Hello folks, in this tutorial, we'll make a simple crud application with VueJS, PHP, and MySQL.On any dynamic web application, the CRUD (Create, Read, Update, and Delete) actions are required.
Although CRUD operations no longer require a page refresh on a single page, PHP requires a page refresh after each button click. We can improve the user experience without refreshing the webpage by combining Ajax with PHP script.
Creating a Vue single-page application (SPA) with PHP can provide a better user experience.
In this example, the frontend will be built with VueJS and the Axios package, while the backend will be built with PHP. The Axios package makes an HTTP call to our PHP server and receives a response in the Vue template without having to reload the page.
Let's get started with the tutorial, where I'll teach you how to create a full-stack Vue Single Page Application with PHP as the backend.
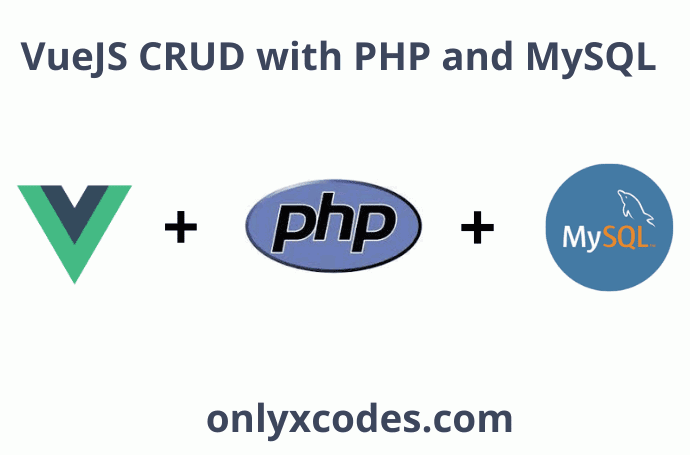
Create Table
First, we must build a table in the Mysql database. To do so, execute the following SQL script on your Mysql localhost.
However, before running the SQL script, you must first create a database, and then execute the SQL script below. It will create a table called tbl_book in your local MySQL database.
CREATE TABLE IF NOT EXISTS `tbl_book` (
`book_id` int(11) NOT NULL,
`book_name` varchar(100) NOT NULL,
`book_author` varchar(50) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
index.html
We've written everything client-side code in this file, including HTML and integrating Vue.js. To utilize Vue.js with the Axios package, we must first include the required libraries.
Before closing the </head> tag, add the CSS libraries listed below.
<!-- Bootstrap CSS -->
<link type="text/css" rel="stylesheet" href="//unpkg.com/bootstrap/dist/css/bootstrap.min.css" />
<!-- BootstrapVue CSS -->
<link type="text/css" rel="stylesheet" href="//unpkg.com/bootstrap-vue@latest/dist/bootstrap-vue.min.css" />
<!-- Bootstrap npm CSS -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-+0n0xVW2eSR5OomGNYDnhzAbDsOXxcvSN1TPprVMTNDbiYZCxYbOOl7+AMvyTG2x" crossorigin="anonymous">
Before closing the /body> tag, include the Javascript libraries mentioned below.
<!-- Vuejs -->
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<!-- BootstrapVue js -->
<script type="text/javascript" src="//unpkg.com/bootstrap-vue@latest/dist/bootstrap-vue.min.js"></script>
<!-- Axios -->
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<!-- Bootstrap js -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js" integrity="sha384-gtEjrD/SeCtmISkJkNUaaKMoLD0//ElJ19smozuHV6z3Iehds+3Ulb9Bn9Plx0x4" crossorigin="anonymous"></script>
All HTML contents are loaded in an element with the id "bookApp" in this division tag.
<div class="container" id="bookApp">
//content
</div>
Initialize VueJS
Before closing the </body> tag, VueJS is being integrated. We have an id named #bookApp, as you can see. It signifies that all of the Vue functionality has been processed, and VueJS has called in a component with the id bookApp.
<script>
var application = new Vue({
el: '#bookApp',
});
</script>
We establish Data property and methods within the Vue method after initializing the Vue part to conduct simple CRUD operations using the Axios technique.
Within the Data property, define seven variables.
Boostrap adds modal form properties to the name and author. We'll bind add modal form input fields using the v-model directive.
All records from the server will be stored in this array and shown in a tabular format. allRecords: [] is an array.
The update id, update name, and update author properties are all bindings for the bootstrap update modal form. We'll bind update modal form input fields with the v-model directive.
Within the bootstrap alert message box, the "successMsg" variable displays record insert, update, and delete messages.
The showModal() and hideModal() functions of the method use the modal references property to show and hide modal activity.
<script>
var application = new Vue({
el: '#bookApp',
data: {
name: '',
author: '',
allRecords: [],
update_id: '',
update_name: '',
update_author: '',
successMsg: ''
},
methods: {
showModal(id) {
this.$refs[id].show()
},
hideModal(id) {
this.$refs[id].hide()
},
onSubmit(){
},
getRecords(){
},
deleteRecord(id){
},
editRecord(id){
},
onUpdate(){
},
mounted: function(){
this.getRecords()
}
})
</script>
Let's take a look at the VueJS CRUD operation in this example step by step.
