In this lesson, we'll look at how to develop a CRUD app in PHP using the OOP (Object Oriented Programming) concept with PDO and Ajax.
I've already uploaded the PHP CRUD operation using the PDO MySQL tutorial. However, in this lesson, I've chosen an Ajax approach that allows you to insert, update, and delete data without having to reload the page.
For the front-end, I used the Bootstrap package. I used bootstrap to design the index page, forms, and a bootstrap modal to update the records.
And I used jQuery Ajax to send each request to the PHP (server) and obtain the result without having to reload the entire page. We can improve user experiences by using this method.
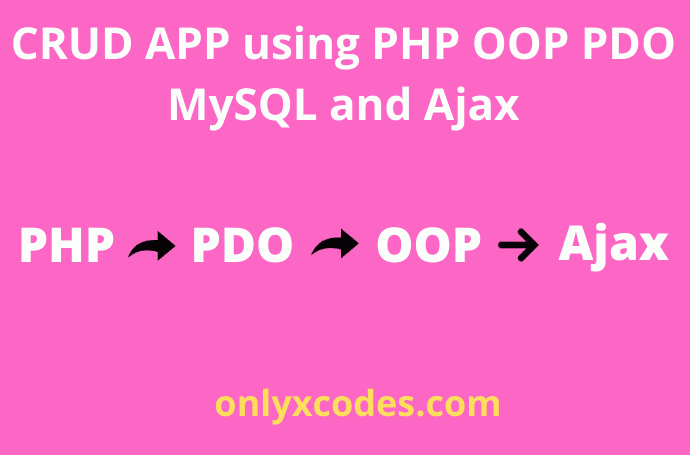
Table Content
1. Create Database And Table
2. Project Directory Structure
3. Designing Index Page
4. Create New Record
5. Read Records
6. Delete Record
7. Update Record
1. Create Database And Table
Create a database called 'crud_app_db' and import the following code into PHPMyAdmin to create a three-field student table.
CREATE TABLE IF NOT EXISTS `tbl_student` (
`student_id` int(11) NOT NULL,
`firstname` varchar(100) NOT NULL,
`lastname` varchar(100) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
2. Project Directory Structure
Our project directory structure is shown below. This project was established in the localhost XAMPP/htdocs folder. Import this project into the www folder if you're using the WAMP server.
xampp/
├── htdocs/
├── CRUD-APP-PHP-OOP-PDO-AJAX/
├── bootstrap/
├── css/
│ └── bootstrap.min.js
├── js/
│ └── bootstrap.min.js
├── fonts/
│ └── glyphicons-halflings-regular.eot
│ └── glyphicons-halflings-regular.svg
│ └── glyphicons-halflings-regular.ttf
│ └── glyphicons-halflings-regular.woff
│ └── glyphicons-halflings-regular.woff2
├── js/
│ └── jquery-1.12.4-jquery.min.js
create.php
delete.php
edit.php
index.php
model.php
read.php
update.php
3. Designing Index Page
I named the new file index.php and placed it in the root of the project directory. This file was created with Bootstrap.
I avoided designing codes and only included the file's main codes. However, all source codes are accessible in a zip file that may be downloaded at the end of this tutorial.
Look through this file. I've made a form that may be used to create or insert new records. There are two fields and an Insert button on this form. We'll use jQuery and the Ajax procedure to send this form request.
There are two divisions tags available after closing the </form> tag. The first division tag has the id attribute value message (id="message"), which displays CRUD operations for all messages without reloading the page.
Also, the second division tag id attribute value fetch (id="fetch") dynamically displays database records in table format without reloading the entire page.
<center><h2>Add Record</h2></center>
<form id="insert_form" method="post" class="form-horizontal">
<div class="form-group">
<label class="col-sm-3 control-label">Firstname</label>
<div class="col-sm-6">
<input type="text" class="form-control" id="txt_firstname" placeholder="enter firstname" />
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">Lastname</label>
<div class="col-sm-6">
<input type="text" class="form-control" id="txt_lastname" placeholder="enter lastname" />
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-3 col-sm-6 m-t-15">
<button type="submit" id="btn_create" class="btn btn-success">Insert</button>
</div>
</div>
</form>
<br />
<div class="col-lg-12">
<div id="message"></div>
<div id="fetch"></div>
</div>
Look below this type for the insert form display.
