Hello and welcome to the new series. In this tutorial, you will learn how to insert, update, and delete data in CodeIgniter 4.
CodeIgniter 4 is an open-source PHP framework. CodeIgniter 4 comes with several libraries that make application development quick and easy.
We will communicate with the application database, controller, routes, model, and other components while developing CRUD (Create, Read, Update, Delete) operations in CodeIgniter 4.
This blog post will go over each section of the CRUD process in CodeIgniter 4 in great detail.
So let's start.
Table Content
1. Download Codeigniter 4
2. Activate the Development Mode
3. Errors in Codeigniter should be Enabled
4. Basic Configurations
5. Database And Table Schema
6. Database Connection
7. Create Model
8. Loading URL Helper
9. Creat Controller
9.1 Read Records
9.2 Insert Records
9.3 Update Records
9.4 Delete Records
1. Download CodeIgniter 4
To begin the insert, update, and delete operations development process, you must first manually download the CodeIgniter 4 framework.
Extract the zip file in your root where you want to add the project, If you are using XAMP let’s add in xamp\htdocs. If you are using ubuntu you can add this in /var/www or /var/www/html directory.
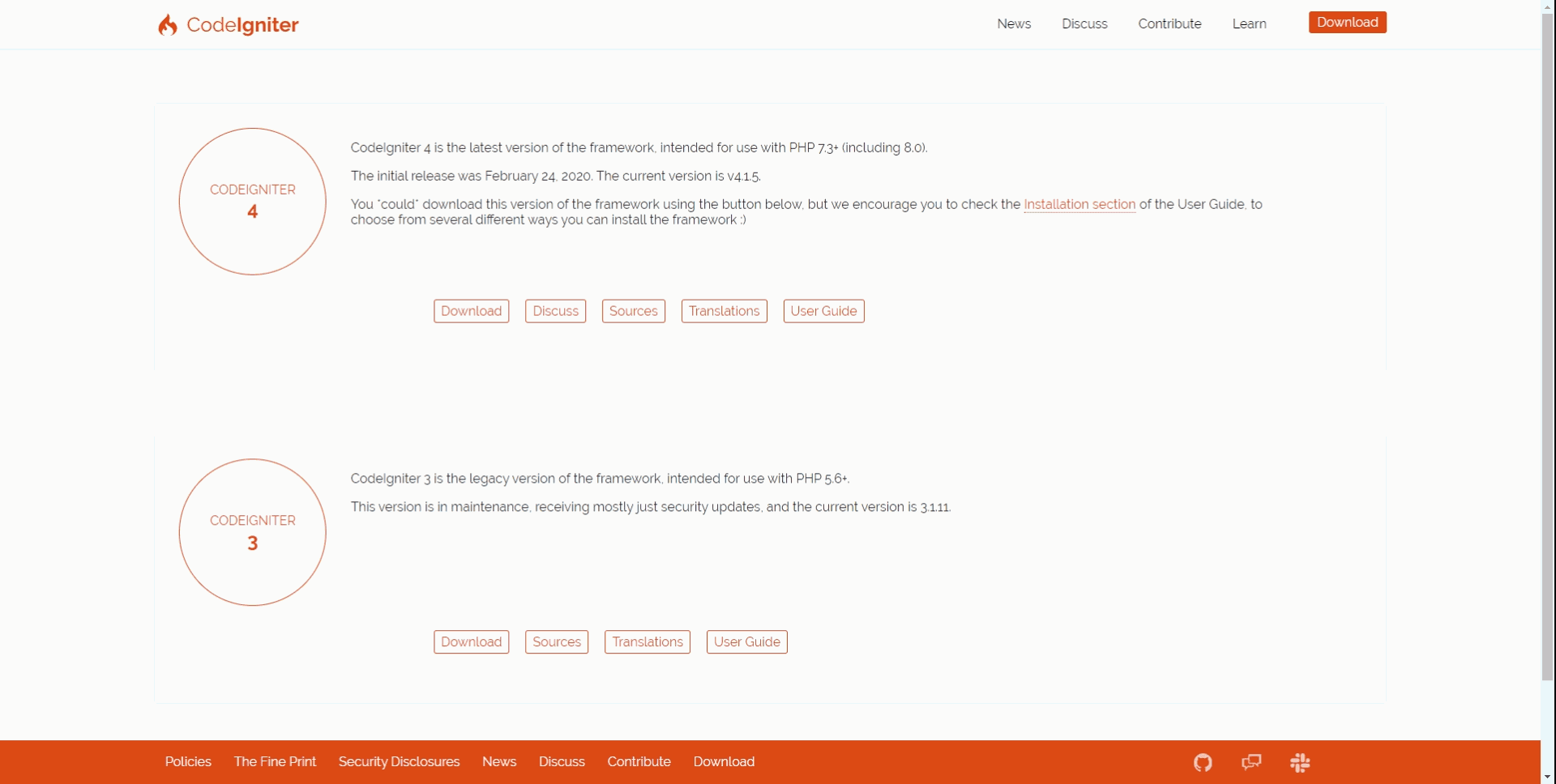
2. Activate the Development Mode
We have an env file at the root of the CodeIgniter 4 installation.