Create Record:
To begin, add a modal like seen above. The add button is where I put it. This modal is displayed using the @click event.
I installed bootstrap add modal underneath division sections. I included a form with two fields within this modal.
See @submit.prevent="onSubmit" in the form tag. It implies that instead of submitting the form normally, we run onSubmit. Within the Vue method, we construct an onSubmit() function that performs an Axios call to PHP to generate a record, then redirects to the table for display.
Directive v-model We have described how to bind input textbox field values in the Data property.
<div class="card-header">
<div class="d-flex d-flex justify-content-between">
<div class="lead"><button id="show-btn" @click="showModal('add-modal')" class="btn btn-sm btn-outline-primary">Add Records</button></div>
</div>
</div>
<div class="card-body">
<!-- Add Book Record Modal Start-->
<b-modal ref="add-modal" hide-footer title="Add Book Records">
<form action="" @submit.prevent="onSubmit">
<div class="form-group">
<label for="">Book Name</label>
<input type="text" v-model="name" class="form-control">
</div>
<br />
<div class="form-group">
<label for="">Author</label>
<input type="text" v-model="author" class="form-control">
</div>
<br />
<div class="form-group">
<button class="btn btn-sm btn-outline-success">Add Records</button>
<b-button class="btn btn-sm btn-outline-danger" variant="outline-danger" block @click="hideModal('add-modal')">Close
</b-button>
</div>
</form>
</b-modal>
<!-- Add Book Record Modal End -->
VueJs code:
When you click the Add Records button below, the onSubmit() function that is defined within the VueJS method is called.
The fd variable is used to create a new FormData() object, where the name and author values are specified using the formData.append() procedure.
We may use "this". syntax to retrieve our data variables from within methods. This corresponds to the parent Vue element, which allows us to access our data variables directly.
The Axios sends the data submitted in the form to the “create.php” file through HTTP POST request if all of the fields are fulfilled.
If the response has a success string, the .then() method displays the record insert successfully message, which is stored in the "successMsg" variable. We used this value in the bootstrap alert box.
Otherwise, the .catch() method will display a suitable error message in the console of your browser.
Here, if(response.data.result == ’success ’), the "result" is an array derived from the create.php file. If the record was correctly saved, it will return a success string; otherwise, it will return an error string.
<script>
var application = new Vue({
el: '#bookApp',
data: {
name: '',
author: '',
allRecords: [],
update_id: '',
update_name: '',
update_author: '',
successMsg: ''
},
methods: {
showModal(id) {
this.$refs[id].show()
},
hideModal(id) {
this.$refs[id].hide()
},
onSubmit(){
if(this.name !== '' && this.author !== '') {
var fd = new FormData()
fd.append('name', this.name)
fd.append('author', this.author)
axios({
url: 'create.php',
method: 'post',
data: fd
})
.then(response => {
if(response.data.result == 'success') {
application.successMsg = 'record insert successfully';
this.name = ''
this.author = ''
application.hideModal('add-modal')
application.getRecords()
}else{
alert('error ! record inserting prolem ')
}
})
.catch(error => {
console.log(error)
})
}else{
alert('sorry ! all fields are required')
}
},
getRecords(){
//
},
mounted: function(){
this.getRecords()
}
})
</script>
Next VueJS connect to the database MySQL.
create.php
This file works quietly on the backend and communicates via an Axios HTTP POST request. This file receives the form value request and sends it to the modal class, which correctly inserts the data.
Here we include the model class, which contains all database-related information.
When $model->insert is invoked, all of the data is saved in the table and a success string is returned via the result array variable.
<?php
if (isset($_POST['name']) && isset($_POST['author']))
{
$name = $_POST['name'];
$author = $_POST['author'];
include 'model.php';
$model = new Model();
if ($model->insert($name, $author))
{
$data = array('result' => 'success');
}
else
{
$data = array('result' => 'error');
}
echo json_encode($data);
}
?>
model.php
Model is the name of the PHP Class that we develop. Object-Oriented Programming in PHP is carried out with the help of these PHP Classes. The values of the table for the Database are wrapped in PHP Classes. In the SQL database, we'll define the values that are saved in variable form.
Each object class has a CRUD method that is used to conduct CREATE, READ, UPDATE, and DELETE actions on the provided table rows using PHP functions.