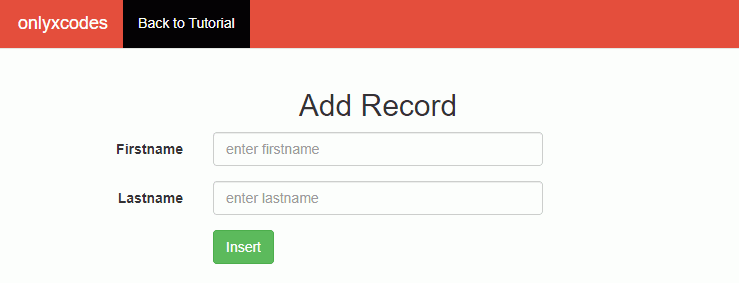
Note:Â I developed each jQuery and Ajax code to perform CRUD app operations separately in the index.php file.
4. Create New Record
To create or insert new records, we will submit the above index file form request using the jQuery Ajax technique below.
The click() method caused and triggered a button click event when users clicked on the insert button. #btn_create is the value of the insert button's id attribute.
The id attribute value of the first name text box is #txt_firstname. The val() method returns the value of the first name text box using this attribute value.
The last name text box's id attribute value is #txt_lastname. The val() method returns the value of the last name text box using this attribute value.
The val() method retrieves the value of the insert button using the #btn_create id attribute value.
If there is no problem, the $.ajax() method is called. This function's task is to use the HTTP POST method to send a form request to the "create.php" file and obtain a response from the server without refreshing the page.
Within the #message division tag, the success function shows data insert successfully message. The id attribute value of the div tag is #message.
The fetch() function is provided within the success function. This function displays database records. In the next stage, we'll create this function.
Finally, after the insertion procedure was completed, the reset() function was used to reset the form. The id attribute value of the form tag is #insert_form.
//Create New Record
$(document).on('click','#btn_create',function(e){
e.preventDefault();
var firstname = $('#txt_firstname').val();
var lastname = $('#txt_lastname').val();
var create = $('#btn_create').val();
$.ajax({
url: 'create.php',
type: 'post',
data:
{studentfirstname:firstname,
studentlastname:lastname,
insertbutton:create
},
success: function(response){
fetch();
$('#message').html(response);
}
});
$('#insert_form')[0].reset();
});
create.php
This file runs in the background on the backend and uses the ajax function to make a call.
I used the require_once function to include the model.php file in this file.
The model class contains database-related information and handles ajax requests using the OOP concept. This is the main PHP class for creating CRUD apps.
The new keyword can be used to make an object. The $model variable is an object of the Model class.
Using the above $model object, call the insert() function as follows:
The arrow (->) is an OOP construct that allows you to access the methods contained within the $model object.
If $model->insert() is called successfully, a new record is created in the table, and the data is successfully inserted, and the response is sent to the index.php file.
<?php
require_once 'model.php';
$model = new Model();
$create = $model->insert();
?>
model.php
I created a Model name class in this file. In PHP-OOP concept, this class file configures the MySQL database connection.
In this file, we'll develop six functions that will be used to make a complete CRUD operation by doing database query operations.
The magic method __construct() is provided by PHP and is invoked automatically whenever an object is created.
To the Model class, add the__construct() method as follows:
__construct() — Within the try/catch block, this function creates a database connection using the PDO extension. An exception can be handled rapidly if it is thrown.
insert() — This function gets services of ajax requests and adds new records to the database.
Within the prepare() function, a PDO insert query was applied and assigned to the $insert_stmt statement.
If the execute() function properly executes the $insert_stmt statement, the insert() function returns data inserted successfully with the attach bootstrap success box class.
Otherwise, if an issue is found, it will display an appropriate error message and attach a bootstrap alert box class.
Ajax data properties are insertbutton, studentfirstname, and studentlastname in this event.
