"></span>
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">Password</label>
<div class="col-sm-6">
<input type="password" class="form-control form_data" name="txt_password" placeholder="enter passowrd" />
<span id="password_error" class="text-danger"></span>
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">Select Gender</label>
<div class="col-sm-6">
<select class="form-control form_data" name="txt_gender">
<option value="" selected="selected"> --- select gender ---</option>
<option value="Male">Male</option>
<option value="Female">Female</option>
</select>
<span id="gender_error" class="text-danger"></span>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-3 col-sm-6 m-t-15">
<button type="button" id="btn_register" onclick="submit_record(); return false;" class="btn btn-success btn-lg btn-block"> Register </button>
</div>
</div>
</form>
Implement Vanilla JavaScript Code
When we click the submit button, the submit_record() function in the JavaScript below is called, and the AJAX procedure sends the form data request to the "send record.php" file and receives a response without reloading the page.
If the PHP script response is successful, it will display a register successfully message; otherwise, it will display the appropriate error message on the relevant field.
<script>
function submit_record()
{
var form_element = document.getElementsByClassName('form_data');
var fd = new FormData();
for(var i = 0; i < form_element.length; i++)
{
fd.append(form_element[i].name, form_element[i].value);
}
document.getElementById('btn_register').disabled = true;
var xhr = new XMLHttpRequest();
xhr.open('POST', 'send_record.php');
xhr.send(fd);
xhr.onreadystatechange = function()
{
if(xhr.readyState == 4 && xhr.status == 200)
{
document.getElementById('btn_register').disabled = false;
var response = JSON.parse(xhr.responseText);
if(response.success != '')
{
document.getElementById('registration_form').reset();
document.getElementById('message_box').innerHTML = response.success;
document.getElementById('username_error').innerHTML = '';
document.getElementById('email_error').innerHTML = '';
document.getElementById('password_error').innerHTML = '';
document.getElementById('gender_error').innerHTML = '';
}
else
{
//show form fields validation error messages
document.getElementById('username_error').innerHTML = response.username_error;
document.getElementById('email_error').innerHTML = response.email_error;
document.getElementById('password_error').innerHTML = response.password_error;
document.getElementById('gender_error').innerHTML = response.gender_error;
}
}
}
}
</script>
Logic Explanation:
Row No 5 – Look at the value form_data in every field's class attribute. We paste this attribute value into the document.getElementsByClassName() method, this method collects data form all fields.
var form_element = document.getElementsByClassName('form_data');
Row No 7 – Make a FormData object to hold the data that will be transmitted to the server. The fd in this case is a FormData object.
var fd = new FormData();
Row No 9 to 12 – To get all of the element names and values, use the for loop. Using the fd object of FormData, we add all fields with the specified name and value to the append() method.
for(var i = 0; i < form_element.length; i++)
{
fd.append(form_element[i].name, form_element[i].value);
}
Row No 14 – Using the document.getElementById() method, we disable the button click event. The btn_register is the register button id attribute value.
document.getElementById('btn_register').disabled = true;
Row No 16 to 20 – We create an XMLHttpRequest object and use it to send an Ajax request. The xhr is object.
The open() method takes two necessaries and three optional arguments.
The first parameter is an HTTP POST request to transfer data via this method, and the second parameter is the URL for the PHP file “send record.php,” which we will build in the next step.
In the send() method, paste the FormData object of fd, and this method will submit an Ajax request.
var xhr = new XMLHttpRequest();
xhr.open('POST', 'send_record.php');
xhr.send(fd);
Row No 22 – Create an onreadystatechange function that replies to changes in the server response. The function onreadystatechange is a callbacks function. This callback function is called whenever the request's status changes.
xhr.onreadystatechange = function()
{
Row No 24 – Take note of the XMLHttpRequest object's other properties.
The readyState parameter, for starters, provides the current condition of our request. Its value changes throughout the AJAX request and might range from 0 to 4. For example, the value 4 indicates that we have access to the response data.