__construct() — Within the try/catch block, this function creates the database connection. If an error occurs, the exception may be simply handled.
insert() — The function creates a database record and returns a true response.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $dbname = 'vuejs_crud_php';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new mysqli($this->host, $this->username, $this->password, $this->dbname);
}
catch (Exception $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert book record into database
public function insert($name, $author)
{
$query = "INSERT INTO tbl_book (book_name, book_author) VALUES ('".$name."', '".$author."')";
if ($sql = $this->connection->query($query))
{
return true;
}
else
{
return;
}
}
//fetch all book record from database
public function fetchAll()
{
}
//delete book record from database
public function delete($id)
{
}
//fetch single book record from database
public function edit($id)
{
}
//update book record
public function update($id, $name, $author)
{
}
}
?>
Demo:
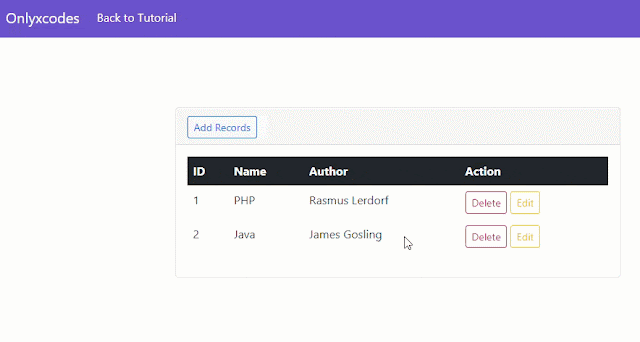
Read Record:
As illustrated in the figure below, the data is shown in a table. So that the data may be shown as I like, I utilize certain vue attributes and binding them to the table components.
We used the v-for directive to repeat all of the "allRecords", as well as some click methods to update and delete book records, which I'll go over in the following part.
<!-- table start-->
<div class="text-muted lead text-center" v-if="!allRecords.length">No record found</div>
<div class="table-responsive" v-if="allRecords.length">
<table class="table table-borderless">
<thead class="table-dark">
<tr>
<th>ID</th>
<th>Name</th>
<th>Author</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr v-for="(record, i) in allRecords" :key="record.book_id">
<td>{{i + 1}}</td>
<td>{{record.book_name}}</td>
<td>{{record.book_author}}</td>
<td>
<button @click="deleteRecord(record.book_id)" class="btn btn-sm btn-outline-danger">Delete</button>
<button @click="editRecord(record.book_id)" class="btn btn-sm btn-outline-warning">Edit</button>
</td>
</tr>
</tbody>
</table>
</div>
</div>
<!-- table end -->
Let's look at how the data in the "allRecords" variable is saved using vue js.
VueJs code:
In Vue, there's a hook called mounted. Whenever a page or a Vue instance launches, this hook is called instantly. So, in this hook, I call a method named "getRecords()", which makes a call to the read.php file, where the data is fetched and returned to view.
<script>
var application = new Vue({
el: '#bookApp',
data: {
name: '',
author: '',
allRecords: [],
update_id: '',
update_name: '',
update_author: '',
successMsg: ''
},
methods: {
showModal(id) {
this.$refs[id].show()
},
hideModal(id) {
this.$refs[id].hide()
},
onSubmit(){
},
getRecords(){
axios({
url: 'read.php',
method: 'get'
})
.then(response => {
this.allRecords = response.data.rows
})
.catch(error => {
console.log(error)
})
},
deleteRecord(id){
},
editRecord(id){
},
onUpdate(){
},
mounted: function(){
this.getRecords()
}
})
</script>
The Axios function sends a request to the read.php file and retrieves all records within the getRecords() method.
The data is put in the variable "allRecords" within the.then() function, therefore iterating(v-for) this variable displays us all the data we need for the view.
We had defined the allRecords array in the data property.
Here, this.allRecords = response.data.rows, the last "rows" is an array derived from the read.php file, which returns all table records in array format.
read.php
Axios HTTP POST request called this file. All table records are sent to the rows array variable if $model->fetchAll is executed.
<?php
include 'model.php';
$model = new Model();
$rows = $model->fetchAll();
$data = array('rows' => $rows);
echo json_encode($data);
?>
model class codes
The code below is pretty simple and clear; the fetchAll() function retrieves all of the data from the database and delivers it, which is then shown on the view using VueJS.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $dbname = 'vuejs_crud_php';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new mysqli($this->host, $this->username, $this->password, $this->dbname);
}
catch (Exception $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert book record into database
public function insert($name, $author)
{
}
//fetch all book record from database
public function fetchAll()
{
$data = [];
$query = "SELECT * FROM tbl_book";
if ($sql = $this->connection->query($query))
{
while ($rows = mysqli_fetch_assoc($sql))
{
$data[] = $rows;
}
}
return $data;
}
//delete book record from database
public function delete($id)
{
}
//fetch single book record from database
public function edit($id)
{
}
//update book record
public function update($id, $name, $author)
{
}
}
?>
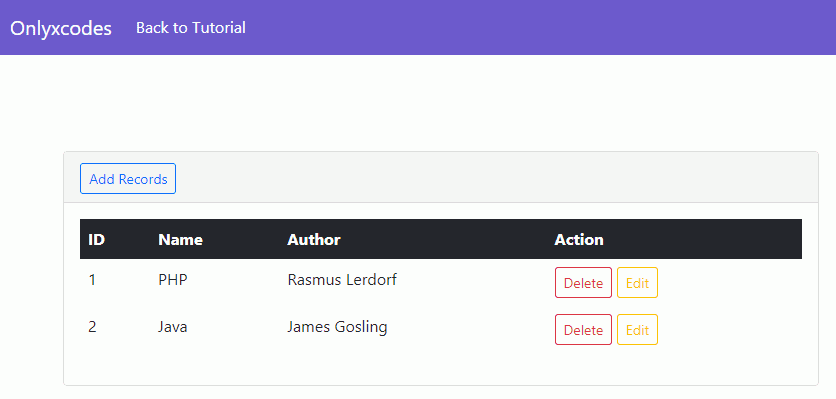
Delete Record
There is a delete icon in the Actions column, which we use to delete stuff.