Insertbutton has the value request for the insert button, studentfirstname holds the value request for the first name textbox, and studentlastname holds the value request for the last name textbox.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $database = 'crud_app_db';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new PDO("mysql:host=$this->host;dbname=$this->database",
$this->username, $this->password);
}
catch (PDOException $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert student new record into database and handle ajax request
public function insert()
{
if(isset($_POST['insertbutton']))
{
if(isset($_POST['studentfirstname']) && isset($_POST['studentlastname']))
{
if(!empty($_POST['studentfirstname']) && !empty($_POST['studentlastname']))
{
$firstname = $_POST['studentfirstname'];
$lastname = $_POST['studentlastname'];
$insert_stmt=$this->connection->prepare('INSERT INTO tbl_student(firstname,
lastname)
VALUES
(:fname,
:lname)');
$insert_stmt->bindParam(':fname',$firstname);
$insert_stmt->bindParam(':lname',$lastname);
if($insert_stmt->execute())
{
echo '<div class="alert alert-success">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong> Inserted : data insert successfully </strong>
</div>';
}
else
{
echo '<div class="alert alert-danger">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong> fail to insert data </strong>
</div>' ;
}
}
else
{
echo '<div class="alert alert-danger">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong> all fields are required ! </strong>
</div>' ;
}
}
}
}
//fetch all student record from database and handle ajax request
public function fetchAllRecords()
{
}
//delete student record from database and handle ajax request
public function deleteRecords()
{
}
//fetch student record from database and handle ajax request
public function edit()
{
}
//update student record and handle ajax request
public function update()
{
}
}
?>
The following is the outcome of the create operation task:
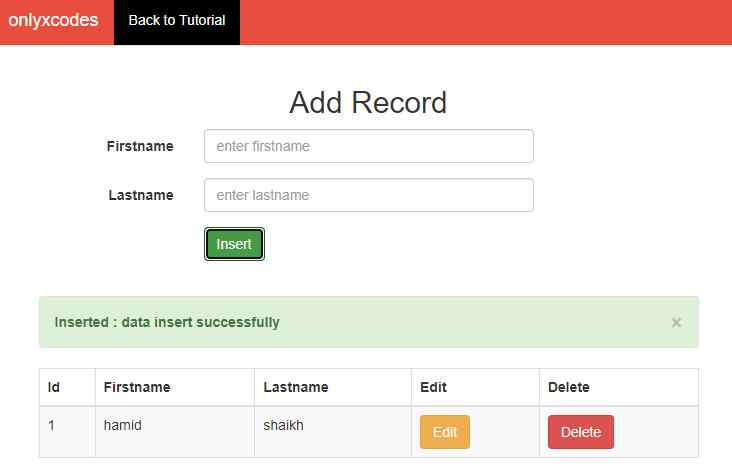
5. Read Records
After you've completed the insert process, you'll need to show the data on the index page.
I developed the fetch() function, which contains the $.ajax() function, which sends our request to the "read.php" file through the HTTP POST method and displays the record without reloading the page.
The success function displays database records as a table.
I defined div and assigned the ID fetch in the index.php file, then I used this ID (#fetch) to display the Database Record inside the HTML Table.
//Fetch All Records
function fetch(){
$.ajax({
url: 'read.php',
type: 'post',
success: function(response){
$('#fetch').html(response);
}
});
}
fetch();
read.php
This file communicates with the back-end using an ajax method and works silently.
All table records are sent to the rows array variable if $model->fetchAllRecords is executed.
This file returns a table with all of the records. Look at the table's last two properties, which have two hyperlinks: edit and delete. We will update and delete data using these hyperlinks.