By default, CodeIgniter starts in production mode. Let's do it in development mode first. So that if we find an issue while working, an error message will appear.
#--------------------------------------------------------------------
# ENVIRONMENT
#--------------------------------------------------------------------
#CI_ENVIRONMENT = production
Remove # and update it to
#--------------------------------------------------------------------
# ENVIRONMENT
#--------------------------------------------------------------------
CI_ENVIRONMENT = development
3. Errors in Codeigniter should be Enabled
Before we begin building a CRUD application in the Codeigniter 4 framework, we must first enable Codeigniter 4 errors. This step is necessary because if we do not enable the display of errors on the web page, we will be unable to determine where the issue occurred.
So let's enable error,
Set display errors to 1 instead of 0 in the app/Config/Boot/development.php file.
error_reporting(-1);
ini_set('display_errors', '0');
to
error_reporting(-1);
ini_set('display_errors', '1');
After that, we must enter the app/Config/Boot/production.php file and change the value of display errors to 1 instead of 0.
ini_set('display_errors', '0');
to
ini_set('display_errors', '1');
4. Basic Configuration
Next, on the app/config/app.php file, you'll specify some basic setup, so navigate to app/config/app.php and open it in an editor.
Set the Base URL to this.
Then, as shown below, empty the index page "index.php" from this file. Change the public $indexPage =" " as shown below.
public $baseURL = 'http://localhost/Insert-Update-Delete-Codeigniter-4/';
/**
* --------------------------------------------------------------------------
* Index File
* --------------------------------------------------------------------------
*
* Typically this will be your index.php file, unless you've renamed it to
* something else. If you are using mod_rewrite to remove the page set this
* variable so that it is blank.
*
* @var string
*/
public $indexPage = '';
5. Database And Table Schema
You must create a database with any name at this stage. Open PHPMyAdmin and create a database there. I used "codeigniter_crud_db" named in my application.
After you've successfully created a database, run the SQL statement below to create a person table in it.
CREATE TABLE `tbl_person` (
`person_id` int(11) NOT NULL,
`firstname` varchar(50) NOT NULL,
`lastname` varchar(50) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
6. Database Connection
You must connect our project to the database in this procedure. Open the Database.php file in an editor by going to app/Config/Database.php. You must set up database connections in this file after opening it in an editor, as shown below.
<?php
public $default = [
'DSN' => '',
'hostname' => 'localhost',
'username' => 'root',
'password' => '',
'database' => 'codeigniter_crud_db',
'DBDriver' => 'MySQLi',
'DBPrefix' => '',
'pConnect' => false,
'DBDebug' => (ENVIRONMENT !== 'production'),
'charset' => 'utf8',
'DBCollat' => 'utf8_general_ci',
'swapPre' => '',
'encrypt' => false,
'compress' => false,
'strictOn' => false,
'failover' => [],
'port' => 3306,
];
7. Create Model
The components, which is an object of table values, is defined using the model. As a result, we'll need to add a new PersonModel.php file to the app/Models/ folder. To create the Person Model, add the following code to the same file.
The name of the database table must be defined in the $table variable of this class.
protected $table = 'tbl_person';
The primary key for the person table must be defined in the $primaryKey variable.
protected $primaryKey = 'person_id';
We must define table fields names (firstname, lastname) in the $allowedFields variable, which will be utilized for database operations.
protected $allowedFields = ['firstname','lastname'];
Complete Codes of PersonModel.php
<?php
namespace App\Models;
use CodeIgniter\Model;
class PersonModel extends Model
{
protected $DBGroup = 'default';
protected $table = 'tbl_person';
protected $primaryKey = 'person_id';
protected $useAutoIncrement = true;
protected $insertID = 0;
protected $returnType = 'array';
protected $useSoftDelete = false;
protected $protectFields = true;
protected $allowedFields = ['firstname','lastname'];
// Dates
protected $useTimestamps = false;
protected $dateFormat = 'datetime';
protected $createdField = 'created_at';
protected $updatedField = 'updated_at';
protected $deletedField = 'deleted_at';
// Validation
protected $validationRules = [];
protected $validationMessages = [];
protected $skipValidation = false;
protected $cleanValidationRules = true;
// Callbacks
protected $allowCallbacks = true;
protected $beforeInsert = [];
protected $afterInsert = [];
protected $beforeUpdate = [];
protected $afterUpdate = [];
protected $beforeFind = [];
protected $afterFind = [];
protected $beforeDelete = [];
protected $afterDelete = [];
}
8. Loading URL Helper
Search for $helpers in the app\Controllers\BaseController.php file, then add this url helper to the $helpers array.