<button @click="deleteRecord(record.book_id)" class="btn btn-sm btn-outline-danger">Delete</button>
VueJs code:
The deleteRecord(id) method is called when you click the delete icon, and a basic confirmation dialogue appears.
The fd variable is used to create a new FormData() object, where the id value is set using the formData.append() mechanism.
If you select yes, Axios will send the id value request to the “delete.php” file via HTTP POST.
If the response has a success string, the .then() method displays a record deletion success message, which is stored in the "successMsg" variable. We used this value in the bootstrap alert box.
Otherwise, the .catch() function will display a suitable error message in the console of your browser.
Here, if(response.data.result == ’success ’) The result is an array obtained from the delete.php file. If the record was successfully destroyed, it will produce a "success" string; otherwise, it will provide an "error" string.
<script>
var application = new Vue({
el: '#bookApp',
data: {
name: '',
author: '',
allRecords: [],
update_id: '',
update_name: '',
update_author: '',
successMsg: ''
},
methods: {
showModal(id) {
this.$refs[id].show()
},
hideModal(id) {
this.$refs[id].hide()
},
onSubmit(){
},
getRecords(){
},
deleteRecord(id){
if(window.confirm('Are you sure want to delete this record ?')) {
var fd = new FormData()
fd.append('id', id)
axios({
url: 'delete.php',
method: 'post',
data: fd
})
.then(response => {
if(response.data.result == 'success') {
application.successMsg = 'record delete successfully';
application.getRecords();
}else{
alert('sorry ! record not deleting')
}
})
.catch(error => {
console.log(error)
})
}
},
editRecord(id){
},
onUpdate(){
},
mounted: function(){
this.getRecords()
}
})
</script>
delete.php
This file receives a request for a specific id value from the Axios HTTP POST method and sends it to the delete() function in the model class.
The $model->delete($id) function was successfully run, and the result array's success string was the return.
If there is an error, it will return an error string, which will be placed in the result array as well.
<?php
if (isset($_POST['id']))
{
$id = $_POST['id'];
include 'model.php';
$model = new Model();
if ($model->delete($id))
{
$data = array('result' => 'success');
}
else
{
$data = array('result' => 'error');
}
echo json_encode($data);
}
?>
model class codes
The delete() function returns true when it deletes a single record from the database table.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $dbname = 'vuejs_crud_php';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new mysqli($this->host, $this->username, $this->password, $this->dbname);
}
catch (Exception $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert book record into database
public function insert($name, $author)
{
}
//fetch all book record from database
public function fetchAll()
{
}
//delete book record from database
public function delete($id)
{
$query = "DELETE FROM tbl_book WHERE book_id = '".$id."' ";
if ($sql = $this->connection->query($query))
{
return true;
}
else
{
return;
}
}
//fetch single book record from database
public function edit($id)
{
}
//update book record
public function update($id, $name, $author)
{
}
}
?>
Demo:
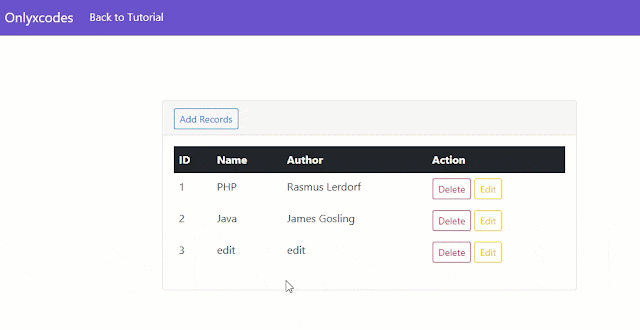
Update Records
Under the "Actions" column, a table containing all of the data is displayed, with two icons "Edit" and "Delete."
When the edit icon is hit, a modal appears displaying the data for that particular row and the choice to modify it.
<button @click="editRecord(record.book_id)" class="btn btn-sm btn-outline-warning">Edit</button>
VueJS code:
The editRecord(id) method is triggered when you click the edit icon, and it returns a specific record id.