<?php
require_once 'model.php';
$model = new Model();
$rows = $model->fetchAllRecords();
?>
<table class="table table-striped table-bordered table-hover">
<thead>
<tr>
<th>Id</th>
<th>Firstname</th>
<th>Lastname</th>
<th>Edit</th>
<th>Delete</th>
</tr>
</thead>
<tbody>
<?php
$i = 1;
if(!empty($rows))
{
foreach($rows as $row)
{
?>
<tr>
<td><?php echo $i++; ?></td>
<td><?php echo $row['firstname']; ?></td>
<td><?php echo $row['lastname']; ?></td>
<td><a id="edit" value="<?php echo $row['student_id']; ?>" class="btn btn-warning" data-toggle="modal" data-target="#updateModal">Edit</a></td>
<td><a id="delete" value="<?php echo $row['student_id']; ?>" class="btn btn-danger">Delete</a></td>
</tr>
<?php
}
}
?>
</tbody>
</table>
model.php class codes
The fetchAllRecords() function retrieves all of the data from the database and sends it to the page via the ajax method, which is then shown on the page.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $database = 'crud_app_db';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new PDO("mysql:host=$this->host;dbname=$this->database",
$this->username, $this->password);
}
catch (PDOException $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert student new record into database and handle ajax request
public function insert()
{
}
//fetch all student record from database and handle ajax request
public function fetchAllRecords()
{
$data = null;
$select_stmt = $this->connection->prepare('SELECT * FROM tbl_student');
$select_stmt->execute();
$data = $select_stmt->fetchAll();
return $data;
}
//delete student record from database and handle ajax request
public function deleteRecords()
{
}
//fetch student record from database and handle ajax request
public function edit()
{
}
//update student record and handle ajax request
public function update($data)
{
}
}
?>
Below this type, all records are displayed in table format:
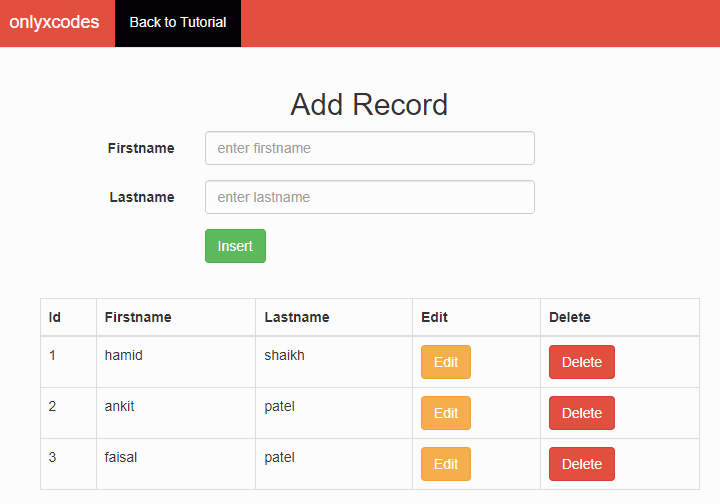
6. Delete Record
The task of deleting data is shown in this stage. The table's last property anchor tag name is delete, as seen in the read.php file. The database table id is contained in the value property of this anchor tag.
<td><a id="delete" value="<?php echo $row['student_id']; ?>" class="btn btn-danger">Delete</a></td>
The click() method caused and triggered a click event when users clicked on the delete hyperlink. The delete anchor tag's id attribute value is #delete.
To begin, a simple JavaScript confirmation modal appears in the header section, asking whether you want to delete data. If you click the yes button, the procedure will continue.
The attr() method returns the current table id value that holds by delete_id variable.
The $.ajax() function will use the HTTP POST method to submit the table id value request to the "delete.php" file and receive a response on the web page. In the #message div tag, the success function will display a data deletion message.
//Delete Record
$(document).on('click','#delete',function(e){
e.preventDefault();
if(window.confirm('are you sure to delete data'))
{
var delete_id = $(this).attr('value');
$.ajax({
url: 'delete.php',
type: 'post',
data:{studentdelete_id:delete_id},
success: function(response){
fetch();
$('#message').html(response);
}
});
}
else
{
return false;
}
});
delete.php
This file receives an Ajax HTTP POST request for a specific id value and passes it to the model class's deleteRecords() function.
The data deletion success message will be returned if the $model-> deleteRecords($delete_id) method was successfully called.
studentdelete_id is an ajax data property that is assigned to a new $delete_id variable and passed to the deleteRecords($delete_id) function in the model class.