The base_url() and site_url() functions will be loaded by this url helper.
protected $helpers = [ ];
to
protected $helpers = ['url'];
9. Creat Controller
In this section of the tutorial, we'll create a new controller called PersonController.php in the app\Controllers folder. Insert, Update, Delete, and View activities will be handled by this controller.
We'll go over each function in this controller one by one to execute CRUD operations.
9.1 Read Records:
First, we import the PersonModel class for database operations in this class file.
listAllPerson() – This is the PersonController's main function. We built an object of the PersonModel class in this method, and we used this object to get data from tbl_person using the findAll() method.
If $personModel->findAll(); is correctly executed, all data held by the $person variable will be returned.
The view() method has been used to load the list-all-person.php file using an array in this method. The allperson variable, on the other hand, causes the browser to display table data.
app/Controllers/PersonController.php
<?php
namespace App\Controllers;
use App\Models\PersonModel;
class PersonController extends BaseController
{
// view all person records
public function listAllPerson()
{
$personModel = new PersonModel();
$person = $personModel->findAll();
return view('list-all-person', [
"allperson" => $person,
]);
}
// insert new person records
public function InsertPerson()
{
}
// update existing person records
public function editPerson($id = null)
{
}
// delete person records
public function deletePerson($id = null)
{
}
}
Create a crudapp.php layout file in the /app/Views directory. This is the layout for the main. Open crudapp.php and paste the following code into it.
Note: With bootstrap package, I created all layout files.
/app/Views/crudapp.php
We'll apply this template to all view files and dynamically attach page views to their body.
Look at the renderSection() method's one argument, "body," which is the section's name. This allows us to include our body content.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<meta name="viewport" content="initial-scale=1.0, maximum-scale=2.0">
<title>Insert, Update, Delete In Codeigniter 4 - Onlyxcodes</title>
<link rel="stylesheet" href="<?= base_url('public/bootstrap/css/bootstrap.min.css') ?>">
<script src="<?= base_url('public/js/jquery-1.12.4-jquery.min.js') ?>"></script>
<script src="<?= base_url('public/bootstrap/js/bootstrap.min.js') ?>"></script>
</head>
<body>
<?= $this->renderSection("body") ?>
</body>
</html>
List Person view file,
list-all-person.php
The crudapp.php file can be extended using the extend() method.
Between $this->section("body") and $this->endSection(), append body content.
The code for $this->endSection() is available at the end of the line.
The successMsg parameter is a session key returned by the PersonController. This key displays messages for data insert, update, and delete operations.
The bootstrap success box class displays all CRUD messages.
Apply <?= base_url('add-person') ?> within the anchor tag. To open the insert form or the add-person.php file, use the href attribute.
All records are displayed within the <table> tag table.
Look at the last two columns, where there is two edit and delete hyperlinks.
The edit link takes you to the edit-person.php file, which has the edit form.
When you click on the specific records, the delete hyperlink deletes the data.