Second, the status property shows whether the request was successful or not; for example, the value 200 indicates that the request was successful.
if(xhr.readyState == 4 && xhr.status == 200)
{
Row No 26 – We enable button click event using document.getElementById() method. The btn_register is button id attribute value.
document.getElementById('btn_register').disabled = false;
Row No 28 – Because the response from PHP to Javascript is in JSON string format, we need to convert (parse) it to Javascript objects and arrays.
var response = JSON.parse(xhr.responseText);
Row No 30 to 43 – If the server response is not null, the process will continue.
The reset() method uses the form's id property value to reset the form. The registration_form is the form's id attribute value. The document.getElementById() method returns the value of the form id property.
The innerHTML property displays the server's response within the span tag, which is located above the form tag.
The message_box is the id attribute value of the span tag.
Here response.success, The success is a JSON string returned by the PHP script.
After the response has been shown, the innerHTML property is set to blank for all fields.
After closing the username textbox field, the id attribute value of the span tag is username_error.
After closing the email textbox field, the email_error is the id attribute value of the span tag.
The password_error is the id attribute value of the span tag that was discovered after the password textbox field was closed.
After closing the select tag option field, the gender_error is the id attribute value of the span tag.
All id attribute value we get using the document.getElementById() method.
if(response.success != '')
{
document.getElementById('registration_form').reset();
document.getElementById('message_box').innerHTML = response.success;
document.getElementById('username_error').innerHTML = '';
document.getElementById('email_error').innerHTML = '';
document.getElementById('password_error').innerHTML = '';
document.getElementById('gender_error').innerHTML = '';
}
Row No 44 to 51 – The else condition displays the appropriate validation error message on the form fields.
I have already explained username_error, email_error, password_error, and gender_error in the last section of Row No 30 to 43. These are the span tag id attribute values that are found after each field.
The innerHTML property displays a specified error message within a span tag.
Here,
Look response.username_error, response.email_error, response.password_error and response.gender_error.
The JSON string responses username_error, email_error, password_error, and gender_error come from the PHP script.
else
{
//show form fields validation error messages
document.getElementById('username_error').innerHTML = response.username_error;
document.getElementById('email_error').innerHTML = response.email_error;
document.getElementById('password_error').innerHTML = response.password_error;
document.getElementById('gender_error').innerHTML = response.gender_error;
}
PHP Script
The Ajax request is handled by the send_record.php file. This file just contains PHP code and works silently in the backend.
This file receives each field's request via the HTTP POST method and validates it.
If everything is right, this file will add new users to the database and deliver a successful registration response to JavaScript.
<?php
require_once "dbconfig.php";
if(isset($_POST["txt_username"]))
{
$success = '';
$username = $_POST["txt_username"];
$email = $_POST["txt_email"];
$password = $_POST["txt_password"];
$gender = $_POST["txt_gender"];
$username_error = '';
$email_error = '';
$password_error = '';
$gender_error = '';
if(empty($username))
{
$username_error = 'Username is Required';
}
else
{
if(!preg_match("/^[a-zA-Z-' ]*$/", $username))
{
$username_error = 'Only Letters and White Space Allowed';
}
}
if(empty($email))
{
$email_error = 'Email is Required';
}
else
{
if(!filter_var($email, FILTER_VALIDATE_EMAIL))
{
$email_error = 'Enter Valid Email Format';
}
}
if(empty($password))
{
$password_error = 'Please enter password';
}
else
{
if(strlen($password) < 6)
{
$password_error = 'Password must be atleast 6 characters';
}
}
if(empty($gender))
{
$gender_error = 'Please Select your gender';
}
if($username_error == '' AND $email_error == '' AND $password_error == '' AND $gender_error == '')
{
$statement=$db->prepare("INSERT INTO tbl_user(username,
email,
password,
gender)
VALUES
(:username,
:useremail,
:userpassword,
:usergender)");
$statement->bindParam(':username',$username);
$statement->bindParam(':useremail',$email);
$statement->bindParam(':userpassword',$password);
$statement->bindParam(':usergender',$gender);
$statement->execute();
$success = '<div class="alert alert-success"><button type="button" class="close" data-dismiss="alert">×</button>
Register Successfully
</div>';
}
$result = array( 'success' => $success,
'username_error' => $username_error,
'email_error' => $email_error,
'password_error' => $password_error,
'gender_error' => $gender_error );
echo json_encode($result);
}
?>
Logic Explanation:
Row no 3 – Using the require_once function, add a connection file to the database. To execute PDO queries, use the database file object $db.
require_once "dbconfig.php";
Row no 5 – If condition, To access the value of the username property "txt username," use the $_POST[ ] array method. The value of this attribute is targeted by the isset() function.
if(isset($_POST["txt_username"]))
{
Row no 7 – $success is a blank variable that we create.