<?php
require_once 'model.php';
$delete_id = $_POST['studentdelete_id'];
$model = new Model();
$delete = $model->deleteRecords($delete_id);
?>
model.php class codes
In the prepare() function, I used PDO delete query and set it to $delete_stmt.
When the execute() method executes $delete_stmt successfully, the deleteRecords() function returns a data deletion success message and deletes a single record from the database table.
The success message is returned by the bootstrap success box class, whereas the failure message is returned by the bootstrap alert box class.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $database = 'crud_app_db';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new PDO("mysql:host=$this->host;dbname=$this->database",
$this->username, $this->password);
}
catch (PDOException $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert student new record into database and handle ajax request
public function insert()
{
}
//fetch all student record from database and handle ajax request
public function fetchAllRecords()
{
}
//delete student record from database and handle ajax request
public function deleteRecords($delete_id)
{
$delete_stmt = $this->connection->prepare('DELETE FROM tbl_student WHERE student_id = :sid ');
$delete_stmt->bindParam(':sid',$delete_id);
if ($delete_stmt->execute())
{
echo '<div class="alert alert-success">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong> Deleted : data deleted successfully </strong>
</div>';
}
else
{
echo '<div class="alert alert-danger">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong> fail to delete data </strong>
</div>' ;
}
}
//fetch student record from database and handle ajax request
public function edit()
{
}
//update student record and handle ajax request
public function update()
{
}
}
?>
If you click on the delete hyperlink, a confirmation popup will display.
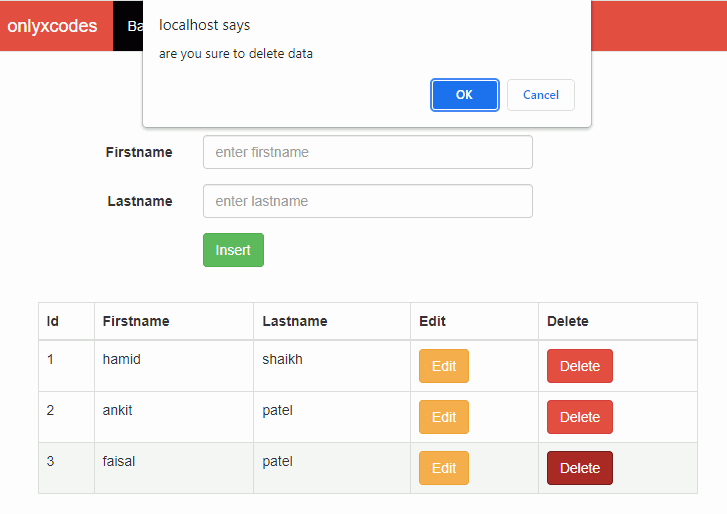
The output will be as follows if the data was deleted.
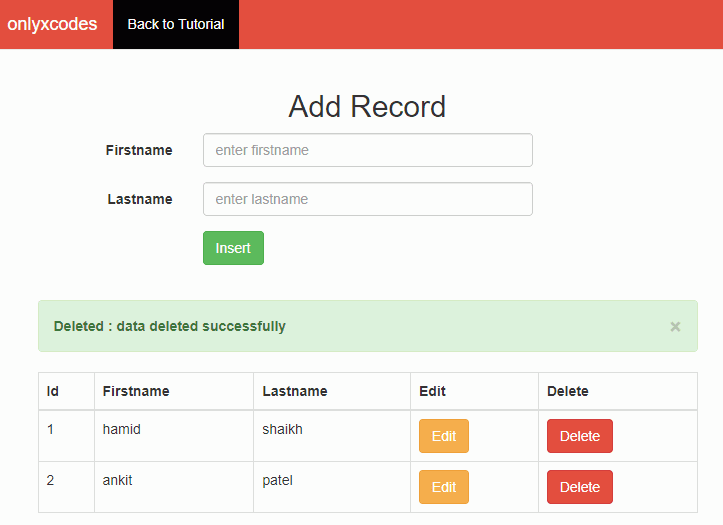
7. Update Record
You must first obtain the specific id record before continuing with the update.