The fd variable is used to create a new FormData() object, where the id value is set using the formData.append() procedure.
The Axios uses an HTTP POST request to send the id value request to the “edit.php” file. If the response has a success string, then it is included within. The records of particular table rows are displayed in the bootstrap update modal using the then() function.
Here, if(response.data.result == ’success ’) the result is an array that come from "edit.php" file. The row is an array that comes from the edit.php code, which displays table records as an array.
And response.data.row[0], response.data.row[1], and response.data.row[2] display table-specific row records that need to be updated.
We assign table records to data property variables update id, update name, and update author using "this" syntax.
<script>
var application = new Vue({
el: '#bookApp',
data: {
name: '',
author: '',
allRecords: [],
update_id: '',
update_name: '',
update_author: '',
successMsg: ''
},
methods: {
showModal(id) {
this.$refs[id].show()
},
hideModal(id) {
this.$refs[id].hide()
},
onSubmit(){
},
getRecords(){
},
deleteRecord(id){
},
editRecord(id){
var fd = new FormData()
fd.append('id', id)
axios({
url: 'edit.php',
method: 'post',
data: fd
})
.then(response => {
if(response.data.result == 'success') {
this.update_id = response.data.row[0]
this.update_name = response.data.row[1]
this.update_author = response.data.row[2]
application.showModal('update-modal')
}
})
.catch(error => {
console.log(error)
})
},
onUpdate(){
},
mounted: function(){
this.getRecords()
}
})
</script>
edit.php
This file receives a request for a specific id value through the Axios POST method and sends it to the edit() function, which is then called in the model class.
After $model->edit($id) is successfully executed, we will receive a row array containing table records.
Return a result array that includes a key string for success and an error key string.
<?php
if (isset($_POST['id']))
{
$id = $_POST['id'];
include 'model.php';
$model = new Model();
if ($row = $model->edit($id))
{
$data = array('result' => 'success', 'row' => $row);
}
else
{
$data = array('result' => 'error');
}
echo json_encode($data);
}
?>
model class codes
The edit($id) function returns data after selecting and fetching table row data by id.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $dbname = 'vuejs_crud_php';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new mysqli($this->host, $this->username, $this->password, $this->dbname);
}
catch (Exception $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert book record into database
public function insert($name, $author)
{
}
//fetch all book record from database
public function fetchAll()
{
}
//delete book record from database
public function delete($id)
{
}
//fetch single book record from database
public function edit($id)
{
$data = [];
$query = "SELECT * FROM tbl_book WHERE book_id = '".$id."' ";
if ($sql = $this->connection->query($query))
{
$row = mysqli_fetch_row($sql);
$data = $row;
}
return $data;
}
//update book record
public function update($id, $name, $author)
{
}
}
?>
Now we updating Records,
We apply the same approach as in the create record scripts.
See @submit.prevent="onUpdate" in the form tag. It implies that instead of submitting the form normally, we run onUpdate. Within the Vue method, we construct the onUpdate() method that sends an Axios request to PHP to update the record and then redirects to the table.
The update_name and update_author bootstrap update modal form parameters are bindings. We'll bind update modal form input fields with the v-model directive.
<!-- Update Book Record Modal Start -->
<div>
<b-modal ref="update-modal" hide-footer title="Update Book Record">
<div>
<form action="" @submit.prevent="onUpdate">
<div class="form-group">
<label for="">Book Name</label>
<input type="text" v-model="update_name" class="form-control">
</div>
<br />
<div class="form-group">
<label for="">Author</label>
<input type="text" v-model="update_author" class="form-control">
</div>
<br />
<div class="form-group">
<button class="btn btn-sm btn-outline-primary">Update Record</button>
<b-button class="btn btn-sm btn-outline-danger" variant="outline-danger" block @click="hideModal('update-modal')">Close
</b-button>
</div>
</form>
</div>
</b-modal>
</div>
<!-- Update Book Record Modal End -->
VueJs code:
The onUpdate() method is activated when you click the Update Records button below.
The fd variable is used to create a new FormData() item, where the id, name, and author values are specified using the formData.append() function.
The Axios sends the data input in the form to the "update.php" file through HTTP POST request if all of the fields have been changed.
If the response has a success string, the .then() function displays the record update successfully message, which is stored in the "successMsg" variable. We used this value in the bootstrap alert box.
Here, if(response.data.result == ’success ’) The output is an array derived from the update.php file. If the record was correctly updated, it will return a success string; otherwise, it will return an error string.