<td><a href="<?= base_url('edit-person/' . $value['person_id']); ?>" class="btn btn-warning">Edit</a></td>
<td><a href="<?= base_url('delete-person/' . $value['person_id']); ?>" class="btn btn-danger">Delete</a></td>
View file - /app/Views/list-all-person.php
<?= $this->extend("crudapp") ?>
<?= $this->section("body") ?>
<nav class="navbar navbar-default navbar-static-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#navbar" aria-expanded="false" aria-controls="navbar">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="https://www.onlyxcodes.com/">onlyxcodes</a>
</div>
<div id="navbar" class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li class="active"><a href="https://www.onlyxcodes.com/2021/11/insert-update-delete-codeigniter-4.html">Back to Tutorial</a></li>
</ul>
</div>
</div>
</nav>
<div class="wrapper">
<div class="container">
<div class="col-lg-12">
<?php
if(session()->has("successMsg"))
{
?>
<div class="alert alert-success">
<?= session("successMsg") ?>
<button type="button" class="close" data-dismiss="alert" aria-label="Close"><span aria-hidden="true">×</span></button>
</div>
<?php
}
?>
<div class="panel panel-default">
<div class="card mt-5">
<div class="panel-heading">
<a href="<?= base_url('add-person') ?>"><button class="btn btn-success"><span class="glyphicon glyphicon-plus"></span> Add Person</button></a>
</div>
<div class="panel-body">
<div class="table-responsive">
<table class="table table-striped table-bordered table-hover">
<thead>
<tr>
<th>Id</th>
<th>Firstname</th>
<th>Lastname</th>
</tr>
</thead>
<tbody>
<?php
foreach($allperson as $value)
{
?>
<tr>
<td><?php echo $value['person_id']; ?></td>
<td><?php echo $value['firstname']; ?></td>
<td><?php echo $value['lastname']; ?></td>
<td><a href="<?= base_url('edit-person/' . $value['person_id']); ?>" class="btn btn-warning">Edit</a></td>
<td><a href="<?= base_url('delete-person/' . $value['person_id']); ?>" class="btn btn-danger">Delete</a></td>
</tr>
<?php
}
?>
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<?= $this->endSection() ?>
Add Routes,
From the /app/Config folder, open Routes.php. This route should be added to it.
I commented default Home::index, which causes Codeigniter 4 to open the default index page.
Our URL route, which displays our table record, is included.
PersonController is the controller, and listAllPerson is the function that displays the table records that we covered before in the controller section.
\app\Config\Routes.php
//$routes->get('/', 'Home::index');
$routes->get('/', 'PersonController::listAllPerson'); // list all records
Below this kind, all records are graphically shown.
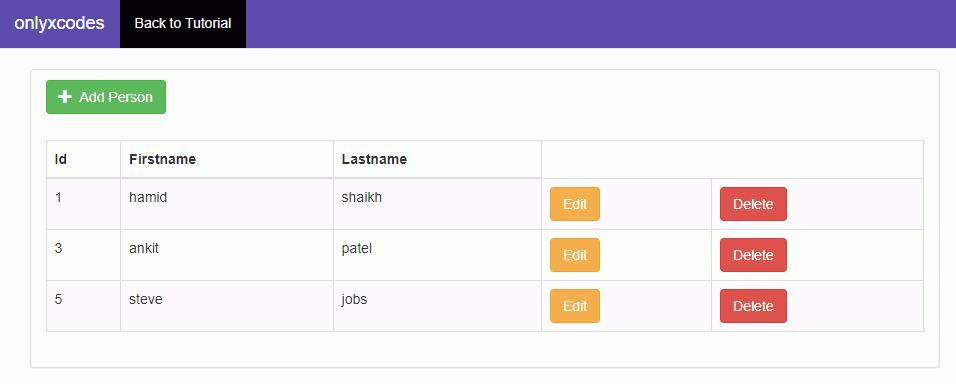
9.2 Insert Records:
How to read data from a database table was covered in the previous stage. You will now learn how to insert data into the table in this stage. To re-insert data, go to the PersonController class's source code.
InsertPerson() – To insert into the MySQL database, use this function.
app/Controllers/PersonController.php
<?php
namespace App\Controllers;
use App\Models\PersonModel;
class PersonController extends BaseController
{
// view all person records
public function listAllPerson()
{
}
// insert new person records
public function InsertPerson()
{
if ($this->request->getMethod() == "post") {
$rules = [
"txt_firstname" => "required|min_length[3]|max_length[40]",
"txt_lastname" => "required|min_length[3]|max_length[40]",
];
if (!$this->validate($rules)) {
return view('add-person', [
"validation" => $this->validator,
]);
}
else
{
$PersonModel = new PersonModel();
$data = [
'firstname' => $this->request->getVar("txt_firstname"),
'lastname' => $this->request->getVar("txt_lastname"),
];
$PersonModel->save($data);
$session = session();
$session->setFlashdata("successMsg", "New Person created successfully");
return redirect()->to(base_url('/'));
}
}
return view('add-person');
}
// update existing person records
public function editPerson($id = null)
{
}
// delete person records
public function deletePerson($id = null)
{
}
}
Explanation:
if ($this->request->getMethod() == “post”) – It determines whether the request is POST or GET.
$rules = [ ]; – The validation rules for the form are stored in this array.
The $rules array, which holds form validation, is applied within the validate() method. The validate() method also performs a validation check.
if (!$this->validate($rules)) { } – It validates form inputs against defined form validation criteria. When the form does not satisfy any of the rules, it returns false.
"validation" => $this->validator messages about form validity are sent to the file add-person.php.
Inside else condition,
$PersonModel is the name of the newly created PersonModel class object.