The table's second last property anchor tag name is edit, as shown in the read.php file. The database table id is contained in the value property of this anchor tag.
<td><a id="edit" value="<?php echo $row['student_id']; ?>" class="btn btn-warning" data-toggle="modal" data-target="#updateModal">Edit</a></td>
Look above anchor tag also contain the two properties these are data-toggle="modal" and data-target="#updateModal".
data-toggle="modal" opens the modal window.
data-target="#updateModal" It will show up the data update modal, which will have the id in it (id="updateModal").
Bootstrap modal Discussion,
I've put the bootstrap update modal below in the index.php file separately.
Look at the modal first div tag property id="updateModal", which specifies that the modal should be opened using the data-target bootstrap modal attribute I previously mentioned.
When the user clicks the edit hyperlink, a modal containing the specific id row record appears.
Within the modal body part, the table id record is displayed. Look <div id="update_data"></div>.
In the next step, we'll open the update modal with jQuery and the Ajax procedure.
<!-- Update Modal Start-->
<div class="modal fade" id="updateModal">
<div class="modal-dialog">
<div class="modal-content">
<!-- Modal Header -->
<div class="modal-header">
<h4 class="modal-title">Update Record</h4>
<button type="button" class="close" data-dismiss="modal">×</button>
</div>
<!-- Modal body -->
<div class="modal-body">
<div id="update_data"></div>
</div>
<!-- Modal footer -->
<div class="modal-footer">
<button type="button" class="btn btn-danger" data-dismiss="modal">Close</button>
<button type="button" id="btn_update" class="btn btn-primary">Update</button>
</div>
</div>
</div>
</div>
<!-- Update Modal End-->
Ajax Codes Discussion,
When a user clicks the edit hyperlink, the click() method releases a click event, which opens the bootstrap update modal. #edit is the value of the anchor tag's id attribute.
The update id variable holds the current table id value, which is returned by the attr() method.
The $.ajax() function will use the HTTP POST method to deliver the table id value request to the "edit.php" file.
In the bootstrap modal body division tag #update_data, the success function loaded specific id row data.
The #update_data is id attribute of div tag. Look <div id="update_data"></div>.
//Get Specific Id record or Edit Record
$(document).on('click','#edit', function(e){
e.preventDefault();
var update_id = $(this).attr('value');
$.ajax({
url: 'edit.php',
type: 'post',
data: {studentupdate_id:update_id},
success: function(response){
$('#update_data').html(response);
}
});
});
edit.php
Through the $.ajax() function property HTTP POST method, this file gets a request for a specific id value and passes it to the edit() function, which is subsequently called in the model class.
We will receive a row array containing table records after $model->edit($update_id) is properly executed.
The table id is given by the studentupdate_id ajax data property, which we pass to the $update_id variable.
I've included a form for updating data in this file. Within the bootstrap modal, this form displays a specified table id row record.
Before closing the </form> tag, look for a hidden input type tag that has the specific table id. This hidden tag will be used to update a specific table id record.
<?php
require_once 'model.php';
$update_id = $_POST['studentupdate_id'];
$model = new Model();
$row = $model->edit($update_id);
if(!empty($row))
{
?>
<form id="edit_form" method="post" class="form-horizontal">
<div class="form-group">
<label class="col-sm-3 control-label">Firstname</label>
<div class="col-sm-6">
<input type="text" class="form-control" id="edit_firstname" value="<?php echo $row['firstname']; ?>" placeholder="enter firstname" />
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">Lastname</label>
<div class="col-sm-6">
<input type="text" class="form-control" id="edit_lastname" value="<?php echo $row['lastname']; ?> " placeholder="enter lastname" />
</div>
</div>
<input type="hidden" id="edit_id" value="<?php echo $row['student_id']; ?>">
</form>
<?php
}
?>
model.php class codes
After selecting and fetching table row data by id, the edit($update_id) method delivers data.
The code below is straightforward: it uses a PDO select query with a WHERE clause in the prepare() method and assigns it to the $edit_stmt statement.
The select query was used to select a specific id row record that was sent via Ajax request.