$success = '';
Row no 9 to 15 – Using the $_POST[ ]array method, all txt_username, txt_email, txt_password, and txt_gender values in the fields of the registration form are retrieved by the name attribute.
Get form fields that save all of the values in the variables we've generated. $gender, $username, $email, and $password.
$username = $_POST["txt_username"];
$email = $_POST["txt_email"];
$password = $_POST["txt_password"];
$gender = $_POST["txt_gender"];
Row no 17 to 20 – $username_error, $email_error, $password_error, and $gender_error are four blank variables. Our validation error message response is stored in these variables.
$username_error = '';
$email_error = '';
$password_error = '';
$gender_error = '';
Row no 22 to 32 – If both the if and else conditions are true, the username value should not be blank. The input type value for the preg replace() function must be small and capital letters; digits and special characters are not permitted.
if(empty($username))
{
$username_error = 'Username is Required';
}
else
{
if(!preg_match("/^[a-zA-Z-' ]*$/", $username))
{
$username_error = 'Only Letters and White Space Allowed';
}
}
Row no 34 to 44 – If and else condition checking valid email address format and email value not null.
filter_var – Filter a variable with a specified filter ( source php.net).
FILTER_VALIDATE_EMAIL – The FILTER_VALIDATE_EMAIL filter validates an e-mail address ( source php.net ).
if(empty($email))
{
$email_error = 'Email is Required';
}
else
{
if(!filter_var($email, FILTER_VALIDATE_EMAIL))
{
$email_error = 'Enter Valid Email Format';
}
}
Row no 46 to 56 – if and else condition checking password value not null and password length at least 6 characters must be needed.
if(empty($password))
{
$password_error = 'Please enter password';
}
else
{
if(strlen($password) < 6)
{
$password_error = 'Password must be atleast 6 characters';
}
}
Row no 58 to 61 – Using the empty() function, ensure that the select tag options values are not null if the statement is fulfilled.
if(empty($gender))
{
$gender_error = 'Please Select your gender';
}
Row no 63 – Continue if condition checks for $email, $password, and $gender do not return error messages.
if($username_error == '' AND $email_error == '' AND $password_error == '' AND $gender_error == '')
{
Row no 66 to 79 – Apply the PDO insert query within the prepare() statement.
The function bindParam() binds the values :username, :useremail, :userpassword and :usergender in the insert query. Along with $username, $email, $password, and $gender, all parameter values contain variables.
Finally, the execute() function executes the insert query statement and returns to JavaScript the register successfully message. The $success variable is assigned to this message.
The message "Register Successfully" is printed in the bootstrap success box.
$statement=$db->prepare("INSERT INTO tbl_user(username,
email,
password,
gender)
VALUES
(:username,
:useremail,
:userpassword,
:usergender)");
$statement->bindParam(':username',$username);
$statement->bindParam(':useremail',$email);
$statement->bindParam(':userpassword',$password);
$statement->bindParam(':usergender',$gender);
$statement->execute();
$success = '<div class="alert alert-success"><button type="button" class="close" data-dismiss="alert">×</button>
Register Successfully
</div>';
Row no 88 to 94 – We build the $result name array and assign all response keys to be passed to JavaScript within it.
The success, username_error, email_error, password_error, and gender_error are response keys to send to JavaScript.
All response keys hold by $username_error, $email_error, $password_error and $gender_error variables.
Because all keys responses must be sent in JSON format, the $result array is pasted into the json_encode() function.
$result = array( 'success' => $success,
'username_error' => $username_error,
'email_error' => $email_error,
'password_error' => $password_error,
'gender_error' => $gender_error );
echo json_encode($result);
Download Codes
No comments:
Post a Comment