Inside $data [ ] array, To get values from a user-inputted form, utilized the getVar() method.
The name attributes of the insert form are txt_firstname and txt_lastname. The values of these variables are controlled by the firstname and lastname variables.
$data array should be included in the save() method.
If the data are valid, the save() method will create a new record in the person table.
If a record is successfully inserted, save the success message in the Session library setFlashdata() function and redirect the form to the '/' or the listAllPerson() method using the redirect() method.
We send base_url(), which holds our main page URL, to the to() method.
$session = session(); – To use, it loads the session service.
$session->setFlashdata("successMsg", "New Person created successfully"); – The session temp data is saved in the successMsg key.
Insert Form file – add-person.php
/app/Views/add-person.php
<?= $this->extend("crudapp") ?>
<?= $this->section("body") ?>
<nav class="navbar navbar-default navbar-static-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#navbar" aria-expanded="false" aria-controls="navbar">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="https://www.onlyxcodes.com/">onlyxcodes</a>
</div>
<div id="navbar" class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li class="active"><a href="https://www.onlyxcodes.com/2021/11/insert-update-delete-codeigniter-4.html">Back to Tutorial</a></li>
</ul>
</div>
</div>
</nav>
<div class="wrapper">
<div class="container">
<div class="col-lg-12">
<?php if(isset($validation)) : ?>
<div class="alert alert-danger" role="alert">
<strong><?= $validation->listErrors() ?></strong>
</div>
<?php endif; ?>
<form action="<?= base_url('add-person') ?>" method="post" class="form-horizontal">
<div class="form-group">
<label class="col-sm-3 control-label">Firstname</label>
<div class="col-sm-6">
<input type="text" name="txt_firstname" id="txt_firstname" class="form-control" placeholder="enter firstname" />
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">Lastname</label>
<div class="col-sm-6">
<input type="text" name="txt_lastname" id="txt_lastname" class="form-control" placeholder="enter lastname" />
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-3 col-sm-9 m-t-15">
<button type="submit" class="btn btn-success">Insert</button>
<a href="<?= base_url('/') ?>" class="btn btn-danger">Cancel</a>
</div>
</div>
</form>
</div>
</div>
</div>
<?= $this->endSection() ?>
Explanation:
The crudapp.php file can be extended using the extend() method.
Between $this->section("body") and $this->endSection(), submit body content (insert form).
Look $this->endSection() code is available end of line.
The InsertPerson() function returns the $validation variable. According to the rules, this displays form validation error messages.
<?= $validation->listErrors() ?> – Any error messages returned by the validator will be returned by the listErrors() function. It returns an empty string if there are no messages.
The bootstrap alert box class displays all error messages.
Look at the value of the form tag action attribute <?= base_url('add-person') ?>.
When a user clicks the insert button, the add-person URL is invoked, and the InsertPerson() function is utilized to insert new records.
Routes.php Discussion,
From the /app/Config folder, open Routes.php. Include the routes listed below.
The URL of the insert form action attribute value we discussed in the add-person.php file is add-person.
The match() method determines if the request is GET or POST.
Our controller is PersonController, and the function that adds new records is InsertPerson.
\app\Config\Routes.php
$routes->match(["get", "post"], "add-person", "PersonController::InsertPerson"); // insert new records
Below this kind is a graphic representation of the insert form:
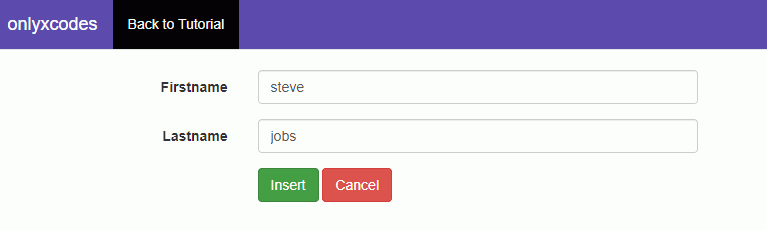
The following output will be returned after a record has been successfully inserted.