The execute() method executes the $edit_stmt statement, which is stored in the $data variable. The fetch() method retrieves the table id for each row record.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $database = 'crud_app_db';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new PDO("mysql:host=$this->host;dbname=$this->database",
$this->username, $this->password);
}
catch (PDOException $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert student new record into database and handle ajax request
public function insert()
{
}
//fetch all student record from database and handle ajax request
public function fetchAllRecords()
{
}
//delete student record from database and handle ajax request
public function deleteRecords($delete_id)
{
}
//fetch student record from database and handle ajax request
public function edit($update_id)
{
$data = null;
$edit_stmt = $this->connection->prepare('SELECT * FROM tbl_student WHERE student_id = :sid');
$edit_stmt->bindParam(':sid',$update_id);
$edit_stmt->execute();
$data = $edit_stmt->fetch();
return $data;
}
//update student record and handle ajax request
public function update()
{
}
}
?>
After you've displayed the specific record within the Modal, you'll be able to do the update operation.
Two buttons are available in the modal code footer section. The close button is the first, and the update button is the second.
The close button closes the modal, and in the second step, we'll use the update button to update the record using an ajax procedure.
<!-- Modal footer -->
<div class="modal-footer">
<button type="button" class="btn btn-danger" data-dismiss="modal">Close</button>
<button type="button" id="btn_update" class="btn btn-primary">Update</button>
</div>
jQuery and Ajax Codes Discussion,
We update specific records using the jQuery Ajax code below.
The click() method caused and triggered a button click event when users clicked on the update button. The id attribute value of the modal update button is #btn_update.
The id attribute value of the first name text box is #edit_firstname. The val() method returns the first name editable value using this attribute value.
The last name text box's id attribute value is #edit_lastname. The val() method returns the last name editable value using this attribute value.
The hidden text box's id attribute value is #edit_id. The val() method returns the table id we discussed in the edit.php file using this attribute value.
Using #btn_update id attribute value the val() method get the update button value.
Note:Â The #edit_firstname, #edit_lastname, and #edit_id were retrieved from the edit.php form. These are the edit.php file form's id attribute values.
The $.ajax() method is then called if there is no problem. This function's responsibility is to use the HTTP POST method to send an edit.php file form request to the update.php file and obtain a response from the server without having to reload the page.
Within the #message division tag, the success function displays a data update successfully message. The id property value of the div tag is #message.
//Update Record
$(document).on('click','#btn_update',function(e){
e.preventDefault();
var firstname = $('#edit_firstname').val();
var lastname = $('#edit_lastname').val();
var edit_id = $('#edit_id').val();
var update_btn = $('#btn_update').val();
$.ajax({
url: 'update.php',
type: 'post',
data:
{update_firstname:firstname,
update_lastname:lastname,
update_id:edit_id,
update_button:update_btn
},
success: function(response){
fetch();
$('#message').html(response);
}
});
});
update.php
This file communicates with the server using the $.ajax() method and an HTTP POST request. This file accepts the request to update modal form values and delivers them to the modal class, which updates the data properly.Â
The ajax data properties update_firstname, update_lastname, and update_id are used to hold edit.php file form values.
We hold table each row value in the $data array, which we paste into the model class update() function.
If $model->update($data) is done, all of the data in the table will be updated.Â
<?php
if(isset($_POST['update_button']))
{
if(isset($_POST['update_firstname']) && isset($_POST['update_lastname']) && isset($_POST['update_id']))
{
if(!empty($_POST['update_firstname']) && !empty($_POST['update_lastname']) && !empty($_POST['update_id']))
{
$data['edit_firstname'] = $_POST['update_firstname'];
$data['edit_lastname'] = $_POST['update_lastname'];
$data['edit_id'] = $_POST['update_id'];
require_once 'model.php';
$model = new Model();
$update = $model->update($data);
}
else
{
echo '<script> alert("all fields are required"); </script>' ;
}
}
}
?>
model.php class code
The update() function updated the table's row record and returned a data update response.
In the prepare() function, I used simple logic to apply a PDO update query with a WHERE clause.