<script>
var application = new Vue({
el: '#bookApp',
data: {
name: '',
author: '',
allRecords: [],
update_id: '',
update_name: '',
update_author: '',
successMsg: ''
},
methods: {
showModal(id) {
this.$refs[id].show()
},
hideModal(id) {
this.$refs[id].hide()
},
onSubmit(){
},
getRecords(){
},
deleteRecord(id){
},
editRecord(id){
},
onUpdate(){
if(this.update_name !== '' && this.update_author !== '' && this.update_id !== '') {
var fd = new FormData()
fd.append('id', this.update_id)
fd.append('name', this.update_name)
fd.append('author', this.update_author)
axios({
url: 'update.php',
method: 'post',
data: fd
})
.then(response => {
if(response.data.result == 'success') {
application.successMsg = 'record update successfully' ;
this.update_name = '';
this.update_author = '';
this.update_id = '';
application.hideModal('update-modal');
application.getRecords();
}
})
.catch(error => {
console.log(error)
})
}else{
alert('sorry ! all fields are required')
}
}
},
mounted: function(){
this.getRecords()
}
})
</script>
update.php
This file works silently on the backend and communicates via an Axios HTTP POST request. This file receives the update modal form values request and sends it to the modal class, which properly updates the data.
If $model->update is done, all of the data in the table will be updated.
<?php
if (isset($_POST['id']) && isset($_POST['name']) && isset($_POST['author']))
{
$id = $_POST['id'];
$name = $_POST['name'];
$author = $_POST['author'];
include 'model.php';
$model = new Model();
if ($model->update($id, $name, $author))
{
$data = array('result' => 'success');
}
else
{
$data = array('result' => 'error');
}
echo json_encode($data);
}
?>
model class code
The update() function changed the row record in the table and returned a true response that's it.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $dbname = 'vuejs_crud_php';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new mysqli($this->host, $this->username, $this->password, $this->dbname);
}
catch (Exception $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert book record into database
public function insert($name, $author)
{
}
//fetch all book record from database
public function fetchAll()
{
}
//delete book record from database
public function delete($id)
{
}
//fetch single book record from database
public function edit($id)
{
}
//update book record
public function update($id, $name, $author)
{
$query = "UPDATE tbl_book SET book_name = '".$name."', book_author = '".$author."' WHERE book_id = '".$id."' ";
if ($sql = $this->connection->query($query))
{
return true;
}
else
{
return;
}
}
}
?>
Demo:
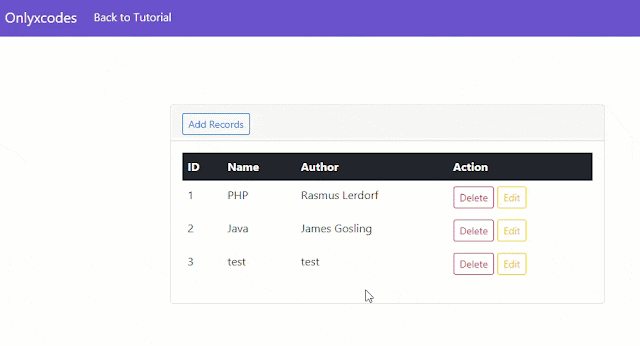
index.html
I've included the whole index file codes here, along with row numbers, so you can find them quickly.
Row no 9 to 15 – Included bootstrap and VueJS CSS files.
Row no 37 – In this division tag, all HTML content is loaded into an element with the id "bookApp." <div class="container" id="bookApp">
Row no 41 to 46 – Available bootstrap alert box that displays VueJS CRUD operations all messages.
Row no 48 to 76 – I placed the bootstrap add modal within the available add records button. The bootstrap adds modal appears when you click the add record button.
Row no 80 to 106 – A table is available that displays all database records in a tabular layout.
Row no 110 to 134 – Here bootstrap update modal available that appear if you click on the edit icon.
Row no 140 to 150 – The JavaScript libraries VueJS, Axios, and Bootstrap are all included.