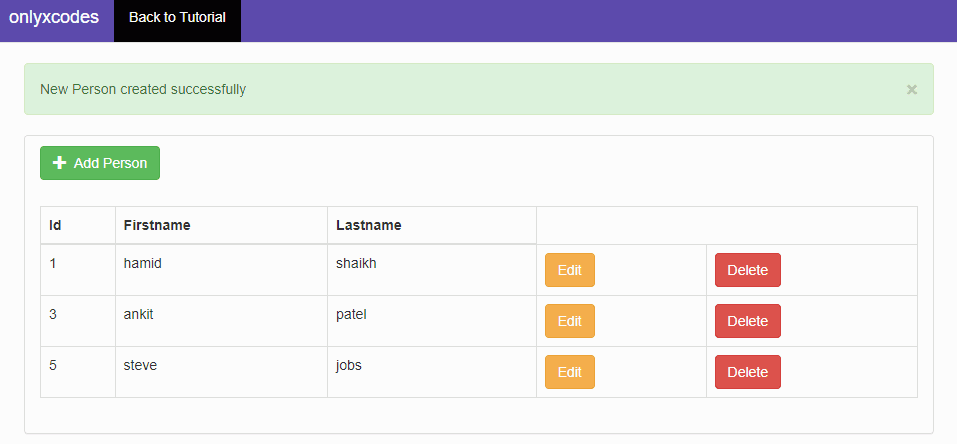
9.3 Update Records:
We now wish to update existing data after inserting it.
The file list-all-person.php was already discussed. Edit hyperlink was found in the last-second column.
<td><a href="<?= base_url('edit-person/' . $value['person_id']); ?>" class="btn btn-warning">Edit</a></td>
editPerson() – This method is used to update existing data by opening the edit form (edit-person.php).
app/Controllers/PersonController.php
<?php
namespace App\Controllers;
use App\Models\PersonModel;
class PersonController extends BaseController
{
// view all person records
public function listAllPerson()
{
}
// insert new person records
public function InsertPerson()
{
}
// update existing person records
public function editPerson($id = null)
{
$personModel = new PersonModel();
$person = $personModel->where("person_id", $id)->first();
if ($this->request->getMethod() == "post") {
$rules = [
"txt_firstname" => "required|min_length[3]|max_length[40]",
"txt_lastname" => "required|min_length[3]|max_length[40]",
];
if (!$this->validate($rules)) {
return view('edit-person', [
"validation" => $this->validator,
"person" => $person,
]);
} else {
$data = [
'firstname' => $this->request->getVar("txt_firstname"),
'lastname' => $this->request->getVar("txt_lastname"),
];
$personModel->update($id, $data);
$session = session();
$session->setFlashdata("successMsg", "Person updated successfully");
return redirect()->to(base_url('/'));
}
}
return view('edit-person', [
"person" => $person,
]);
}
// delete person records
public function deletePerson($id = null)
{
}
}
Explanation:
$personModel is an object I created. The PersonModel class's model.
$personModel->where("person_id", $id)->first() – A single row of data is read from a given id value. We also assign data to the $person variable.
if ($this->request->getMethod() == "post") – It verifies that the request type is POST.
$rules = [ ] –The edit form validation conditions are stored in this array.
The $rules array holds form validation in the validate() method. The validate() method also executes a check for validity.
if (!$this->validate($rules)) { } – It validates form inputs against defined form validation rules. When the form does not satisfy any of the rules, it returns false.
"validation" => $this->validator :– The add-person.php file receives form validation messages.
Inside else condition,
$PersonModel is the name of the created PersonModel class object.
Inside $data [ ] array, To edit existing values, I used the getVar() method.
The name attributes of the edit form are txt_firstname and txt_lastname. The values of these variables are set by the firstname and lastname variables.
In the update() method, include the $id and $data array.
If the values are valid, the update() method will be used to update an existing record in the person table.
If a record is successfully updated, save the update successful message in the Session library setFlashdata() function and redirect the form to the '/' or the listAllPerson() method using the redirect() method.
We send base_url(), which includes the URL of our main page, to the to() method.
$session = session(); It activates the session service.
$session->setFlashdata("successMsg", "Person updated successfully"); – The successMsg key stores session temporary information.
The edit-person.php file was loaded with an array using the view() method in editPerson() method. On the edit-person.php file, the person variable will display existing table data.
Edit Form file – edit-person.php
/app/Views/edit-person.php
<?= $this->extend("crudapp") ?>
<?= $this->section("body") ?>
<nav class="navbar navbar-default navbar-static-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#navbar" aria-expanded="false" aria-controls="navbar">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="https://www.onlyxcodes.com/">onlyxcodes</a>
</div>
<div id="navbar" class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li class="active"><a href="https://www.onlyxcodes.com/2021/11/insert-update-delete-codeigniter-4.html">Back to Tutorial</a></li>
</ul>
</div>
</div>
</nav>
<div class="wrapper">
<div class="container">
<div class="col-lg-12">
<?php if(isset($validation)) : ?>
<div class="alert alert-danger" role="alert">
<strong><?= $validation->listErrors() ?></strong>
</div>
<?php endif; ?>
<form action="<?= base_url('edit-person/' . $person['person_id']) ?>" method="post" class="form-horizontal">
<div class="form-group">
<label class="col-sm-3 control-label">Firstname</label>
<div class="col-sm-6">
<input type="text" name="txt_firstname" value="<?= $person['firstname'] ?>" id="txt_firstname" class="form-control"/>
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">Lastname</label>
<div class="col-sm-6">
<input type="text" name="txt_lastname" value="<?= $person['lastname'] ?>" id="txt_lastname" class="form-control" />
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-3 col-sm-9 m-t-15">
<button type="submit" class="btn btn-primary">Update</button>
<a href="<?= base_url('/') ?>" class="btn btn-danger">Cancel</a>
</div>
</div>
</form>
</div>
</div>
</div>
<?= $this->endSection() ?>
Explanation:
Expand the crudapp.php file with the extend() method.