The update query placeholder variables :fname, :lname, and :id are binding by the bindParam() function. These variables' values are stored in the $data array variable, which contains the table values for each row we want to update.
If the $update_stmt command is correctly executed by the execute() function, it will produce a data update successfully message with an attached bootstrap success class box.
After an updated record, the modal("hide") function hides the modal. #updateModal is the update modal's id attribute. Look at the first division tag I mentioned in the modal codes section.
If the data update operation fails, the appropriate message with the attached bootstrap alert box class will be returned.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $database = 'crud_app_db';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new PDO("mysql:host=$this->host;dbname=$this->database",
$this->username, $this->password);
}
catch (PDOException $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert student new record into database and handle ajax request
public function insert()
{
}
//fetch all student record from database and handle ajax request
public function fetchAllRecords()
{
}
//delete student record from database and handle ajax request
public function deleteRecords($delete_id)
{
}
//fetch student record from database and handle ajax request
public function edit($update_id)
{
}
//update student record and handle ajax request
public function update($data)
{
$update_stmt=$this->connection->prepare('UPDATE tbl_student SET firstname=:fname,
lastname=:lname
WHERE
student_id=:id');
$update_stmt->bindParam(':fname',$data['edit_firstname']);
$update_stmt->bindParam(':lname',$data['edit_lastname']);
$update_stmt->bindParam(':id',$data['edit_id']);
if($update_stmt->execute())
{
echo '<div class="alert alert-success">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong> Updated : data update successfully </strong>
</div>
<script> $("#updateModal").modal("hide"); </script> ';
}
else
{
echo '<div class="alert alert-danger">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong> fail to update data </strong>
</div>' ;
}
}
}
?>
The following is the outcome of the update operation:
When you click the edit hyperlink, a modal containing specific table id row records appears.
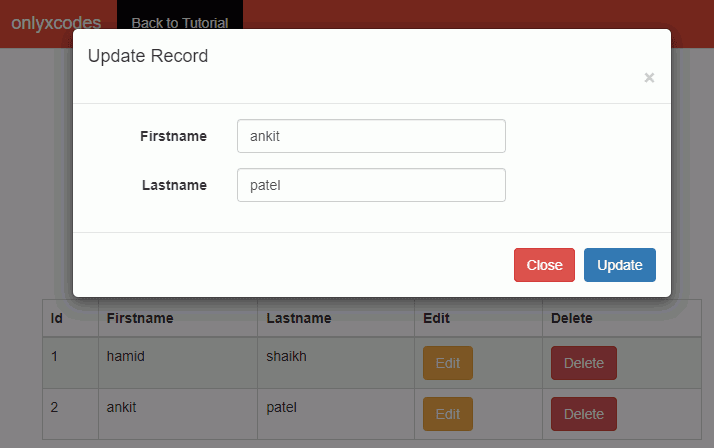
If the data has been updated, the following is the result:
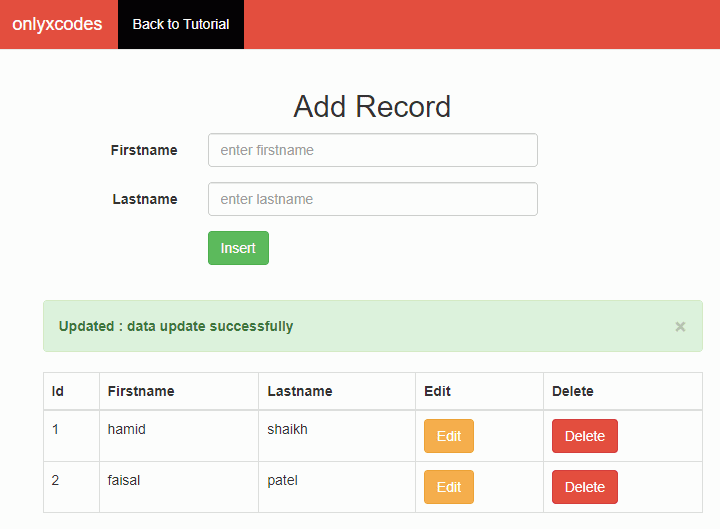
No comments:
Post a Comment