Row no 152 to 309 – I put all of the VueJS CRUD operations codes here.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link type="text/css" rel="stylesheet" href="//unpkg.com/bootstrap/dist/css/bootstrap.min.css" />
<!-- BootstrapVue CSS -->
<link type="text/css" rel="stylesheet" href="//unpkg.com/bootstrap-vue@latest/dist/bootstrap-vue.min.css" />
<!-- Bootstrap npm CSS -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-+0n0xVW2eSR5OomGNYDnhzAbDsOXxcvSN1TPprVMTNDbiYZCxYbOOl7+AMvyTG2x" crossorigin="anonymous">
<title>VUEJS CRUD With PHP With Source Code</title>
</head>
<body class="bg-white">
<nav class="navbar navbar-expand-lg navbar-dark" style="background-color: #6a5acd;">
<div class="container-fluid">
<a class="navbar-brand" href="https://www.onlyxcodes.com/">Onlyxcodes</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav me-auto mb-2 mb-lg-0">
<li class="nav-item">
<a class="nav-link active" aria-current="page" href="https://www.onlyxcodes.com/2021/06/vuejs-crud-with-php-mysql.html">Back to Tutorial</a>
</li>
</ul>
</div>
</div>
</nav>
<div class="container" id="bookApp">
<div class="row">
<div class="col-md-8 mx-auto my-5">
<!-- Below Bootstrap Alert dialog show record Insert and Update Messages -->
<div v-if="successMsg">
<b-alert show variant="success">
{{ successMsg }}
</b-alert>
</div>
<div class="card mt-5">
<div class="card-header">
<div class="d-flex d-flex justify-content-between">
<div class="lead"><button id="show-btn" @click="showModal('add-modal')" class="btn btn-sm btn-outline-primary">Add Records</button></div>
</div>
</div>
<div class="card-body">
<!-- Add Book Record Modal Start-->
<b-modal ref="add-modal" hide-footer title="Add Book Records">
<form action="" @submit.prevent="onSubmit">
<div class="form-group">
<label for="">Book Name</label>
<input type="text" v-model="name" class="form-control">
</div>
<br />
<div class="form-group">
<label for="">Author</label>
<input type="text" v-model="author" class="form-control">
</div>
<br />
<div class="form-group">
<button class="btn btn-sm btn-outline-success">Add Records</button>
<b-button class="btn btn-sm btn-outline-danger" variant="outline-danger" block @click="hideModal('add-modal')">Close
</b-button>
</div>
</form>
</b-modal>
<!-- Add Book Record Modal End -->
<!-- table start-->
<div class="text-muted lead text-center" v-if="!allRecords.length">No record found</div>
<div class="table-responsive" v-if="allRecords.length">
<table class="table table-borderless">
<thead class="table-dark">
<tr>
<th>ID</th>
<th>Name</th>
<th>Author</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr v-for="(record, i) in allRecords" :key="record.book_id">
<td>{{i + 1}}</td>
<td>{{record.book_name}}</td>
<td>{{record.book_author}}</td>
<td>
<button @click="deleteRecord(record.book_id)" class="btn btn-sm btn-outline-danger">Delete</button>
<button @click="editRecord(record.book_id)" class="btn btn-sm btn-outline-warning">Edit</button>
</td>
</tr>
</tbody>
</table>
<!-- table end -->
</div>
</div>
</div>
<!-- Update Book Record Modal Start -->
<div>
<b-modal ref="update-modal" hide-footer title="Update Book Record">
<div>
<form action="" @submit.prevent="onUpdate">
<div class="form-group">
<label for="">Book Name</label>
<input type="text" v-model="update_name" class="form-control">
</div>
<br />
<div class="form-group">
<label for="">Author</label>
<input type="text" v-model="update_author" class="form-control">
</div>
<br />
<div class="form-group">
<button class="btn btn-sm btn-outline-primary">Update Record</button>
<b-button class="btn btn-sm btn-outline-danger" variant="outline-danger" block @click="hideModal('update-modal')">Close
</b-button>
</div>
</form>
</div>
</b-modal>
</div>
<!-- Update Book Record Modal End -->
</div>
</div>
</div>
<!-- Vuejs -->
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<!-- BootstrapVue js -->
<script type="text/javascript" src="//unpkg.com/bootstrap-vue@latest/dist/bootstrap-vue.min.js"></script>
<!-- Axios -->
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<!-- Bootstrap js -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js" integrity="sha384-gtEjrD/SeCtmISkJkNUaaKMoLD0//ElJ19smozuHV6z3Iehds+3Ulb9Bn9Plx0x4" crossorigin="anonymous"></script>
<script>
var application = new Vue({
el: '#bookApp',
data: {
name: '',
author: '',
allRecords: [],
update_id: '',
update_name: '',
update_author: '',
successMsg: ''
},
methods: {
showModal(id) {
this.$refs[id].show()
},
hideModal(id) {
this.$refs[id].hide()
},
onSubmit(){
if (this.name !== '' && this.author !== '') {
var fd = new FormData()
fd.append('name', this.name)
fd.append('author', this.author)
axios({
url: 'create.php',
method: 'post',
data: fd
})
.then(response => {
if (response.data.result == 'success') {
application.successMsg = 'record insert successfully';
this.name = ''
this.author = ''
application.hideModal('add-modal')
application.getRecords()
}else{
alert('error ! record inserting prolem ')
}
})
.catch(error => {
console.log(error)
})
}else{
alert('sorry ! all fields are required')
}
},
getRecords(){
axios({
url: 'read.php',
method: 'get'
})
.then(response => {
this.allRecords = response.data.rows
})
.catch(error => {
console.log(error)
})
},
deleteRecord(id){
if (window.confirm('Are you sure want to delete this record ?')) {
var fd = new FormData()
fd.append('id', id)
axios({
url: 'delete.php',
method: 'post',
data: fd
})
.then(response => {
if (response.data.result == 'success') {
application.successMsg = 'record delete successfully';
application.getRecords();
}else{
alert('sorry ! record not deleting')
}
})
.catch(error => {
console.log(error)
})
}
},
editRecord(id){
var fd = new FormData()
fd.append('id', id)
axios({
url: 'edit.php',
method: 'post',
data: fd
})
.then(response => {
if (response.data.result == 'success') {
this.update_id = response.data.row[0]
this.update_name = response.data.row[1]
this.update_author = response.data.row[2]
application.showModal('update-modal')
}
})
.catch(error => {
console.log(error)
})
},
onUpdate(){
if (this.update_name !== '' && this.update_author !== '' && this.update_id !== '') {
var fd = new FormData()
fd.append('id', this.update_id)
fd.append('name', this.update_name)
fd.append('author', this.update_author)
axios({
url: 'update.php',
method: 'post',
data: fd
})
.then(response => {
if (response.data.result == 'success') {
application.successMsg = 'record update successfully' ;
this.update_name = '';
this.update_author = '';
this.update_id = '';
application.hideModal('update-modal');
application.getRecords();
}
})
.catch(error => {
console.log(error)
})
}else{
alert('sorry ! all fields are required')
}
}
},
mounted: function(){
this.getRecords()
}
})
</script>
</body>
</html>
model.php
Each function executes CRUD actions on the book table, while the model class codes underneath contain MySQL database connection details.