Between $this->section("body") and $this->endSection(), put body content (edit form).
The code for $this->endSection() is available at the end of the line.
The editPerson() method returns the $validation variable. According to the regulations, this displays form validation error messages.
<?= $validation->listErrors() ?> – Any error messages returned by the validator will be returned by the listErrors() function. It returns an empty string if there are no messages.
The bootstrap alert box class displays all error information.
Look at the value of the form tag action attribute <?= base_url('edit-person/' . $person['person_id']) ?>.
The $person variable comes from the editPerson() function's view() method. This variable stores data for a single row based on an id value.
Here, person_id is the table id field.
When a user clicks the Update button, the edit-person URL is called, and the editPerson() function is utilized to update existing records.
Routes.php discussion,
From the /app/Config folder, open Routes.php. Integrate the routes listed below.
The first portion of the URL contains the edit-person, while the second segment has a number.
(:num) – will work with any integer.
The match() method analyzes if the request is GET or POST.
Our controller is PersonController, and the editPerson function modifies existing records.
\app\Config\Routes.php
$routes->match(["get", "post"], "edit-person/(:num)", "PersonController::editPerson/$1"); // update existing record
The updated form is visually displayed below this type:
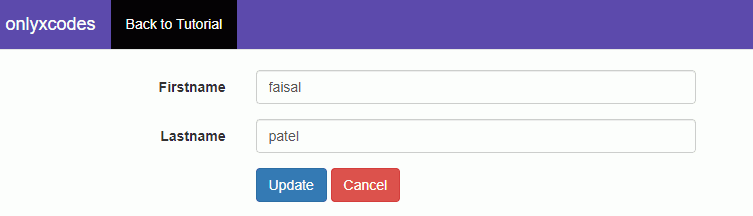
It will return the following output with the bootstrap success box class after any record is successfully updated.
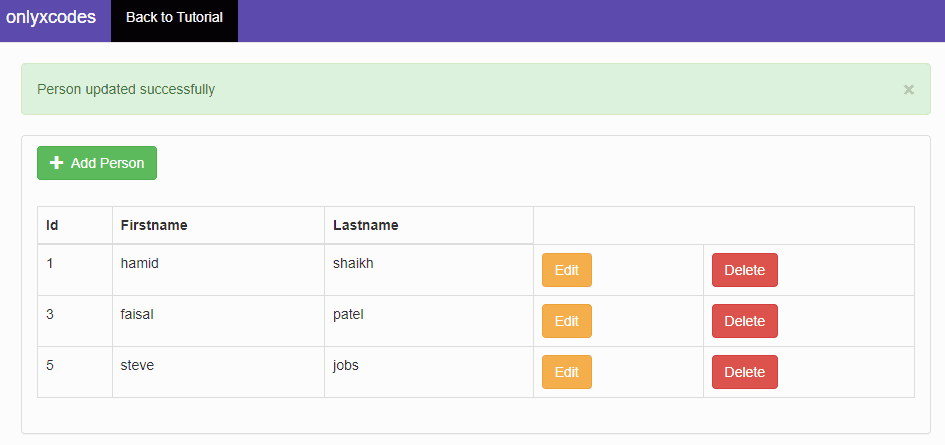
9.4 Delete Records:
This section will teach you how to delete a record.
You've previously seen the contents of the file list-all-person.php. The delete hyperlink was in the last column.
<td><a href="<?= base_url('delete-person/' . $value['person_id']); ?>" class="btn btn-danger">Delete</a></td>
deletePerson() – This function was used to delete already stored data.
app/Controllers/PersonController.php
Explanation:
The delete() method removes an existing record based on its id value.
If a record is successfully deleted, to save delete the successful message in the Session library setFlashdata() function and redirect to the '/' or the listAllPerson() method using the redirect() method.