<?php
Class Model
{
private $host = 'localhost';
private $username = 'root';
private $password = '';
private $dbname = 'vuejs_crud_php';
private $connection;
//create connection
public function __construct()
{
try
{
$this->connection = new mysqli($this->host, $this->username, $this->password, $this->dbname);
}
catch (Exception $e)
{
echo "Connection error " . $e->getMessage();
}
}
// insert book record into database
public function insert($name, $author)
{
$query = "INSERT INTO tbl_book (book_name, book_author) VALUES ('".$name."', '".$author."')";
if ($sql = $this->connection->query($query))
{
return true;
}
else
{
return;
}
}
//fetch all book record from database
public function fetchAll()
{
$data = [];
$query = "SELECT * FROM tbl_book";
if ($sql = $this->connection->query($query))
{
while ($rows = mysqli_fetch_assoc($sql))
{
$data[] = $rows;
}
}
return $data;
}
//delete book record from database
public function delete($id)
{
$query = "DELETE FROM tbl_book WHERE book_id = '".$id."' ";
if ($sql = $this->connection->query($query))
{
return true;
}
else
{
return;
}
}
//fetch single book record from database
public function edit($id)
{
$data = [];
$query = "SELECT * FROM tbl_book WHERE book_id = '".$id."' ";
if ($sql = $this->connection->query($query))
{
$row = mysqli_fetch_row($sql);
$data = $row;
}
return $data;
}
//update book record
public function update($id, $name, $author)
{
$query = "UPDATE tbl_book SET book_name = '".$name."', book_author = '".$author."' WHERE book_id = '".$id."' ";
if ($sql = $this->connection->query($query))
{
return true;
}
else
{
return;
}
}
}
?>
Download Codes
what version of php?
ReplyDeleteHi. Could you give me access to the source code, please? I need it badly. Thanks!
ReplyDeleteLike the Facebook page and put your email here after I enable source code link in your email.
Delete[email protected]
ReplyDeleteSorry your email [email protected] unverified
Deleteoh... I used [email protected].. could you check it again and give me the permission to download the source code afterwards. thanks.
Delete[email protected]
ReplyDeleteSorry your email [email protected] unverified
Deletesorry this email not found
Delete[email protected]
ReplyDeleteSorry your email not verify please verify email and like Facebook page. After I enable source code link in your email
DeleteHi i liked your fb as Piotr Zawadzki but I don't have access to my fb email. Can you send the invitation to [email protected] instead?
ReplyDeletesubscribe manually I will check and enable source code link in your email
DeleteHi, I followed using [email protected] yet I didn't receive any email and after clicking the link below the article I still get the message that the code isn't available for me. Did I do something wrong?
DeleteOK, I enable source code link in your email check again and download it. Keep Visiting
DeleteHey there, good example. I liked your FB page. Would it be possible to get the source code please? Andy
ReplyDeletealso subscribe required
Delete[email protected] or [email protected]
ReplyDeletesubscribe also required including with fb like page
DeleteSubscribed as [email protected]
DeleteOK, I enable source code link in your email check again and download it. Keep Visiting
Delete[email protected]
ReplyDeleteHello Sir, Can I access your code please?
ReplyDelete[email protected]
ReplyDeleteOk I enable source code link in your email check again and download it
DeleteMorning sir, my e-mail for access is [email protected]
ReplyDeleteThank you.
Ok I enable source code link in your email check again and download it.
DeleteKeep visiting
Please share source code to [email protected]
ReplyDeleteHi, Only Subscriber and Facebook Page Like Users Can Download Source Code
DeleteHello, sorry, is possible to have source code please.
ReplyDeleteI already suscribe and like your page. Thanks you
odilonserandrian (at) gmail.com
I enabled source code link in your email check again and download it
Deletehello, thanks for sharing on this email
ReplyDeletesubscribed also, odilonserandrian (at) gmail.com