Other codes are the same as the ones we discussed in the insert and update operations.
<?php
namespace App\Controllers;
use App\Models\PersonModel;
class PersonController extends BaseController
{
// view all person records
public function listAllPerson()
{
}
// insert new person records
public function InsertPerson()
{
}
// update existing person records
public function editPerson($id = null)
{
}
// delete person records
public function deletePerson($id = null)
{
$personModel = new PersonModel();
$person = $personModel->delete($id);
$session = session();
$session->setFlashdata("successMsg", "Person deleted successfully");
return redirect()->to(base_url('/'));
}
}
If a record is successfully deleted, the following output with the bootstrap success box class will be returned.
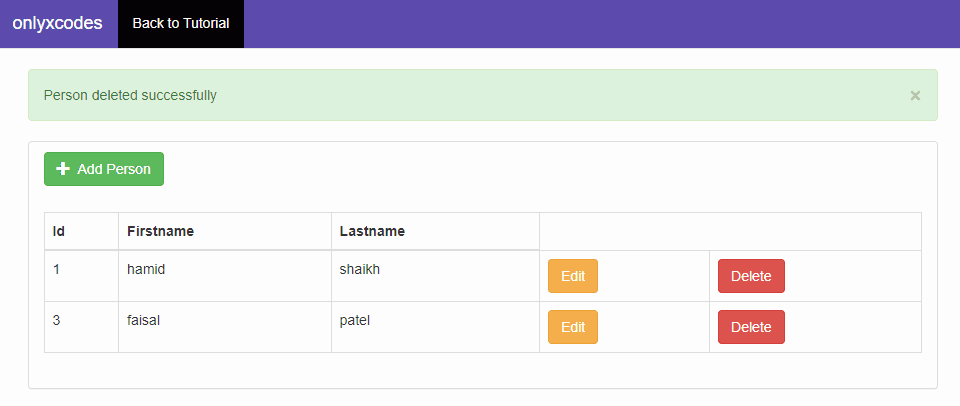
PersonController.php all codes
app/Controllers/PersonController.php
<?php
namespace App\Controllers;
use App\Models\PersonModel;
class PersonController extends BaseController
{
// view all person records
public function listAllPerson()
{
$personModel = new PersonModel();
$person = $personModel->findAll();
return view('list-all-person', [
"allperson" => $person,
]);
}
// insert new person records
public function InsertPerson()
{
if ($this->request->getMethod() == "post") {
$rules = [
"txt_firstname" => "required|min_length[3]|max_length[40]",
"txt_lastname" => "required|min_length[3]|max_length[40]",
];
if (!$this->validate($rules)) {
return view('add-person', [
"validation" => $this->validator,
]);
}
else
{
$PersonModel = new PersonModel();
$data = [
'firstname' => $this->request->getVar("txt_firstname"),
'lastname' => $this->request->getVar("txt_lastname"),
];
$PersonModel->save($data);
$session = session();
$session->setFlashdata("successMsg", "New Person created successfully");
return redirect()->to(base_url('/'));
}
}
return view('add-person');
}
// update existing person records
public function editPerson($id = null)
{
$personModel = new PersonModel();
$person = $personModel->where("person_id", $id)->first();
if ($this->request->getMethod() == "post") {
$rules = [
"txt_firstname" => "required|min_length[3]|max_length[40]",
"txt_lastname" => "required|min_length[3]|max_length[40]",
];
if (!$this->validate($rules)) {
return view('edit-person', [
"validation" => $this->validator,
"person" => $person,
]);
} else {
$data = [
'firstname' => $this->request->getVar("txt_firstname"),
'lastname' => $this->request->getVar("txt_lastname"),
];
$personModel->update($id, $data);
$session = session();
$session->setFlashdata("successMsg", "Person updated successfully");
return redirect()->to(base_url('/'));
}
}
return view('edit-person', [
"person" => $person,
]);
}
// delete person records
public function deletePerson($id = null)
{
$personModel = new PersonModel();
$person = $personModel->delete($id);
$session = session();
$session->setFlashdata("successMsg", "Person deleted successfully");
return redirect()->to(base_url('/'));
}
}
Routes.php all codes
\app\Config\Routes.php
//$routes->get('/', 'Home::index');
$routes->get('/', 'PersonController::listAllPerson'); // list all records
$routes->match(["get", "post"], "add-person", "PersonController::InsertPerson"); // insert new records
$routes->match(["get", "post"], "edit-person/(:num)", "PersonController::editPerson/$1"); // update existing record
$routes->get("delete-person/(:num)", "PersonController::deletePerson/$1"); // delete existing record
No comments:
Post a Comment